For Checkpoint B you will extend Checkpoint A to do the following: 1. Prompts the user for an additional parameter: the bound on the timescale $n$ of the simulation • If a user inputs a negative timescale, the program should immediately print an error message and exit. 2. The program will calculate , u for every time in and output these populations at each step • If the population grows negative, treat it as population that has become zero. Hint: your program will need a for-loop. Complete this checkpoint after we have introduced for-loops in class. Sample Output Sample input/output behavior for the checkpoint are provided below. Your program's spacing, spelling, capitalization, and punctuation need to match the sample output EXACTLY for this project. Ex 1 Sample Input/Output Given inputs a, B, 7, 8, ko, uo, nas: 1.5 .001 .05 2.5 100 2 10 The program outputs ==>Bull Kelp and Purple Urchin Population Simulator <== --- Model Parameters
For Checkpoint B you will extend Checkpoint A to do the following: 1. Prompts the user for an additional parameter: the bound on the timescale $n$ of the simulation • If a user inputs a negative timescale, the program should immediately print an error message and exit. 2. The program will calculate , u for every time in and output these populations at each step • If the population grows negative, treat it as population that has become zero. Hint: your program will need a for-loop. Complete this checkpoint after we have introduced for-loops in class. Sample Output Sample input/output behavior for the checkpoint are provided below. Your program's spacing, spelling, capitalization, and punctuation need to match the sample output EXACTLY for this project. Ex 1 Sample Input/Output Given inputs a, B, 7, 8, ko, uo, nas: 1.5 .001 .05 2.5 100 2 10 The program outputs ==>Bull Kelp and Purple Urchin Population Simulator <== --- Model Parameters
C++ for Engineers and Scientists
4th Edition
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Bronson, Gary J.
Chapter7: Arrays
Section: Chapter Questions
Problem 9PP: (Data processing) Your professor has asked you to write a C++ program that determines grades at the...
Related questions
Question
How to slove this question when using the code i give

Transcribed Image Text:Checkpoint B
For Checkpoint B you will extend Checkpoint A to do the following:
1. Prompts the user for an additional parameter: the bound on the timescale $n$ of the simulation
• If a user inputs a negative timescale, the program should immediately print an error message and exit.
2. The program will calculate ki, u for every time in and output these populations at each step
• If the population grows negative, treat it as population that has become zero.
Hint: your program will need a for-loop. Complete this checkpoint after we have introduced for-loops in class.
Sample Output
Sample input/output behavior for the checkpoint are provided below. Your program's spacing, spelling, capitalization, and punctuation will
need to match the sample output EXACTLY for this project.
Ex 1 Sample Input/Output
Given inputs a, 6, 7, 8, ko, ug, nas:
1.5
.001
.05
2.5
100
2
10
The program outputs
==>Bull Kelp and Purple Urchin Population Simulator <==
- Model Parameters
Kelp growth rate:
Kelp death rate:
Urchin birth rate:
Urchin death rate:
Initial Population
Kelp population (in thousands) at t = 0:
Urchin population (in thousands) at t = 0:
--- Simulation
---
Timescale:
Time t = 0: 100.000k kelp, 2.000k urchins
249.800k kelp, 7.000k urchins
Time t = 1:
Time t = 2:
622.751k kelp, 76.930k urchins
Time t = 3: 1508.970k kelp, 2280.018k urchins
Time t = 4: 331.946k kelp, 168603.957k urchins
Time t = 5: 0.000k kelp, 2545463.659k urchins
Time t = 6: 0.000k kelp, 0.000k urchins
Time t = 7:
Time t = 8:
Time t = 9: 0.000k kelp, 0.000k urchins
Time t = 10: 0.000k kelp, 0.000k urchins
0.000k kelp, 0.000k urchins
0.000k kelp, 0.000k urchins

Transcribed Image Text:1 print('==> Bull Kelp and Purple Urchin Population Simulator <==\n')
2 print('- Model Parameters ---')
4
5
6
9
print("Error:
exit()
b =float (input("Kelp death rate: \n"))
8 if b<0:
10
16
17
18
19
---
a float (input("Kelp growth rate: \n"))
if a<0:
29
30
31
32
11 c =float
12 if c<0:
13
14
print("Error:
exit()
=
(input("Urchin birth rate: \n"))
print("Error: cannot have a negative birth rate")
exit()
d =float (input("Urchin death rate: \n"))
if d<0:
cannot have a negative growth rate")
print("Error: cannot have a negative death rate")
exit()
k0 max(0, float(input()))
20 u0= max(0, float(input()))
21 k =k0
22 u =u0
23 print('\n--- Initial Population ---')
24 print (f"Kelp population (in thousands) at t = 0: ")
25 print (f"Urchin population (in thousands) at t = 0: \n")
26 print('- Simulation ---')
27
28
cannot have a negative death rate")
=
for t in range (2): # 0, 1
print (f"Time t = {t}: {k:.3f}k kelp, {u:.3f}k urchins")
k_next max(0, k + a*k - b*k*u)
u_next = max(0, u + c*k*u d*u)
k k_next
u =
u_next
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 4 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
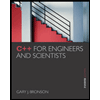
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr
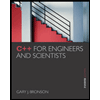
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr