Given the water temperature T in degrees Kelvin, ocean depth D in meters and current speed C in meters per second, the water salinity S in parts-per-hundred (pph) is given as logD+1)VT/223.1 + R VC+1 166 S = 33 where R is the reference salinity for a particular body of water (i.e., a particular ocean). Your task today is to create a program to compute S in pph. You are to query the user to enter the
Given the water temperature T in degrees Kelvin, ocean depth D in meters and current speed C in meters per second, the water salinity S in parts-per-hundred (pph) is given as logD+1)VT/223.1 + R VC+1 166 S = 33 where R is the reference salinity for a particular body of water (i.e., a particular ocean). Your task today is to create a program to compute S in pph. You are to query the user to enter the
C++ Programming: From Problem Analysis to Program Design
8th Edition
ISBN:9781337102087
Author:D. S. Malik
Publisher:D. S. Malik
Chapter5: Control Structures Ii (repetition)
Section: Chapter Questions
Problem 28PE
Related questions
Question
C code, please.

Transcribed Image Text:Practice Practicum 1
Name:
Ocean Salinity
Problem & Instructions:
The salinity of the water in the ocean is a function of the water temperature, ocean depth and
current speed. Additionally, the salinity is highly dependent upon the particular ocean that is
under consideration. Your task today will be to write a program that will serve as an ocean
salinity calculator.
Given the water temperature Tin degrees Kelvin, ocean depth D in meters and current speed C in
meters per second, the water salinity S in parts-per-hundred (pph) is given as
logD+1)\T/223.1
+ R
VC+1
166
S =
33
where R is the reference salinity for a particular body of water (i.e., a particular ocean).
Your task today is to create a program to compute S in pph. You are to query the user to enter the
water temperature T in degrees Celsius (you will need to convert T temporarily to degrees Kelvin
to compute S), ocean depth D in meters and current speed C in meters per second. Assume that
the user enters the data correctly and in the correct units (no error checking on the data format is
necessary). Furthermore, you are to input a single character that will be used to indicate the
reference salinity R for a particular body of water. The choices for reference salinity are the
following: P for the Pacific Ocean, A for the Atlantic Ocean, I for the Indian Ocean, S for the
Southern Ocean and R for the Arctic Ocean. Allow the user to enter either upper or lower case
letters. Furthermore, in the event that the user does not enter a correct character denoting one of
the given oceans, you are to assume that they meant the Pacific Ocean. Based upon the character
the user enters, you will need to choose the correct value for the reference salinity R.
Reference Data:
Table 1: Ocean Reference Salinity in pph
Осean
Reference Salinity (pph)
Pacific
3.0
Atlantic
3.5
Indian
4.0
Southern
2.1
Arctic
2.3
Тетр(°К) — Тетp(°C) + 273. 15

Transcribed Image Text:Practice Practicum 1
Follow these detailed instructions:
1. Start from the provided template code. Include any additional libraries that are
necessary. Use good formatting and style. Match your output to the examples given.
2. Include the usual comment block, including the program name, author, date, class,
professor, description, and notes. Include descriptive comments throughout.
3. Prompt user and scan in values for temperature (in Celsius), depth, and speed.
4. Display the requested output for temperature, depth, and speed. Your output
statement must display as shown in the examples (note spelling, punctuation, newlines, and
lack of trailing zeros):
5. Convert the temperature from Celsius to Kelvin and output as shown.
6 Prompt user and scan in character for ocean name.
а.
The user indicates the ocean choice by entering one of five characters. Assume that
the user may enter upper case or lower case letters. In the event that the user does not
enter one of the five letter choices but something else, use the reference salinity for
the Pacific Ocean. Otherwise, based on their entry, use the proper value for R.
7. Assign the correct reference salinity and print the ocean name.
а.
Write out the name of the Ocean (Pacific, Atlantic, Southern, Arctic or
Indian) that corresponds to the character the user entered. In the case where the
user's ocean choice did not match any of the available options, write out Unknown,
using Pacific for that field.
8. Calculate the salinity using the given equation.
9. Display the salinity and output as shown.
- 3 -
Expert Solution

Step 1 Code for the given question
C code for the given question
#include <math.h>
#include <stdio.h>
int main() {
double s,d,t,c,r;
scanf("%lf", &s);
scanf("%lf", &d);
scanf("%lf", &t);
scanf("%lf", &c);
scanf("%lf", &r);
t=t+273.15;
double x= (5*d/4)+1;
double y =sqrt(t/223.1);
double z = sqrt(c+1);
s=(((166/33)*log(x)*y)/z)+r;
printf("Salinity : %f",s);
}
Step by step
Solved in 2 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
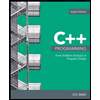
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
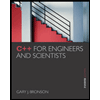
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr
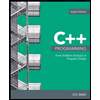
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
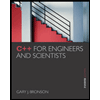
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr