def cost_to_hike_naive(m: list[list[int]], start_point: tuple[int, int], end_point: tuple[int, int]) -> int: """ Given an elevation map and a start and end point, calculate the cost it would take to hike from to . If the start and end points are the same, then return 0. Some definitions and rules: 1. You can only hike to either a vertically or horizontally adjacent location (you cannot travel diagonally). 2. You must only travel in the direction of the . More explicitly, this means that any move you make MUST take you closer to the end point, so you cannot travel in the other direction. 3. We define the cost to travel between two adjacent blocks as the absolute difference in elevation between those blocks. 4. You will calculate the naive route here, so at every position, you will have two choices, to either move closer to the end point along a row, or along a column, and you will chose the position that costs the least at that point (you do not need to look ahead to see if the costlier route now will result in an overall cheaper route). 5. If two choices along the route have the exact same cost, you will choose the direction that comes first clockwise. For example, you will choose to move horizontally right before vertically down, vertically down before horizontally left, horizontally left before vertically up, and vertically up before horizontally right. Preconditions: the start and end points will be a valid position on the map. >>> sample_map = [[1, 3], ... [0, 4]] >>> cost_to_hike_naive(sample_map, (0, 0), (1, 1)) 5 >>> cost_to_hike_naive(sample_map, (1, 1), (0, 0)) 3
RESTRICTIONS:
- Do not add any imports and do it on python .Do not use recursion. Do not use break/continue.Do not use try-except statements
def cost_to_hike_naive(m: list[list[int]], start_point: tuple[int, int],
end_point: tuple[int, int]) -> int:
"""
Given an elevation map <m> and a start and end point, calculate the cost it
would take to hike from <start_point> to <end_point>. If the start and end
points are the same, then return 0.
Some definitions and rules:
1. You can only hike to either a vertically or horizontally adjacent
location (you cannot travel diagonally).
2. You must only travel in the direction of the <end_point>. More
explicitly, this means that any move you make MUST take you closer
to the end point, so you cannot travel in the other direction.
3. We define the cost to travel between two adjacent blocks as the
absolute difference in elevation between those blocks.
4. You will calculate the naive route here, so at every position, you
will have two choices, to either move closer to the end point along
a row, or along a column, and you will chose the position that costs
the least at that point (you do not need to look ahead to see if the
costlier route now will result in an overall cheaper route).
5. If two choices along the route have the exact same cost, you will
choose the direction that comes first clockwise. For example, you
will choose to move horizontally right before vertically down,
vertically down before horizontally left, horizontally left before
vertically up, and vertically up before horizontally right.
Preconditions:
the start and end points will be a valid position on the map.
Tips to get started:
since there is no guarantee where the start and end points will be in
relation to each other, it may be easier to use your rotate function
from last week to rotate the map such that the end point is always to
the bottom right of the start point. That is, rotate the map such that
start_point[0] <= end_point[0] and start_point[1] <= end_point[1].
Keep in mind that if you choose to rotate the map, the start and end
point coordinates will change as well. If you choose to do it this way,
then you can code your function to only consider moving horizontally
right, or vertically down. Not rotating is fine as well, but you would
have to consider moves in all 4 directions, depending on the start and
end coordinates. If you choose to rotate the map, you may also find the
crop function you implmented last week useful, as that will guarantee
that the start and end points will be at corners of the map.
>>> sample_map = [[1, 3],
... [0, 4]]
>>> cost_to_hike_naive(sample_map, (0, 0), (1, 1))
5
>>> cost_to_hike_naive(sample_map, (1, 1), (0, 0))
3
"""

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 3 images

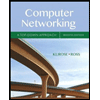
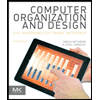
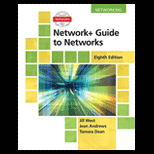
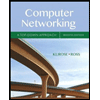
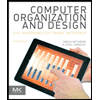
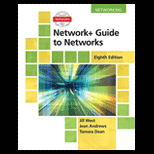
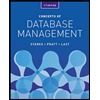
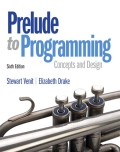
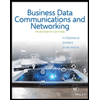