complete the //TODOs #include #include "swap.h" using namespace std; int main(int argc, char const *argv[]) { intfoo[5] = {1, 2, 3, 4, 5}; cout<<"Addresses of elements:"<>a; cout<<"Second index"<>b; cout<<"Enter 0 for pass-by-value, 1 for pass-by-pointer"<>f; switch (f) { case0: // Pass by Value // Compare the resultant array with the array you get after passing by pointer cout<<"Before swapping"<>flag; } cout<<"Reversing the array"; // Reverse your array // TODO complete the function in swap.cpp file reverse(foo,5); cout<<"\nAfter reversing"<,5); cout<<"\nAfter cumulative summation"<

swap.h
void swap_by_value(int x,int y);
void swap_by_pointers(int *x,int *y);
void reverse_array(int x[],int n);
void cumulative_sum(int x[],int n);
swap.cpp
#include <iostream>
#include "swap.h"
void swap_by_value(int x,int y)
{
int temp=x;
x=y;
y=temp;
}
void swap_by_pointers(int *x,int *y)
{
int temp=*x;
*x=*y;
*y=temp;
}
void reverse_array(int x[],int n)
{
int i=0,j=n-1;
while(i<j)
{
int temp=x[i];
x[i]=x[j];
x[j]=temp;
i++;
j--;
}
}
void cumulative_sum(int x[],int n)
{
int sum=x[0];
for(int i=1;i<n;i++)
{
sum=sum+x[i];
x[i]=sum;
}
}
main.cpp
#include<iostream>
#include "swap.h"
using namespace std;
int main(int argc, char const *argv[])
{
int foo[5] = {1, 2, 3, 4, 5};
cout<<"Addresses of elements:"<<endl;
for(int i=0;i<5;i++)
{
cout<<&foo[i]<<" ";
}
cout<<endl;
cout<<"Elements:"<<endl;;
int *ptr=foo;
for(int i=0;i<5;i++)
{
cout<<*ptr<<" ";
ptr=ptr+1;
}
cout<<endl;
int a,b;
int f;
int flag = 1;
while(flag == 1)
{
cout<<"Enter indices of elements you want to swap?"<<endl;
cout<<"First index"<<endl;
cin>>a;
cout<<"Second index"<<endl;
cin>>b;
cout<<"Enter 0 for pass-by-value, 1 for pass-by-pointer"<<endl;
cin>>f;
switch (f)
{
case 0:
// Pass by Value
// Compare the resultant array with the array you get after passing by pointer
cout<<"Before swapping"<<endl;
for(int i = 0;i<5;i++)
{
cout<<foo[i]<<" ";
}
cout<<endl;
swap_by_value(foo[a],foo[b]);
cout<<"\nAfter swapping"<<endl;
for(int i = 0;i<5;i++)
{
cout<<foo[i]<<" ";
}
cout<<endl;
break;
case 1:
// Pass by pointer
cout<<"Before swapping"<<endl;
for(int i = 0;i<5;i++)
{
cout<<foo[i]<<" ";
}
cout<<endl;
swap_by_pointers(&foo[a],&foo[b]);
cout<<"\nAfter swapping"<<endl;
for(int i = 0;i<5;i++)
{
cout<<foo[i]<<" ";
}
cout<<endl;
break;
}
cout<<endl;
cout<<"Press 1 to continue else press 0"<<endl;
cin>>flag;
}
cout<<"Reversing the array";
// Reverse your array
reverse_array(foo,5);
cout<<"\nAfter reversing"<<endl;
for(int i = 0;i<5;i++){
cout<<foo[i]<<" ";
}
cout<<endl;
// GOLD problem
// Cumulative sum of the array
// TODO call this function cumulative_sum properly so that it passes the array 'foo'
// and updates 'foo' with cumulative summation.
// After this function call, you will get the updated array here
// TODO complete the function declaration in swap.h file
// TODO complete the function in swap.cpp file
cumulative_sum(foo,5);
cout<<"\nAfter cumulative summation"<<endl;
for(int i = 0;i<5;i++){
cout<<foo[i]<<" ";
}
cout<<endl;
return 0;
}
Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

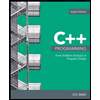
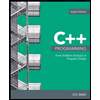