Every cylinder has a base and height, where the base is a circle. Design the class Cylinder that can capture the properties of a cylinder and perform the usual operations on a cylinder. Derive this class from the class Circle (done through Problem 3.2). Please do the following: Some of the operations that can be performed on a cylinder are as follows: calculate and print the volume, calculate and print the surface area, set the height, set the radius of the base, and set the center of the base. Write a test program to test your program. Note: Java programming. Please also send the test program so I can run it in dr java and learn. Use given code and design the class cylinder that can capture the properties of a cylinder and perform the usual operations on a cylinder. Point code: // class Point public class Point { protected double x, y; // coordinates of the Point //Default constructor public Point() { setPoint( 0, 0 ); } //Constructor with parameters public Point(double xValue, double yValue ) { setPoint(xValue, yValue ); } // set x and y coordinates of Point public void setPoint(double xValue, double yValue ) { x = xValue; y = yValue; } // get x coordinate public double getX() { return x; } // get y coordinate public double getY() { return y; } // convert point into String representation public String toString() { return "[" + String.format("%.2f", x) + ", " + String.format("%.2f", y) + "]"; } //Method to compare two points public boolean equals(Point otherPoint) { return(x == otherPoint.x && y == otherPoint.y); } //Method to compare two points public void makeCopy(Point otherPoint) { x = otherPoint.x; y = otherPoint.y; } public Point getCopy() { Point temp = new Point(); temp.x = x; temp.y = y; return temp; } // print method public void printPoint() { System.out.print("[" + String.format("%.2f", x) + ", " + String.format("%.2f", y) + "]"); } } // end class Point Circle code: public class Circle { //declaring data members protected double radius; protected Point center; //constructor public Circle() { radius=0; center=new Point(); } //parameterized constructor public Circle(Point p, double radius) { center=p; this.radius=radius; } //getters and setters for radius public double getRadius() { return radius; } public void setRadius(double radius) { this.radius=radius; } //getters and setters for center public void setCenter(Point p) { center=p; } public Point getPoint() { return center; } //finding area public double getArea() { return 3.14*radius*radius; } //finding circumference public double getCircumference() { return 2*3.14*radius; } }
Every cylinder has a base and height, where the base is a circle. Design the class Cylinder that can capture the properties of a cylinder and perform the usual operations on a cylinder. Derive this class from the class Circle (done through Problem 3.2). Please do the following:
- Some of the operations that can be performed on a cylinder are as follows: calculate and print the volume, calculate and print the surface area, set the height, set the radius of the base, and set the center of the base.
- Write a test program to test your program.
Note: Java programming. Please also send the test program so I can run it in dr java and learn. Use given code and design the class cylinder that can capture the properties of a cylinder and perform the usual operations on a cylinder.
Point code:
// class Point
public class Point
{
protected double x, y; // coordinates of the Point
//Default constructor
public Point()
{
setPoint( 0, 0 );
}
//Constructor with parameters
public Point(double xValue, double yValue )
{
setPoint(xValue, yValue );
}
// set x and y coordinates of Point
public void setPoint(double xValue, double yValue )
{
x = xValue;
y = yValue;
}
// get x coordinate
public double getX()
{
return x;
}
// get y coordinate
public double getY()
{
return y;
}
// convert point into String representation
public String toString()
{
return "[" + String.format("%.2f", x)
+ ", " + String.format("%.2f", y) + "]";
}
//Method to compare two points
public boolean equals(Point otherPoint)
{
return(x == otherPoint.x &&
y == otherPoint.y);
}
//Method to compare two points
public void makeCopy(Point otherPoint)
{
x = otherPoint.x;
y = otherPoint.y;
}
public Point getCopy()
{
Point temp = new Point();
temp.x = x;
temp.y = y;
return temp;
}
// print method
public void printPoint()
{
System.out.print("[" + String.format("%.2f", x)
+ ", " + String.format("%.2f", y) + "]");
}
} // end class Point
Circle code:
public class Circle
{
//declaring data members
protected double radius;
protected Point center;
//constructor
public Circle()
{
radius=0;
center=new Point();
}
//parameterized constructor
public Circle(Point p, double radius)
{
center=p;
this.radius=radius;
}
//getters and setters for radius
public double getRadius()
{
return radius;
}
public void setRadius(double radius)
{
this.radius=radius;
}
//getters and setters for center
public void setCenter(Point p)
{
center=p;
}
public Point getPoint()
{
return center;
}
//finding area
public double getArea()
{
return 3.14*radius*radius;
}
//finding circumference
public double getCircumference()
{
return 2*3.14*radius;
}
}

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 2 images

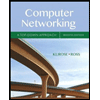
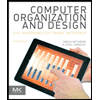
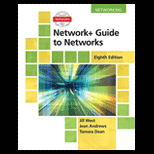
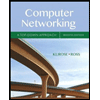
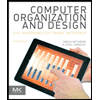
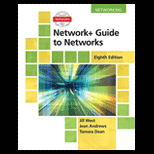
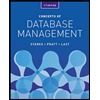
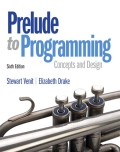
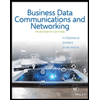