Compute the edit distance between “PLASMA” and “ALTRUISM”. A) Write down the 7 × 9 array of distances between all prefixes as computed by the following algorithm. int EditDistance(char s[1..m], char t[1..n]) { // for all i and j, d[i,j] will hold the edit distance between the first i characters of s and the first j characters of t; note that d has (m+1)x(n+1) values declare int d[0..m, 0..n] for i from 0 to m d[i, 0] := i // the distance of any first string to an empty second string for j from 0 to n d[0, j] := j // the distance of any second string to an empty first string for j from 1 to n { for i from 1 to m { if s[i] = t[j] then d[i, j] := d[i-1, j-1] // no operation required else d[i, j] := minimum ( d[i-1, j] + 1, // a deletion d[i, j-1] + 1, // an insertion d[i-1, j-1] + 1 // a substitution ) } } return d[m,n]
Compute the edit distance between “PLASMA” and “ALTRUISM”.
A) Write down the 7 × 9 array of distances between all prefixes as computed by the
following algorithm.
int EditDistance(char s[1..m], char t[1..n])
{
// for all i and j, d[i,j] will hold the edit distance between the first i characters of s and the first j
characters of t; note that d has (m+1)x(n+1) values
declare int d[0..m, 0..n]
for i from 0 to m
d[i, 0] := i // the distance of any first string to an empty second string
for j from 0 to n
d[0, j] := j // the distance of any second string to an empty first string
for j from 1 to n
{
for i from 1 to m
{
if s[i] = t[j] then
d[i, j] := d[i-1, j-1] // no operation required
else
d[i, j] := minimum
( d[i-1, j] + 1, // a deletion
d[i, j-1] + 1, // an insertion
d[i-1, j-1] + 1 // a substitution )
}
}
return d[m,n]

Trending now
This is a popular solution!
Step by step
Solved in 3 steps

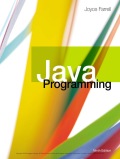
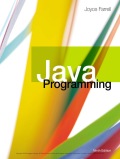