Consider the instance variables and constructors. Given Instance Variables and Constructors: public class SimpleLinkedList implements SimpleList, Iterable, Serializable { // First Node of the List private Node first; // Last Node of the List private Node last; // Number of List Elements private int count; // Total Number of Modifications (Add and Remove Calls) private int modCount; /** * Creates an empty SimpleLinkedList. */ publicSimpleLinkedList(){ first = null; last = null; count = 0; modCount = 0; } ... Assume the class contains the following methods that work correctly: public boolean isEmpty() public int size() public boolean add(E e) public E get(int index) private void validateIndex(int index, int end) Complete the following methods based on the given information from above. /** * Adds an element to the list at the specified position. * * @param index the position where the element should be added. * @param e the element to be added to the list. * @return true if the list was changed * @throws IndexOutOfBoundsException if the index out of range, i.e. index < 0 or index >= the size(). */ public boolean add(int index, E e) { // TODO } /** * Returns the index of the first occurrence of a given element on the list equal * or -1 if the given element is not on the list. If the argument element is null, * the method returns the index of the first null element on the list, or -1 if the list * has no null elements. * * @param e the element to be located on the list. * @return the index of the first occurrence of a given element on the list equal or -1 * if the given element is not on the list. */ public int indexOf(E e) { // TODO } /** * Removes an element from the list at the specified position. * * @param index the position where the element should be removed. * @return the removed element * @throws IndexOutOfBoundsException if the index out of range, i.e. index < 0 or index >= the size(). */ public E remove(int index) { // TODO }
Consider the instance variables and constructors.
Given Instance Variables and Constructors:
public class SimpleLinkedList<E> implements SimpleList<E>, Iterable<E>, Serializable {
// First Node of the List
private Node<E> first;
// Last Node of the List
private Node<E> last;
// Number of List Elements
private int count;
// Total Number of Modifications (Add and Remove Calls)
private int modCount;
/**
* Creates an empty SimpleLinkedList.
*/
publicSimpleLinkedList(){
first = null;
last = null;
count = 0;
modCount = 0;
}
...
Assume the class contains the following methods that work correctly:
public boolean isEmpty()
public int size()
public boolean add(E e)
public E get(int index)
private void validateIndex(int index, int end)
Complete the following methods based on the given information from above.
/**
* Adds an element to the list at the specified position.
*
* @param index the position where the element should be added.
* @param e the element to be added to the list.
* @return true if the list was changed
* @throws IndexOutOfBoundsException if the index out of range, i.e. index < 0 or index >= the size().
*/
public boolean add(int index, E e) {
// TODO
}
/**
* Returns the index of the first occurrence of a given element on the list equal
* or -1 if the given element is not on the list. If the argument element is null,
* the method returns the index of the first null element on the list, or -1 if the list
* has no null elements.
*
* @param e the element to be located on the list.
* @return the index of the first occurrence of a given element on the list equal or -1
* if the given element is not on the list.
*/
public int indexOf(E e) {
// TODO
}
/**
* Removes an element from the list at the specified position.
*
* @param index the position where the element should be removed.
* @return the removed element
* @throws IndexOutOfBoundsException if the index out of range, i.e. index < 0 or index >= the size().
*/
public E remove(int index) {
// TODO
}

Trending now
This is a popular solution!
Step by step
Solved in 3 steps

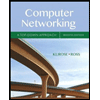
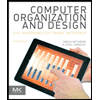
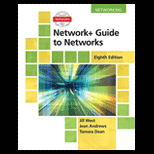
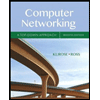
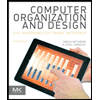
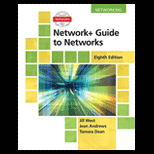
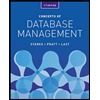
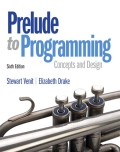
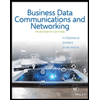