FIX ERRORS GIVEN IN IMAGE #include #include #include using namespace std; typedef float f; class vector3d { public: f x,y,z; vector3d() { x=0; y=0; z=0; } vector3d(f x1,f y1,f z1=0) { x=x1; y=y1; z=z1; } vector3d(const vector3d &vec); vector3d operator+(const vector3d &vec); vector3d &operator+=(const vector3d &vec); vector3d operator-(const vector3d &vec); vector3d &operator-=(const vector3d &vec); vector3d operator*(f value); //multiplication vector3d &operator*=(f value); //assigning new result to the vector. vector3d &operator/=(f value); vector3d &operator=(const vector3d &vec); bool coplanar(vector3d p,vector3d q,vector3d r,vector3d s); f dot_product(const vector3d &vec); vector3d cross_product(const vector3d &vec); f magnitude(); vector3d normalization(); f square(); f distance(const vector3d &vec); //gives distance between two vectors f show_X(); //return x f show_Y(); //return y f show_Z(); //return z void disp(); //display value of vectors }; vector3d::vector3d(const vector3d &vec) { x=vec.x; y=vec.y; z=vec.z; } vector3d vector3d::bool coplanar(vector3d p,vector3d q,vector3d r,vector3d s); { a=distance(const vector3d &p); b=distance(const vector3d &q); e=distance(const vector3d &r); c=distance(const vector3d &s); d=a*p+b*q+c*d; if(d==0) { return true; } else {return false;} } vector3d vector3d ::operator+(const vector3d &vec) { return vector3d(x+vec.x,y+vec.y,z+vec.z); } vector3d &vector3d ::operator+=(const vector3d &vec) { x+=vec.x; y+=vec.y; z+=vec.z; return *this; } //substraction// vector3d vector3d ::operator-(const vector3d &vec) { return vector3d(x-vec.x,y-vec.y,z-vec.z); } vector3d &vector3d::operator-=(const vector3d &vec) { x-=vec.x; y-=vec.y; z-=vec.z; return *this; } //scalar multiplication vector3d vector3d ::operator*(f value) { return vector3d(x*value,y*value,z*value); } vector3d &vector3d::operator*=(f value) { x*=value; y*=value; z*=value; return *this; } vector3d &vector3d ::operator/=(f value) { assert(value!=0); x/=value; y/=value; z/=value; return *this; } vector3d &vector3d::operator=(const vector3d &vec) { x=vec.x; y=vec.y; z=vec.z; return *this; } f vector3d::dot_product(const vector3d &vec) { return x*vec.x+vec.y*y+vec.z*z; } vector3d vector3d ::cross_product(const vector3d &vec) { f ni=y*vec.z-z*vec.y; f nj=z*vec.x-x*vec.z; f nk=x*vec.y-y*vec.x; return vector3d(ni,nj,nk); } f vector3d::magnitude() { return sqrt(square()); } f vector3d::square() { return x*x+y*y+z*z; } vector3d vector3d:: normalization() { assert(magnitude()!=0); *this/=magnitude(); return *this; } f vector3d::distance(const vector3d &vec) { vector3d dist=*this-vec; return dist.magnitude(); } f vector3d::show_X() { return x; } f vector3d::show_Y() { return y; } f vector3d::show_Z() { return z; } void vector3d::disp() { cout<
FIX ERRORS GIVEN IN IMAGE
#include<iostream>
#include<math.h>
#include<assert.h>
using namespace std;
typedef float f;
class vector3d
{
public:
f x,y,z;
vector3d() {
x=0;
y=0;
z=0;
}
vector3d(f x1,f y1,f z1=0) {
x=x1;
y=y1;
z=z1;
}
vector3d(const vector3d &vec);
vector3d operator+(const vector3d &vec);
vector3d &operator+=(const vector3d &vec);
vector3d operator-(const vector3d &vec);
vector3d &operator-=(const vector3d &vec);
vector3d operator*(f value); //multiplication
vector3d &operator*=(f value); //assigning new result to the vector.
vector3d &operator/=(f value);
vector3d &operator=(const vector3d &vec);
bool coplanar(vector3d p,vector3d q,vector3d r,vector3d s);
f dot_product(const vector3d &vec);
vector3d cross_product(const vector3d &vec);
f magnitude();
vector3d normalization();
f square();
f distance(const vector3d &vec); //gives distance between two
f show_X(); //return x
f show_Y(); //return y
f show_Z(); //return z
void disp(); //display value of vectors
};
vector3d::vector3d(const vector3d &vec)
{
x=vec.x;
y=vec.y;
z=vec.z;
}
vector3d vector3d::bool coplanar(vector3d p,vector3d q,vector3d r,vector3d s);
{
a=distance(const vector3d &p);
b=distance(const vector3d &q);
e=distance(const vector3d &r);
c=distance(const vector3d &s);
d=a*p+b*q+c*d;
if(d==0)
{
return true;
}
else {return false;}
}
vector3d vector3d ::operator+(const vector3d &vec)
{
return vector3d(x+vec.x,y+vec.y,z+vec.z);
}
vector3d &vector3d ::operator+=(const vector3d &vec)
{
x+=vec.x;
y+=vec.y;
z+=vec.z;
return *this;
}
//substraction//
vector3d vector3d ::operator-(const vector3d &vec)
{
return vector3d(x-vec.x,y-vec.y,z-vec.z);
}
vector3d &vector3d::operator-=(const vector3d &vec)
{
x-=vec.x;
y-=vec.y;
z-=vec.z;
return *this;
}
//scalar multiplication
vector3d vector3d ::operator*(f value)
{
return vector3d(x*value,y*value,z*value);
}
vector3d &vector3d::operator*=(f value)
{
x*=value;
y*=value;
z*=value;
return *this;
}
vector3d &vector3d ::operator/=(f value)
{
assert(value!=0);
x/=value;
y/=value;
z/=value;
return *this;
}
vector3d &vector3d::operator=(const vector3d &vec)
{
x=vec.x;
y=vec.y;
z=vec.z;
return *this;
}
f vector3d::dot_product(const vector3d &vec)
{
return x*vec.x+vec.y*y+vec.z*z;
}
vector3d vector3d ::cross_product(const vector3d &vec)
{
f ni=y*vec.z-z*vec.y;
f nj=z*vec.x-x*vec.z;
f nk=x*vec.y-y*vec.x;
return vector3d(ni,nj,nk);
}
f vector3d::magnitude()
{
return sqrt(square());
}
f vector3d::square()
{
return x*x+y*y+z*z;
}
vector3d vector3d:: normalization()
{
assert(magnitude()!=0);
*this/=magnitude();
return *this;
}
f vector3d::distance(const vector3d &vec)
{
vector3d dist=*this-vec;
return dist.magnitude();
}
f vector3d::show_X()
{
return x;
}
f vector3d::show_Y()
{
return y;
}
f vector3d::show_Z()
{
return z;
}
void vector3d::disp()
{
cout<<x<<" "<<y<<" "<<z<<endl;
if(x<y)
cout<<"coplanar";
else
cout<<"No coplanar";
}
int main()
{
vector3d a=vector3d(2,5,7);
vector3d b=vector3d(3,7,10);
vector3d d=vector3d(3,4,11);
vector3d c=a+b+d;
c.disp();
// call other functions
}


Step by step
Solved in 2 steps with 1 images

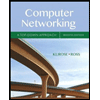
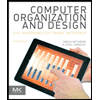
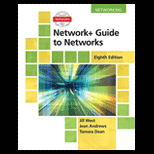
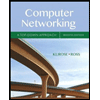
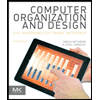
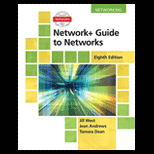
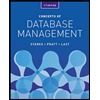
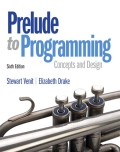
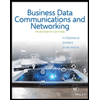