Create an abstract Student class for Parker University. The class contains fields for student ID number, last name, and annual tuition. Include a constructor that requires parameters for the ID number and name. Include get and set methods for each field; the setTuition() method is abstract. Create three Student subclasses named UndergraduateStudent, GraduateStudent, and StudentAtLarge, each with a unique setTuition() method. Tuition for an UndergraduateStudent is $4,000 per semester, tuition for a GraduateStudent is $6,000 per semester, and tuition for a StudentAtLarge is $2,000 per semester. Code I was given - public class GraduateStudent extends Student { public static final double GRAD_TUITION = 6000; public GraduateStudent(String id, String name) { // write your code here } public void setTuition() { tuition = GRAD_TUITION; } } public abstract class Student { private String id; private String lastName; protected double tuition; public Student(String id, String name) { // write your code here } public void setId(String idNum) { // write your code here } public void setLastName(String name) { // write your code here } public String getId() { // write your code here } public String getLastName() { // write your code here } public double getTuition() { // write your code here } public abstract void setTuition(); } public class StudentAtLarge extends Student { public static final double SAL_TUITION = 2000; public StudentAtLarge (String id, String name) { // write your code here } public void setTuition() { tuition = SAL_TUITION; } } public class StudentDemo { public static void main(String[] args) { Student students[] = new Student[6]; int i; students[0] = new UndergraduateStudent("111", "Lambert"); students[1] = new UndergraduateStudent("122", "Lembeck"); students[2] = new GraduateStudent("233", "Miller"); students[3] = new GraduateStudent("256", "Marmon"); students[4] = new StudentAtLarge("312", "Nichols"); students[5] = new StudentAtLarge("376", "Nussbaum"); for(i = 0; i < students.length; ++i) System.out.println("\nStudent # " + students[i].getId() + " Name: " + students[i].getLastName() + " Tuition: " + students[i].getTuition() + " per year"); } } public class UndergraduateStudent extends Student { public static final double UNDERGRAD_TUITION = 4000; public UndergraduateStudent(String id, String name) { // write your code here } public void setTuition() { tuition = UNDERGRAD_TUITION; } }
Create an abstract Student class for Parker University. The class contains fields for student ID number, last name, and annual tuition. Include a constructor that requires parameters for the ID number and name. Include get and set methods for each field; the setTuition() method is abstract. Create three Student subclasses named UndergraduateStudent, GraduateStudent, and StudentAtLarge, each with a unique setTuition() method. Tuition for an UndergraduateStudent is $4,000 per semester, tuition for a GraduateStudent is $6,000 per semester, and tuition for a StudentAtLarge is $2,000 per semester. Code I was given - public class GraduateStudent extends Student { public static final double GRAD_TUITION = 6000; public GraduateStudent(String id, String name) { // write your code here } public void setTuition() { tuition = GRAD_TUITION; } } public abstract class Student { private String id; private String lastName; protected double tuition; public Student(String id, String name) { // write your code here } public void setId(String idNum) { // write your code here } public void setLastName(String name) { // write your code here } public String getId() { // write your code here } public String getLastName() { // write your code here } public double getTuition() { // write your code here } public abstract void setTuition(); } public class StudentAtLarge extends Student { public static final double SAL_TUITION = 2000; public StudentAtLarge (String id, String name) { // write your code here } public void setTuition() { tuition = SAL_TUITION; } } public class StudentDemo { public static void main(String[] args) { Student students[] = new Student[6]; int i; students[0] = new UndergraduateStudent("111", "Lambert"); students[1] = new UndergraduateStudent("122", "Lembeck"); students[2] = new GraduateStudent("233", "Miller"); students[3] = new GraduateStudent("256", "Marmon"); students[4] = new StudentAtLarge("312", "Nichols"); students[5] = new StudentAtLarge("376", "Nussbaum"); for(i = 0; i < students.length; ++i) System.out.println("\nStudent # " + students[i].getId() + " Name: " + students[i].getLastName() + " Tuition: " + students[i].getTuition() + " per year"); } } public class UndergraduateStudent extends Student { public static final double UNDERGRAD_TUITION = 4000; public UndergraduateStudent(String id, String name) { // write your code here } public void setTuition() { tuition = UNDERGRAD_TUITION; } }
Chapter11: Advanced Inheritance Concepts
Section: Chapter Questions
Problem 7PE
Related questions
Question
This is the question -
Create an abstract Student class for Parker University. The class contains fields for student ID number, last name, and annual tuition. Include a constructor that requires parameters for the ID number and name. Include get and set methods for each field; the setTuition() method is abstract.
Create three Student subclasses named UndergraduateStudent, GraduateStudent, and StudentAtLarge, each with a unique setTuition() method. Tuition for an UndergraduateStudent is $4,000 per semester, tuition for a GraduateStudent is $6,000 per semester, and tuition for a StudentAtLarge is $2,000 per semester.
Code I was given -
public class GraduateStudent extends Student
{
public static final double GRAD_TUITION = 6000;
public GraduateStudent(String id, String name)
{
// write your code here
}
public void setTuition()
{
tuition = GRAD_TUITION;
}
}
public abstract class Student
{
private String id;
private String lastName;
protected double tuition;
public Student(String id, String name)
{
// write your code here
}
public void setId(String idNum)
{
// write your code here
}
public void setLastName(String name)
{
// write your code here
}
public String getId()
{
// write your code here
}
public String getLastName()
{
// write your code here
}
public double getTuition()
{
// write your code here
}
public abstract void setTuition();
}
public class StudentAtLarge extends Student
{
public static final double SAL_TUITION = 2000;
public StudentAtLarge (String id, String name)
{
// write your code here
}
public void setTuition()
{
tuition = SAL_TUITION;
}
}
public class StudentDemo
{
public static void main(String[] args)
{
Student students[] = new Student[6];
int i;
students[0] = new UndergraduateStudent("111", "Lambert");
students[1] = new UndergraduateStudent("122", "Lembeck");
students[2] = new GraduateStudent("233", "Miller");
students[3] = new GraduateStudent("256", "Marmon");
students[4] = new StudentAtLarge("312", "Nichols");
students[5] = new StudentAtLarge("376", "Nussbaum");
for(i = 0; i < students.length; ++i)
System.out.println("\nStudent # " +
students[i].getId() + " Name: " +
students[i].getLastName() + " Tuition: " +
students[i].getTuition() + " per year");
}
}
public class UndergraduateStudent extends Student
{
public static final double UNDERGRAD_TUITION = 4000;
public UndergraduateStudent(String id, String name)
{
// write your code here
}
public void setTuition()
{
tuition = UNDERGRAD_TUITION;
}
}
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 6 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
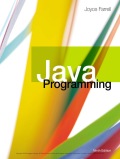
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
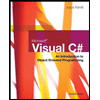
Microsoft Visual C#
Computer Science
ISBN:
9781337102100
Author:
Joyce, Farrell.
Publisher:
Cengage Learning,
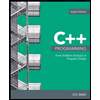
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
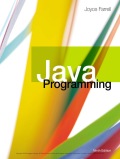
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
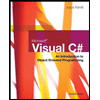
Microsoft Visual C#
Computer Science
ISBN:
9781337102100
Author:
Joyce, Farrell.
Publisher:
Cengage Learning,
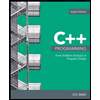
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning