Do not add statements that call print , input , or open , or add an tmport statement. • Do not use any break or continue statements. We are imposing this restriction (and we have not even taught you these statements) because they are very easy to abuse, resulting in terrible, hard to read code. Do not modify or add to the import statements provided in the starter code. import math from typing import List, TextI0 # For simplicity, we'll use "Station" in our type contracts to indicate that # we mean a list containing station data. 2#3 # You can read "Station" in a type contract as: List[int, str, float, float, int, int, int] %23 23 # where the values at each index represent the station data as described in the # handout on Quercus. # A set of constants, each representing a list index for station information. ID - 0 NAME - 1 LATITUDE - 2 LONGITUDE - 3 САРАСITY - 4 BIKES_AVAILABLE - 5 DOCKS_AVAILABLE - 6 "SMART NO_KIOSK
Do not add statements that call print , input , or open , or add an tmport statement. • Do not use any break or continue statements. We are imposing this restriction (and we have not even taught you these statements) because they are very easy to abuse, resulting in terrible, hard to read code. Do not modify or add to the import statements provided in the starter code. import math from typing import List, TextI0 # For simplicity, we'll use "Station" in our type contracts to indicate that # we mean a list containing station data. 2#3 # You can read "Station" in a type contract as: List[int, str, float, float, int, int, int] %23 23 # where the values at each index represent the station data as described in the # handout on Quercus. # A set of constants, each representing a list index for station information. ID - 0 NAME - 1 LATITUDE - 2 LONGITUDE - 3 САРАСITY - 4 BIKES_AVAILABLE - 5 DOCKS_AVAILABLE - 6 "SMART NO_KIOSK
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
![• Do not add statements that call print, input , or open , or add an import statement.
• Do not use any break or continue statements. We are imposing this restriction (and we have not even taught you
these statements) because they are very easy to abuse, resulting in terrible, hard to read code.
• Do not modify or add to the import statements provided in the starter code.
import math
from typing import List, TextIO
# For simplicity, we'll use "Station" in our type contracts to indicate that
# we mean a list containing station data.
# You can read "Station" in a type contract as:
List[int, str, float, float, int, int, int]
23
# where the values at each index represent the station data as described in the
# handout on Quercus.
# A set of constants, each representing a list index for station information.
ID - 0
NAME - 1
LATITUDE - 2
LONGITUDE - 3
CAPACITY - 4
BIKES_AVAILABLE - 5
DOCKS_AVAILABLE - 6
NO_KIOSK - 'SMART"
# For use in the get_lat_lon_distance helper function
EARTH RADIUS - 6371
# SAMPLE DATA TO USE IN DOCSTRING EXAMPLES
SAMPLE_STATIONS - [
[7090, 'Danforth Ave / Lamb Ave',
43.681991, -79.329455, 15, 4, 10],
[7486, "Gerrard St E / Ted Reeve Dr',
43.684261, -79.299332, 24, 5, 19],
[7571, 'Highfield Rd / Gerard St E - SMART",
43.671685, -79.325176, 19, 14, 5]]
HANDOUT_STATIONS - [
[7000, 'Ft. York / Capreol Crt.',
43.639832, -79.395954, 31, 20, 11],
[7001, 'Lower Jarvis St SMART / The Esplanade',
43.647992, -79.370907, 15, 5, 10]]](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F5da1a8b3-4dda-44c5-977e-a3d5025a2246%2Fceee62a5-6263-4ca4-8c00-057f0bf604ed%2F830np6t_processed.png&w=3840&q=75)
Transcribed Image Text:• Do not add statements that call print, input , or open , or add an import statement.
• Do not use any break or continue statements. We are imposing this restriction (and we have not even taught you
these statements) because they are very easy to abuse, resulting in terrible, hard to read code.
• Do not modify or add to the import statements provided in the starter code.
import math
from typing import List, TextIO
# For simplicity, we'll use "Station" in our type contracts to indicate that
# we mean a list containing station data.
# You can read "Station" in a type contract as:
List[int, str, float, float, int, int, int]
23
# where the values at each index represent the station data as described in the
# handout on Quercus.
# A set of constants, each representing a list index for station information.
ID - 0
NAME - 1
LATITUDE - 2
LONGITUDE - 3
CAPACITY - 4
BIKES_AVAILABLE - 5
DOCKS_AVAILABLE - 6
NO_KIOSK - 'SMART"
# For use in the get_lat_lon_distance helper function
EARTH RADIUS - 6371
# SAMPLE DATA TO USE IN DOCSTRING EXAMPLES
SAMPLE_STATIONS - [
[7090, 'Danforth Ave / Lamb Ave',
43.681991, -79.329455, 15, 4, 10],
[7486, "Gerrard St E / Ted Reeve Dr',
43.684261, -79.299332, 24, 5, 19],
[7571, 'Highfield Rd / Gerard St E - SMART",
43.671685, -79.325176, 19, 14, 5]]
HANDOUT_STATIONS - [
[7000, 'Ft. York / Capreol Crt.',
43.639832, -79.395954, 31, 20, 11],
[7001, 'Lower Jarvis St SMART / The Esplanade',
43.647992, -79.370907, 15, 5, 10]]
![def upgrade stations (threshold: int, num bikes: int,
stations: List["Station"]) -> int:
"" "Modify each station in stations that has a capacity that is less than
threshold by adding num bikes to the capacity and bikes available counts.
Modify each station at most once.
Return the total number of bikes that were added to the bike share network.
Precondition: num bikes >= 0
>>> handout_copy = [HANDOUT_STATIONS[0] [:1, HANDOUT_STATIONS [1] [:]]
>>> upgrade_stations (25, 5, handout_copy)
5
>>> handout_copy[0]
== HANDOUT STATIONS [0]
True
== [7001, 'Lower Jarvis St SMART / The Esplanade', \
43.647992, -79.370907, 20, 10, 10]
>>> handout_copy[1]
True](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F5da1a8b3-4dda-44c5-977e-a3d5025a2246%2Fceee62a5-6263-4ca4-8c00-057f0bf604ed%2Fngzi5dx_processed.png&w=3840&q=75)
Transcribed Image Text:def upgrade stations (threshold: int, num bikes: int,
stations: List["Station"]) -> int:
"" "Modify each station in stations that has a capacity that is less than
threshold by adding num bikes to the capacity and bikes available counts.
Modify each station at most once.
Return the total number of bikes that were added to the bike share network.
Precondition: num bikes >= 0
>>> handout_copy = [HANDOUT_STATIONS[0] [:1, HANDOUT_STATIONS [1] [:]]
>>> upgrade_stations (25, 5, handout_copy)
5
>>> handout_copy[0]
== HANDOUT STATIONS [0]
True
== [7001, 'Lower Jarvis St SMART / The Esplanade', \
43.647992, -79.370907, 20, 10, 10]
>>> handout_copy[1]
True
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 2 steps with 2 images

Recommended textbooks for you
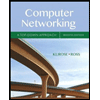
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
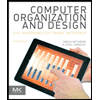
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
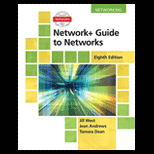
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
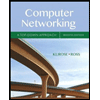
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
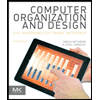
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
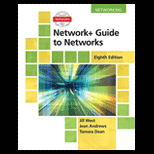
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
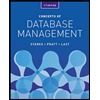
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
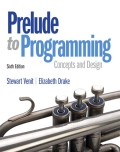
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
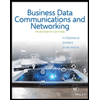
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY