he Ministry of Vybsie_Culcha intends to implement a grant for singers in order to offset difficulties experienced in the pandemic. The grant is to be funded from a fixed pool of funds (so the first set of singers that exhaust the funds are the only ones that are able to receive the grant). You are contracted to write a program that models the expected payouts. In order to reduce the possibility of fraud however, the ministry has decided to implement a (controversial) strategy that requires entertainers to prove they are the song owners by recording it at a Studio, even if already released. The Ministry maintains a list of Studios, and code for the Ministry and Studio classes have already been fully implemented. The starting code also includes calls to a template of a Singer class that interacts with a Song class. Your task is to execute eight(8) tasks that complete the Singer and Song classes. MARKING CRITERIA (PLEASE REVIEW BEFORE STARTING) Criterion Mark(s) Accessors and mutators for Singer and Song 1 Explanation of the importance of the toString method 1 Correct implementation of logic to sum items in the song ArrayList 1 Explanation of your logic for tryToRegisterSong() 2 NOTE ::: No part of Ministry or Studio should be modified. Note that where convenient, and allowed, you may declare private (support) methods.
The Ministry of Vybsie_Culcha intends to implement a grant for singers in order to offset difficulties experienced in the pandemic. The grant is to be funded from a fixed pool of funds (so the first set of singers that exhaust the funds are the only ones that are able to receive the grant). You are contracted to write a program that models the expected payouts. In order to reduce the possibility of fraud however, the ministry has decided to implement a (controversial) strategy that requires entertainers to prove they are the song owners by recording it at a Studio, even if already released. The Ministry maintains a list of Studios, and code for the Ministry and Studio classes have already been fully implemented. The starting code also includes calls to a template of a Singer class that interacts with a Song class. Your task is to execute eight(8) tasks that complete the Singer and Song classes.
MARKING CRITERIA (PLEASE REVIEW BEFORE STARTING) Criterion Mark(s) Accessors and mutators for Singer and Song 1 Explanation of the importance of the toString method 1 Correct implementation of logic to sum items in the song ArrayList 1 Explanation of your logic for tryToRegisterSong() 2
NOTE ::: No part of Ministry or Studio should be modified. Note that where convenient, and allowed, you may declare private (support) methods.
LAB EXERCISES
Figure 1:Simplified UML Class Diagram for the exercise Figure 1 depicts the classes to which you will interface while completing the exercise.
- In class Singer,: a. Complete the first constructor that accepts name, genre, budget, as well as a reference to the Ministry(incoming arguments include n for the name, g for genre, and b for budget).
b. Implement the second constructor so that all instance variables are initialized. - In class Song, write the calculation in method getEstEarnings of class Song to evaluate estimated earnings. Note that while in the real world, other methods would be used to estimate earnings, in this simplified model, the estimated earnings will be the length of the title, multiplied by the a value extracted by the ministry using the method getSongPartEst(hint.. the singer keeps a reference to the ministry. You can observe how the reference is used in method getClaimableEarnings).
- In class Singer, correct the method sumEstEarnings to evaluate the sum of estimates earnings for the singer.
- Record a song in a studio and try to register the song a. In class Song write a mutator named setStudio(Studio studio) -method for the song that accepts a Studio, and sets the associated studio on the on the song to the referenced Studio. b. In class Singer, method tryToRegisterSong, if the singer has a preferred studio, set studio to the preferred studio (use method setStudio()), then add the song to the list of registered songs.
- In class Singer, method tryToRegisterSong, update logic to check if artist can afford studio before setting the studio on the song.
- In class Singer, method tryToRegisterSong, update logic to allow ministry to suggest a studio by calling the method getBestAvailableStudio(int budget, Studio preferred) from Ministry, which returns a suggested studio. If the method returns a studio (returned value is not null) then set the studio for the song to the returned studio if the singer is able to afford it. You can then check if the song has been connected with a studio, and if it is, add the song to the list of registered songs.
- In class Singer, method tryToRegisterSong , ensure singer's budget is reduced by the cost of the studio.
- In class Singer, method tryToRegisterSong , call the Studio's reserve method when adding a song to the list of registered songs. Also, check to see if a studio is available (using the isAvailable() method in the studio class) before assigning the song to the studio.
Input Format
Template code handles inputs
Constraints
t<=10
Output Format
as defined

![64
65 }
66
67
68 class Singer {
69
70
71
72
73
74
74
75
76
76
77
77
79
78
79 ▾
80
81
82
83
84
85
86
87
::25 =ö×ÉL:SŠESSÉNER?
88
89
90
91 v
92
93 ▼
94
95
96 ▼
97
98
99
100
100
101
101
102
103
104 v
105
106
107
108
100
109 ▼
110
111
113
112
113
ve
114
115
116
117
118
119
119
120
120
121
121
122
122
123
124
121.
125
126
137
127
179
128
120
129
120
130
131
132
133
134
135
138
139
140
141
142
143
142
^^^
144
145
145
146
146
147
148
149
150
161
151
152
152
153 v
199
}
154
K
155
Ar
156
157
157
158
159
160
161
162
private String name="", genre;
private int budget;
private Studio favStudio;
private ArrayList<Song> songs, registeredSongs;
private Ministry ministry;
private int grantValue;
private String grantMessage;
private boolean willApply;
public
}
}
public
Singer (String name, String genre, int budget, int fav, Ministry ministry,
boolean willApply ) {
this. favStudio= ministry.getStudio (fav);
/*Q1b. Add code to complete this constructor*/
}
public Ministry getMinistry() {
return ministry;
}
public
Singer (String n, String g, int b, Ministry min, boolean want ToApply ) {
//question la
ministry= min;
}
public boolean studioExists (Studio favStudio)
{
return (favStudio!=null);
}
songs new ArrayList< Song> ();
registeredSongs= new ArrayList< Song>();
/*Qla. Add code to complete this constructor*/
grantMessage = "";
this. favStudio=null;
willApply wantToApply;
public boolean canAfford (Studio studio)
{
return studio.getCost() <= budget;
}
136 - public void tryToRegister Song (Song song) {
137
boolean applying () {
return willApply;
public int getGrantValue() {
return grantValue;
public int sumRegistered Songs () {
int sum= 0;
}
}
for (Song regsong: registeredSongs)
}
public int sumEstValue() {
int sum= 0;
/*Code to implement Q3 here */
return sum;
public String getName() {
return name;
}
sum+=regsong.getClaimable Earnings ();
return sum;
String.format("%,d", budget) + ".";
if (favStudio==null)
String str "Registering "+song.getTitle()+" with budget $"+
}
str = str + "No preferred studio.";
else
{
str = str + "Prefers "+favStudio.getName();
if (!favStudio.isAvailable())
str+=" (Not available).";
else
str+="(Available:cost [$"+String.format("%,d", favStudio.getCost())+"]).";
System.out.println(str);
//////////IN THIS METHOD, DO NOT MODIFY ABOVE THIS LINE //////////////
/*Code for logic that can pass test cases 5, 6 and 7 can go here* /
public void addSong (String title) {
songs.add(new Song (title, genre, this ));](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F2ca3eaf2-3bda-40e1-af2e-245059bd57cf%2Fc4ac0290-f65c-4e63-bdc0-e73861a1a386%2Fs1k0t0v_processed.jpeg&w=3840&q=75)

Step by step
Solved in 2 steps

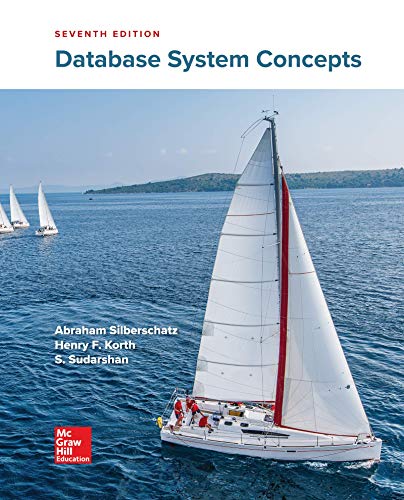
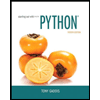
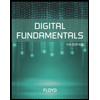
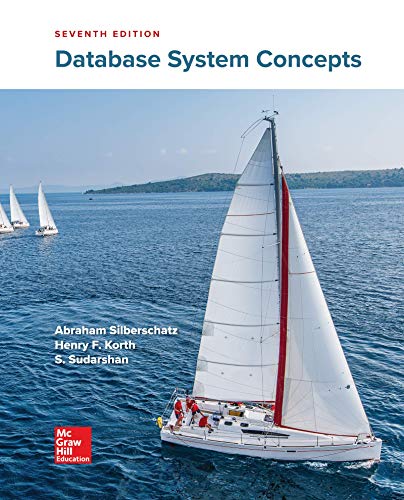
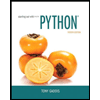
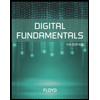
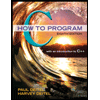
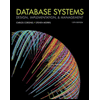
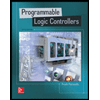