Create a Post class, with separate interface (Post.h) and implementation (Post.cpp) files, comprised of the following attributes: Data Members (private) Data Members string: body_ string: post_author__ int: num_likes_ string: date_ Member Functions (public) Default: constructor Member Functions Description The main body (content) of the post Author of the post Number of likes the post has received. Date the post was made, in mm/dd/yy format Parameterized: constructor string: getPostBody() void setPostBody(string post_body) string: getPostAuthor() void: setPostAuthor(string author) int: getPostLikes () void setPostLikes (int likes) string: getPostDate() void setPostDate(string post_date) } int main() { Description Sets body, post_author_, date_ to empty string and num_likes_ to 0 Takes a string for body, string for post_author_, int for num_likes_, and string for date_ this order) for initializing the data members of a Post object Returns the body of the post Sets new body for the post Returns the post_author__ of the post Sets the new post_author__ for the post The zip submission should have three files for this problem: return 0; Returns num_likes_ that the post has received Sets num_likes_ data member to likes if likes is a positive integer or 0. Returns the date of the post Post.h Post.cpp • A driver file called postDriver.cpp, with a main() function to test your member functions. • In your main() function, the test cases should include the creation of class objects with both the default and parameterized constructors. You must also test each of the getter and setter member functions by creating and manipulating class objects using assert tests to verify that things are working properly. You will only have one main function which is in this driver file. an example of calling some Post member functions in your main method in your postDriver.cpp file. Sets new date for the post Post my_post_1 = Post(); // default constructor cout << "Post author " << my_post_1.getPostAuthor() << endl; cout << "Post body " << my_post_1.getPostBody() << endl; cout << "Post date " << my_post_1.getPostDate() << endl; cout << "Post likes " << my_post_1.getPostLikes() << endl; Post my_post_2 = Post("new post","Raegan", 10, "10/02/22"); // parameterized constructor cout << "Post author " << my_post_2.getPostAuthor() << endl; cout << "Post body " << my_post_2.getPostBody() << endl; cout << "Post date " << my_post_2.getPostDate() << endl; cout << "Post likes " << my_post_2.getPostLikes() << endl;
Create a Post class, with separate interface (Post.h) and implementation (Post.cpp) files, comprised of the following attributes: Data Members (private) Data Members string: body_ string: post_author__ int: num_likes_ string: date_ Member Functions (public) Default: constructor Member Functions Description The main body (content) of the post Author of the post Number of likes the post has received. Date the post was made, in mm/dd/yy format Parameterized: constructor string: getPostBody() void setPostBody(string post_body) string: getPostAuthor() void: setPostAuthor(string author) int: getPostLikes () void setPostLikes (int likes) string: getPostDate() void setPostDate(string post_date) } int main() { Description Sets body, post_author_, date_ to empty string and num_likes_ to 0 Takes a string for body, string for post_author_, int for num_likes_, and string for date_ this order) for initializing the data members of a Post object Returns the body of the post Sets new body for the post Returns the post_author__ of the post Sets the new post_author__ for the post The zip submission should have three files for this problem: return 0; Returns num_likes_ that the post has received Sets num_likes_ data member to likes if likes is a positive integer or 0. Returns the date of the post Post.h Post.cpp • A driver file called postDriver.cpp, with a main() function to test your member functions. • In your main() function, the test cases should include the creation of class objects with both the default and parameterized constructors. You must also test each of the getter and setter member functions by creating and manipulating class objects using assert tests to verify that things are working properly. You will only have one main function which is in this driver file. an example of calling some Post member functions in your main method in your postDriver.cpp file. Sets new date for the post Post my_post_1 = Post(); // default constructor cout << "Post author " << my_post_1.getPostAuthor() << endl; cout << "Post body " << my_post_1.getPostBody() << endl; cout << "Post date " << my_post_1.getPostDate() << endl; cout << "Post likes " << my_post_1.getPostLikes() << endl; Post my_post_2 = Post("new post","Raegan", 10, "10/02/22"); // parameterized constructor cout << "Post author " << my_post_2.getPostAuthor() << endl; cout << "Post body " << my_post_2.getPostBody() << endl; cout << "Post date " << my_post_2.getPostDate() << endl; cout << "Post likes " << my_post_2.getPostLikes() << endl;
Chapter11: Advanced Inheritance Concepts
Section: Chapter Questions
Problem 3PE
Related questions
Question
In C++

Transcribed Image Text:Create a Post class, with separate interface (Post.h) and implementation (Post.cpp) files, comprised of the following attributes:
Data Members (private)
Data Members
string: body_
string: post_author__
int: num_likes_
string: date_
Member Functions (public)
Default: constructor
Member Functions
Description
The main body (content) of the post
Author of the post
Number of likes the post has received.
Date the post was made, in mm/dd/yy format
Parameterized: constructor
string: getPostBody()
void setPostBody(string
post_body)
string: getPostAuthor()
void: setPostAuthor(string
author)
int: getPostLikes ()
void setPostLikes (int likes)
string: getPostDate()
void setPostDate(string
post_date)
}
int main()
{
Description
Sets body, post_author_, date_ to empty string and num_likes_ to 0
Takes a string for body, string for post_author_, int for num_likes_, and string for date_ (in
this order) for initializing the data members of a Post object
Returns the body of the post
Sets new body for the post
Returns the post_author_ of the post
Sets the new post_author_ for the post
The zip submission should have three files for this problem:
Returns num_likes that the post has received
Sets num_likes_ data member to likes if likes is a positive integer or 0.
Returns the date of the post
return 0;
Sets new date for the post
Post.h
Post.cpp
A driver file called postDriver.cpp, with a main() function to test your member functions.
• In your main () function, the test cases should include the creation of class objects with both the default and parameterized
constructors. You must also test each of the getter and setter member functions by creating and manipulating class objects and
using assert tests to verify that things are working properly. You will only have one main function which is in this driver file. Here is
an example of calling some Post member functions in your main method in your postDriver.cpp file.
Post my_post_1 = Post(); // default constructor
cout << "Post author " << my_post_1.getPostAuthor() << endl;
cout << "Post body " << my_post_1.getPostBody() << endl;
cout << "Post date " << my_post_1.getPostDate() << endl;
cout << "Post likes " << my_post_1.getPostLikes () << endl;
Post my_post_2 = Post("new post","Raegan", 10, "10/02/22"); // parameterized constructor
cout << "Post author " << my_post_2.getPostAuthor() << endl;
cout << "Post body " << my_post_2.getPostBody() << endl;
cout << "Post date " << my_post_2.getPostDate() << endl;
cout << "Post likes " << my_post_2.getPostLikes() << endl;
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
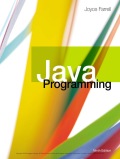
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
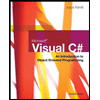
Microsoft Visual C#
Computer Science
ISBN:
9781337102100
Author:
Joyce, Farrell.
Publisher:
Cengage Learning,
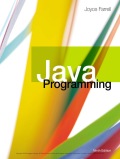
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
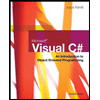
Microsoft Visual C#
Computer Science
ISBN:
9781337102100
Author:
Joyce, Farrell.
Publisher:
Cengage Learning,