Given three integers, i, j, and k, a sequence sum to be the value of i + (i + 1) + (i + 2) + (i + 3) + ... + j + (j − 1) + (j − 2) + (j − 3) + ... + k (increment from /until it equals j, then decrement from juntil it equals k). Given values i, j, and k, calculate the sequence sum as described. Example i=5 j=9 k=6 Sum all the values from ito jand back to k: 5+ 6+ 7+8+9+8+ 7 + 6 = 56. Function Description Complete the function getSequenceSum in the editor below. getSequenceSum has the following parameter(s): int i, int j, int k: three integers Return long: the value of the sequence sum Constraints ● -108 si, j, ks 108 • i, ksj ▼ Input Format For Custom Testing The first line contains an integer, i. The next line contains an integer, j. The last line contains an integer, k. Sample Case 0 Sample Input 0 STDIN Function 0 5 -1 → i = 0 ➜ j=5 k = -1 Sample Output 0 24
Given three integers, i, j, and k, a sequence sum to be the value of i + (i + 1) + (i + 2) + (i + 3) + ... + j + (j − 1) + (j − 2) + (j − 3) + ... + k (increment from /until it equals j, then decrement from juntil it equals k). Given values i, j, and k, calculate the sequence sum as described. Example i=5 j=9 k=6 Sum all the values from ito jand back to k: 5+ 6+ 7+8+9+8+ 7 + 6 = 56. Function Description Complete the function getSequenceSum in the editor below. getSequenceSum has the following parameter(s): int i, int j, int k: three integers Return long: the value of the sequence sum Constraints ● -108 si, j, ks 108 • i, ksj ▼ Input Format For Custom Testing The first line contains an integer, i. The next line contains an integer, j. The last line contains an integer, k. Sample Case 0 Sample Input 0 STDIN Function 0 5 -1 → i = 0 ➜ j=5 k = -1 Sample Output 0 24
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question

Transcribed Image Text:Given three integers, i, j, and k, a sequence sum to be the value of i+ (i + 1) + (i + 2) + (i + 3) + ... + j + (j − 1)
+ (j − 2) + (j − 3) + ... + k (increment from /until it equals j, then decrement from juntil it equals k). Given
values i, j, and k, calculate the sequence sum as described.
Example
i=5
j=9
k = 6
Sum all the values from ito jand back to k: 5+ 6+ 7+ 8+9+8+ 7 + 6 = 56.
Function Description
Complete the function getSequenceSum in the editor below.
getSequenceSum has the following parameter(s):
int i, int j, int k: three integers
Return
long: the value of the sequence sum
Constraints
-108 si, j, ks 108
• i, ksj
▼ Input Format For Custom Testing
The first line contains an integer, i.
The next line contains an integer, j.
The last line contains an integer, k.
▾ Sample Case 0
Sample Input 0
STDIN
0
5
-1
➜
24
→
Function
i=0
j = 5
k = -1
Sample Output 0
![▼ Input Format For Custom Testing
The first line contains an integer, i.
The next line contains an integer, j.
The last line contains an integer, k.
▾ Sample Case 0
Sample Input 0
STDIN
5
-1
15
16
17
18
19
20
21
22
23
24
25
26
27
28
24
Sample Output 0
Explanation 0
i=0
j=5
k = -1
0 +1+2+3+4+5+4+3+2+1+0+ -1 = 24
STDIN
▾ Sample Case 1
Sample Input 1
-5
Function
-3
----
i = 0
j=5
k = -1
-20
→
Sample Output 1
Explanation 1
Function
i = -5
j = -1
k = -3
i=-5
j=-1
k=-3
-5 +4+3+-2 +-1 + 2 + 3 = -20
13
14 if
1 ✓ #!/bin/python3
2
3
4
5
6
7
8
9
10
11
12
import math
import os
import random
import re
import sys
# Complete the getSequenceSum function below.
def getSequenceSum(i, j, k):
== '__main__':
name
fptr = open(os.environ ['OUTPUT_PATH'], 'w')
i = int(input().strip())
j = int(input().strip())
k = int(input().strip())
res = getSequenceSum (i, j, k)
fptr.write(str(res) + '\n')
fptr.close()](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F2cd2d665-daee-46b8-ac4c-2b4cec66538a%2F89fe89f5-8b48-462f-81d0-2a4b90bc6f52%2F0j05nos_processed.png&w=3840&q=75)
Transcribed Image Text:▼ Input Format For Custom Testing
The first line contains an integer, i.
The next line contains an integer, j.
The last line contains an integer, k.
▾ Sample Case 0
Sample Input 0
STDIN
5
-1
15
16
17
18
19
20
21
22
23
24
25
26
27
28
24
Sample Output 0
Explanation 0
i=0
j=5
k = -1
0 +1+2+3+4+5+4+3+2+1+0+ -1 = 24
STDIN
▾ Sample Case 1
Sample Input 1
-5
Function
-3
----
i = 0
j=5
k = -1
-20
→
Sample Output 1
Explanation 1
Function
i = -5
j = -1
k = -3
i=-5
j=-1
k=-3
-5 +4+3+-2 +-1 + 2 + 3 = -20
13
14 if
1 ✓ #!/bin/python3
2
3
4
5
6
7
8
9
10
11
12
import math
import os
import random
import re
import sys
# Complete the getSequenceSum function below.
def getSequenceSum(i, j, k):
== '__main__':
name
fptr = open(os.environ ['OUTPUT_PATH'], 'w')
i = int(input().strip())
j = int(input().strip())
k = int(input().strip())
res = getSequenceSum (i, j, k)
fptr.write(str(res) + '\n')
fptr.close()
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 3 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
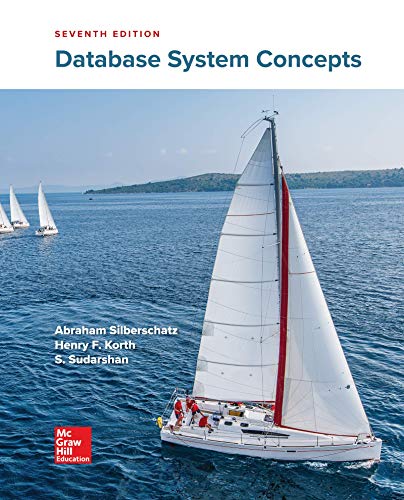
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
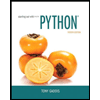
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
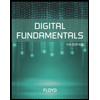
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
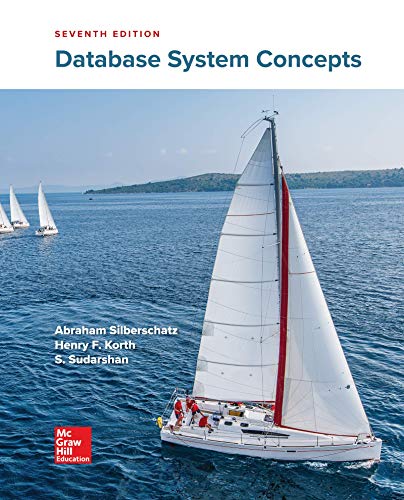
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
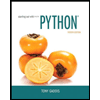
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
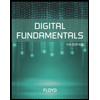
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
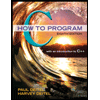
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
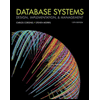
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
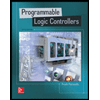
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education