Consider the IntArrayBag data structure that is represented by the following UML. IntArrayBag -data:int[] -manyltems:int +IntArrayBag() +IntArrayBag(capacity:int) +add(element:int):void +countOcuurances(target:int):int +grab(index:i):int +remove(target:int):void +size():int +max():int +countEven():int +change(oldVal:int, newVal:int):int (1) Implement an instance method, called bagMax, in the IntArrayBag class that finds and return the maximum value in the bag. (2) Implement an instance method, called equals To, in the IntArrayBag's class that takes one input parameter and returns a boolean value. The input parameter, called otherBag, is of type IntArrayBag as well. The method returns true if otherBag and the bag that activates the method have exactly the same number of every element. Otherwise the method return false. Note that the locations of the elements in the data arrays are not necessarily the same, it is only the number of occurrences of each element that must be the same. (3) Implement a static method, called sumEven, in the IntArrayBag class that takes one input parameter of type IntArrayBag. The method then calculates and returns the sum of all even elements in the bag. (4) Re-implement the static sumEven method, in the IntArrayBag class that takes one input parameter of type IntArrayBag. The method then calculates and returns the sum of all even elements in the bag.
Consider the IntArrayBag data structure that is represented by the following UML. IntArrayBag -data:int[] -manyltems:int +IntArrayBag() +IntArrayBag(capacity:int) +add(element:int):void +countOcuurances(target:int):int +grab(index:i):int +remove(target:int):void +size():int +max():int +countEven():int +change(oldVal:int, newVal:int):int (1) Implement an instance method, called bagMax, in the IntArrayBag class that finds and return the maximum value in the bag. (2) Implement an instance method, called equals To, in the IntArrayBag's class that takes one input parameter and returns a boolean value. The input parameter, called otherBag, is of type IntArrayBag as well. The method returns true if otherBag and the bag that activates the method have exactly the same number of every element. Otherwise the method return false. Note that the locations of the elements in the data arrays are not necessarily the same, it is only the number of occurrences of each element that must be the same. (3) Implement a static method, called sumEven, in the IntArrayBag class that takes one input parameter of type IntArrayBag. The method then calculates and returns the sum of all even elements in the bag. (4) Re-implement the static sumEven method, in the IntArrayBag class that takes one input parameter of type IntArrayBag. The method then calculates and returns the sum of all even elements in the bag.
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
![100% +
Consider the IntArrayBag data structure that is represented by the following UML.
IntArrayBag
-data:int[]
manyltems:int
+IntArrayBag()
+IntArrayBag(capacity:int)
+add(element:int) void
+countOcuurances(target:int):int
+grab(index:i):int
+remove(target:int):void
+size():int
+max():int
+countEven():int
+change(oldVal:int, newVal:int):int
(1) Implement an instance method, called bagMax, in the IntArrayBag class that finds and
return the maximum value in the bag.
(2) Implement an instance method, called equalsTo, in the IntArrayBag's class that takes
one input parameter and returns a boolean value. The input parameter, called otherBag,
is of type IntArrayBag as well. The method returns true if otherBag and the bag that
activates the method have exactly the same number of every element. Otherwise the method
return false. Note that the locations of the elements in the data arrays are not necessarily
the same, it is only the number of occurrences of each element that must be the same.
(3) Implement a static method, called sumEven, in the IntArrayBag class that takes one
input parameter of type IntArrayBag. The method then calculates and returns the sum of
all even elements in the bag.
(4) Re-implement the static sumEven method, in the IntArrayBag class that takes one
input parameter of type IntArrayBag. The method then calculates and returns the sum of
all even elements in the bag.](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F38483929-e474-4cf5-b387-34c783578bc2%2F0fdfbbc8-3517-4693-a8e0-557c95e796f2%2Faq19dg_processed.jpeg&w=3840&q=75)
Transcribed Image Text:100% +
Consider the IntArrayBag data structure that is represented by the following UML.
IntArrayBag
-data:int[]
manyltems:int
+IntArrayBag()
+IntArrayBag(capacity:int)
+add(element:int) void
+countOcuurances(target:int):int
+grab(index:i):int
+remove(target:int):void
+size():int
+max():int
+countEven():int
+change(oldVal:int, newVal:int):int
(1) Implement an instance method, called bagMax, in the IntArrayBag class that finds and
return the maximum value in the bag.
(2) Implement an instance method, called equalsTo, in the IntArrayBag's class that takes
one input parameter and returns a boolean value. The input parameter, called otherBag,
is of type IntArrayBag as well. The method returns true if otherBag and the bag that
activates the method have exactly the same number of every element. Otherwise the method
return false. Note that the locations of the elements in the data arrays are not necessarily
the same, it is only the number of occurrences of each element that must be the same.
(3) Implement a static method, called sumEven, in the IntArrayBag class that takes one
input parameter of type IntArrayBag. The method then calculates and returns the sum of
all even elements in the bag.
(4) Re-implement the static sumEven method, in the IntArrayBag class that takes one
input parameter of type IntArrayBag. The method then calculates and returns the sum of
all even elements in the bag.
![2 / 2
100% +| E O
Consider the IntArraySequence data structure that is represented by the following UML.
IntArraySequence
-data:int[]
-manyltems:int
-currentIndex:int
+start():void
+advance():void
+getCurrent():int
+addAfter(element:int):void
+addBefore(element:int):void
+removeCurrent():void
+size():int
(1) Implement an instance method, called reverse, in the IntArraySequence class that
returns another sequence as output where the output sequence has the same elements
as the input sequence but in reverse order. For example, if the input sequence contains
the numbers {10,20,30,40}, then output sequence will contain (40,30,20,10}. You can use
any of the methods listed in the above UML.
(2) Assume that two sequences are considered equal is they contain the same values in the
same order. Implement a static method outside the IntArraySequence called
equalSeqeunces that takes two IntArraySequence parameters as input and
returns true or false based on whether the two input sequences are equal or no. You
can use any of the methods listed in the above UML.](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F38483929-e474-4cf5-b387-34c783578bc2%2F0fdfbbc8-3517-4693-a8e0-557c95e796f2%2Fvwf8ie_processed.jpeg&w=3840&q=75)
Transcribed Image Text:2 / 2
100% +| E O
Consider the IntArraySequence data structure that is represented by the following UML.
IntArraySequence
-data:int[]
-manyltems:int
-currentIndex:int
+start():void
+advance():void
+getCurrent():int
+addAfter(element:int):void
+addBefore(element:int):void
+removeCurrent():void
+size():int
(1) Implement an instance method, called reverse, in the IntArraySequence class that
returns another sequence as output where the output sequence has the same elements
as the input sequence but in reverse order. For example, if the input sequence contains
the numbers {10,20,30,40}, then output sequence will contain (40,30,20,10}. You can use
any of the methods listed in the above UML.
(2) Assume that two sequences are considered equal is they contain the same values in the
same order. Implement a static method outside the IntArraySequence called
equalSeqeunces that takes two IntArraySequence parameters as input and
returns true or false based on whether the two input sequences are equal or no. You
can use any of the methods listed in the above UML.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps

Recommended textbooks for you
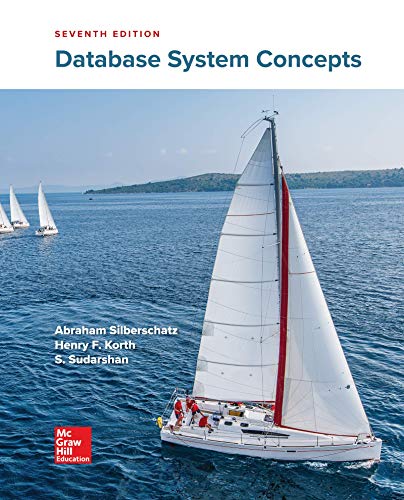
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
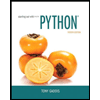
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
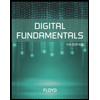
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
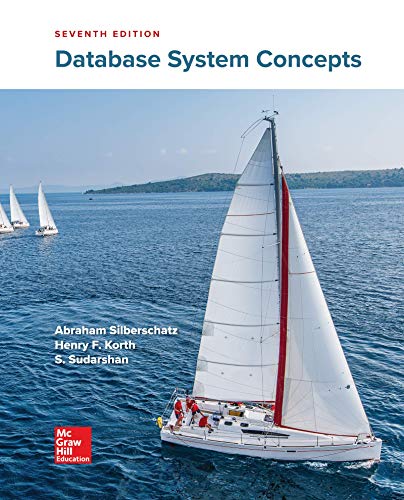
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
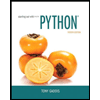
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
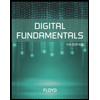
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
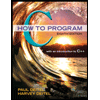
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
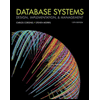
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
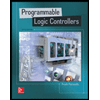
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education