Help me in C# please, this is what I have done so far. I'm stuck at if user input just Enter key, or 12:20 (Input is not the right format, or "Blahhaha" (string letter) will print out "Enter valid input" as instructions. And when the user input the wrong format for the first time, it has to catch an error and stop the program immediately. class Program { public static int militaryTime (string s1, string s2) { string[] arr1 = s1.Split(":", 3); string[] arr2 = s2.Split(":", 3); if (Convert.ToInt32(arr1[0]) > 23 || Convert.ToInt32(arr1[0]) < 0 || Convert.ToInt32(arr2[0]) > 23 || Convert.ToInt32(arr2[0]) < 0) throw new InvalidTimeException("Hour must be below 24"); if (Convert.ToInt32(arr1[1]) > 59 || Convert.ToInt32(arr1[0]) < 0 || Convert.ToInt32(arr2[1]) > 59 || Convert.ToInt32(arr2[1]) < 0) throw new InvalidTimeException("Minutes must be less than 60"); if (Convert.ToInt32(arr1[2]) > 23 || Convert.ToInt32(arr1[0]) < 0 || Convert.ToInt32(arr2[2]) > 59 || Convert.ToInt32(arr2[2]) < 0) throw new InvalidTimeException("Seconds must be less than 60"); if (Convert.ToBoolean (arr1.Length != 3)) throw new InvalidTimeException("Seconds must be less than 60"); //if (arr1.Length != 3 || arr2.Length != 3) //{ // throw new InvalidTimeException("Enter a valid time"); //} else { int hour = Convert.ToInt32(arr1[0]) - Convert.ToInt32(arr2[0]); int min = Convert.ToInt32(arr1[1]) - Convert.ToInt32(arr2[1]); int sec = Convert.ToInt32(arr1[2]) - Convert.ToInt32(arr2[2]); int result = (hour * 3600) + (min * 60) + sec; if (result < 0) { result *= -1; } return result; } } static void Main(string[] args) { Console.WriteLine("Enter time 1 in 24hr format as follows (HH:MM:SS) "); string input1 = Console.ReadLine(); Console.WriteLine("Enter time 2 in 24hr format as follows (HH:MM:SS) "); string input2 = Console.ReadLine(); try { Console.WriteLine("Difference in seconds: " + militaryTime(input1, input2)); } catch (InvalidTimeException e) {
Help me in C# please, this is what I have done so far. I'm stuck at if user input just Enter key, or 12:20 (Input is not the right format, or "Blahhaha" (string letter) will print out "Enter valid input" as instructions. And when the user input the wrong format for the first time, it has to catch an error and stop the program immediately.
class Program
{
public static int militaryTime (string s1, string s2)
{
string[] arr1 = s1.Split(":", 3);
string[] arr2 = s2.Split(":", 3);
if (Convert.ToInt32(arr1[0]) > 23 || Convert.ToInt32(arr1[0]) < 0 || Convert.ToInt32(arr2[0]) > 23 || Convert.ToInt32(arr2[0]) < 0)
throw new InvalidTimeException("Hour must be below 24");
if (Convert.ToInt32(arr1[1]) > 59 || Convert.ToInt32(arr1[0]) < 0 || Convert.ToInt32(arr2[1]) > 59 || Convert.ToInt32(arr2[1]) < 0)
throw new InvalidTimeException("Minutes must be less than 60");
if (Convert.ToInt32(arr1[2]) > 23 || Convert.ToInt32(arr1[0]) < 0 || Convert.ToInt32(arr2[2]) > 59 || Convert.ToInt32(arr2[2]) < 0)
throw new InvalidTimeException("Seconds must be less than 60");
if (Convert.ToBoolean (arr1.Length != 3))
throw new InvalidTimeException("Seconds must be less than 60");
//if (arr1.Length != 3 || arr2.Length != 3)
//{
// throw new InvalidTimeException("Enter a valid time");
//}
else
{
int hour = Convert.ToInt32(arr1[0]) - Convert.ToInt32(arr2[0]);
int min = Convert.ToInt32(arr1[1]) - Convert.ToInt32(arr2[1]);
int sec = Convert.ToInt32(arr1[2]) - Convert.ToInt32(arr2[2]);
int result = (hour * 3600) + (min * 60) + sec;
if (result < 0)
{
result *= -1;
}
return result;
}
}
static void Main(string[] args)
{
Console.WriteLine("Enter time 1 in 24hr format as follows (HH:MM:SS) ");
string input1 = Console.ReadLine();
Console.WriteLine("Enter time 2 in 24hr format as follows (HH:MM:SS) ");
string input2 = Console.ReadLine();
try
{
Console.WriteLine("Difference in seconds: " + militaryTime(input1, input2));
}
catch (InvalidTimeException e)
{
Console.WriteLine(e.Message);
}
}
public class InvalidTimeException : Exception
{
public InvalidTimeException() { }
public InvalidTimeException(string message) : base(message) { }
public InvalidTimeException(string message, Exception inner) : base(message, inner) { }
}
}



Trending now
This is a popular solution!
Step by step
Solved in 2 steps

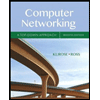
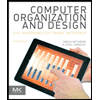
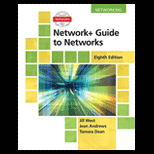
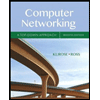
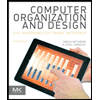
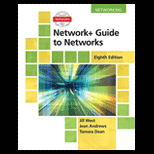
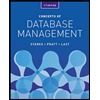
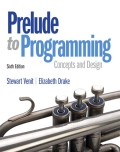
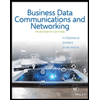