Column1 // Instance variable public class Monkey extends RescueAnimal { private String species; private int tailLength; private int height; private int bodyLength; // Constructor using super public Monkey(String name String tailLength super(); setName(name); setAnimalType("Monkey"); setGender(gender); setAge (age); } setWeight(weight); setAcquisitionDate(acquisitionDate); setAcquisitionLocation(acquisitionCountry); setTrainingStatus(trainingStatus); setReserved(reserved); setInServiceCountry(inService Country); this.species = species; this.tailLength = Integer.parseInt(tailLength); this.height = Integer.parseInt(height); this.bodyLength = Integer.parseInt(bodyLength); // Getters and setters for Monkey attributes public String getSpecies() { return species; } public void setSpecies(String species) { this.species species; } public int getTailLength() { return tailLength; } public void setTail Length (int tailLength) { this.tailLength = tailLength; } public int getHeight() { return height; } public void setHeight(int height) { this.height height; } public int getBody Length() [ return bodyLength; } } } public void setBodyLength(int bodyLength) [ this.bodyLength = bodyLength; Column2 Column3 Column4 Column5 Column6 Column7 Column8 Column9 Column10 Column11 String species String gender String age String weight String acquisitionDate String acquisitionCountry String trainingStatus boolean reserved String inService Country String height String bodyLength) { Column1 public class Driver { // Array list for Dogs private static ArrayList dogList = new ArrayList(); // Array list for Monkeys private static ArrayList monkeyList = new ArrayList(); public static void main(String[] args) { // Instance variables initializeDogList(); initialize MonkeyList(); // Loop that displays the menu // appropriate action. Scanner scanner = new Scanner(System.in); String choice; do { displayMenu(); choice = scanner.nextLine(); switch (choice) { case "1": intakeNewDog(scanner); break; case "2": intakeNewMonkey(scanner); break; case "3": reserveAnimal(scanner); break; case "4": printAnimals('4'); break; case "5": printAnimals('5'); break; case "6": printAnimals('6'); break; case "q": System.out.println("Exiting the program."); break; default: System.out.println("Invalid choice. Please try again."); } break; } } while (!choice.equalsIgnoreCase("q")); scanner.close(); // This method checks if the menu choice is valid (input validation) public static boolean isValid MenuChoice(String choice) { return choice.equals("1") || choice.equals("2") || choice.equals("3") || choice.equals("4") || choice.equals("5") || choice.equals("6") || choice.equalsIgnoreCase("q"); } // This method prints the menu options public static void displayMenu() { System.out.println("\n\n"); System.out.println("\t\t\t\tRescue Animal System Menu"); System.out.println("[1] Intake a new dog"); System.out.println("[2] Intake a new monkey"); System.out.println("[3] Reserve an animal"); System.out.println("[4] Print a list of all dogs"); System.out.println("[5] Print a list of all monkeys"); System.out.println("[6] Print a list of all animals that are not reserved"); System.out.println("[q] Quit application"); System.out.println(); System.out.println("Enter a menu selection"); } // Adds dogs to a list for testing public static void initialize DogList() { Dog dog1 = new Dog("Spot" false Dog dog2 = new Dog("Rex" "United States"); Dog dog3 = new Dog("Bella" "Canada"); dogList.add(dog1); dogList.add(dog2); dogList.add(dog3); // Adds monkeys to a list for testing public static void initialize MonkeyList() { // Add monkeys to the list Monkey monkey1 = new Monkey("Max" "intake" Monkey monkey2 = new Monkey("Ross" false Monkey monkey3 = new Monkey("Bella" true Monkey monkey4 = new Monkey("Marcelle" "intake" Monkey monkey5 = new Monkey("Rex" "Phase I" Monkey monkey6 = new Monkey("Andie" true } monkeyList.add(monkey1); monkeyList.add(monkey2); monkeyList.add(monkey3); monkeyList.add(monkey4); monkeyList.add(monkey5); monkeyList.add(monkey6); // IntakeNewDog method with input validation public static void intakeNewDog(Scanner scanner) { System.out.println("What is the dog's name?"); String name = scanner.nextLine(); for (Dog dog dogList) { if (dog.getName().equalsIgnoreCase(name}} { System.out.println("\n\nThis dog is already in our system\n\n"); return; // returns to menu } } } // Instantiate a new dog and add it to the appropriate list System.out.print("Enter the dog's breed: "); String breed = scanner.nextLine(); System.out.print("Enter the dog's gender: "); String gender = scanner.nextLine(); System.out.print("Enter the dog's age: "); int age = Integer.parseInt(scanner.nextLine()); System.out.print("Enter the dog's weight: "); double weight = Double.parseDouble(scanner.nextLine()); System.out.print("Enter the date the dog was acquired (MM-DD-YYYY):"); String acquisitionDate = scanner.nextLine(); System.out.print("Enter the country where the dog was acquired: "); String acquisitionCountry = scanner.nextLine(); System.out.print("Enter the dog's training status: "); String trainingStatus = scanner.nextLine(); System.out.print("Is this dog reserved? (true/false): "); boolean reserved = Boolean.parseBoolean[scanner.nextLine()); System.out.print("Enter the country where the dog is in service: "); String inService Country = scanner.nextLine(); // Create a new Dog object Dog newDog = new Dog(name reserved // Add the new dog to the dogList dogList.add(newDog); System.out.println("New dog added successfully!"); // IntakeNewMonkey with input validation public static void intakeNewMonkey(Scanner scanner) { System.out.println("What is the monkey's name?"); String name = scanner.nextLine(); for (Monkey monkey : monkeyList) { if (monkey.getName().equalsIgnoreCase(name)) { System.out.println("\nThis monkey is already in our system.\n"); return; // Return to the menu } } String species = ""; boolean validSpecies = false; while (!validSpecies) { System.out.println( "Enter monkey species (Capuchin species = scanner.nextLine(); // Perform input validation for species type if (species.equalsIgnoreCase("Capuchin") || species.equalsIgnoreCase("Guenon") || species.equalsIgnoreCase("Macaque") || species.equalsIgnoreCase("Marmaset") || species.equalsIgnoreCase("Squirrel monkey") || species.equalsIgnoreCase("Tamarin") || species.equalsIgnoreCase("other valid species")) { validSpecies = true; } else { System.out.println("Invalid species type. Please enter a valid species."); } } System.out.print("Enter the monkey's gender: "); String gender = scanner.nextLine(); System.out.print("Enter the monkey's age: "); int age = Integer.parseInt(scanner.nextLine()); System.out.print("Enter the monkey's weight: "); double weight = Double.parseDouble(scanner.nextLine()); System.out.print("Enter the date the monkey was acquired (MM-DD-YYYY): "); String acquisitionDate = scanner.nextLine(); System.out.print("Enter the country where the monkey was acquired: "); String acquisitionCountry = scanner.nextLine(); System.out.print("Enter the monkey's training status: "); String trainingStatus = scanner.nextLine(); System.out.print("Is this monkey reserved? (true/false): "); boolean reserved = Boolean.parseBoolean(scanner.nextLine()); System.out.print("Enter the country where the monkey is in service: "); String inServiceCountry = scanner.nextLine(); // Create a new Monkey object Monkey newMonkey = new Monkey(name trainingStatus // Add the new monkey to the monkeyList monkeyList.add(newMonkey); System.out.println("New monkey added successfully!"); // ReserveAnimal public static void reserve Animal(Scanner scanner) { // Prompt user for animal type and in service country System.out.println("What is the animal type? Dog or Monkey?"); String animalType = scanner.nextLine(); System.out.println("What is the animal's in service country?"); String inServiceCountry = scanner.nextLine(); // Check for Monkey availability and reserve first available boolean reservation = false; if (animalType.equalsIgnoreCase("Monkey")) { for (Monkey monkey: monkeyList) { f (monkey.getInServiceLocation().equalsIgnoreCase(inServiceCountry) && monkey.getReser && monkey.getTrainingStatus() == "in service") { monkey.setReserved(true); System.out.println("You have reserved" + monkey.toString()); reservation = true; return; } } if (reservation = false) { System.out.println("No Monkey is available to reserve at this service location."); } } // Check for Dog availability and reserve first available if (animalType.equalsIgnoreCase("Dog"}} { for (Dog dog dogList) { if (dog.getInServiceLocation().equalsIgnoreCase(inServiceCountry) && dog.getReserved() == && dog.getTrainingStatus() == "in service") { dog.setReserved(true); System.out.println("You have reserved" + dog.toString()); reservation = true; return; } } if (reservation == false) { System.out.println("No Dog is available to reserve at this service location."); } } else { System.out.println("Invalid Animal Type. Please reselect from main menu."); } } public static void printAnimals(char menuChoice) { // Prints list of all dogs if (menuChoice = '4') { for (int i = 0; i < dogList.size(); i++) { System.out.println(dogList.get(i).toString()); } } // Prints list of all monkeys else if (menuChoice == '5') { for (int i = 0; i < monkeyList.size(); i++) { System.out.println(monkeyList.get(i).toString()); } } // Prints a list of all animals that are in service and not reserved else if (menuChoice == '6') { for (int i = 0; i < dogList.size(); i++) { // Iterates through dog list to find dogs who are in service and available if (dogList.get(i).getTrainingStatus().equals("in service") && dogList.get(i).getReserved() == fa // Prints dogs that are in service and available System.out.println(dogList.get(i).toString()); } } // Iterates through monkey list to find monkeys who are in service and available for (int i = 0; i < monkeyList.size(); i++) { if (monkeyList.get(i).getTrainingStatus().equalsIgnoreCase("in service") && monkeyList.get(i).getReserved() == false) { // Prints monkeys that are in service and available System.out.println("The method printAnimals needs to be implemented"); } } } } Column2 Column 3 Column4 Column5 Column6 Column7 Column8 Column9 Column10 accepts the users input and takes the "German Shepherd" "male" "1" "25.6" "05-12-2019" "United States" "intake" "United States"); "Great Dane" "Chihuahua" "male" "3" "35.2" "02-03-2020" "United States" "Phase I" FALSE "female" "4" "25.6" "12-12-2019" "Canada" "in service" TRUE "Capuchin" "male" "2" "24.2" "20.2" "05-05-2021" "United States" false "Guenon" "United States"); "male" יידיי "20.2" "02-03-2020" "United States" "Phase I" "United States"); "Macaque" "female" "4" "20.3" "12-12-2019" "Canada" "in service" "Canada"); "Marmaset" "male" "1" "25.6" "05-12-2019" "United States" false "Squirrel monkey" false "United States"); "male" "United States"); "3" "25.2" "02-03-2020" "United States" "Tamarin" "female" "4" "25.6" "12-12-2019" "Canada" "in service" "Canada"); breed gender age weight acquisitionDate acquisitionCountry trainingStatus inServiceCountry); Guenon Macaque Marmaset Squirrel monkey Tamarin or another valid species): "); species gender age weight acquisitionDate acquisitionCountry reserved inServiceCountry);
I don't know what to do. I keep gettnig errors. I can't seem to get these two files to work correctly. Every time I think I fix an error, more errors pop up.
Exception in thread "main" java.lang.Error: Unresolved compilation problems:
Type mismatch: cannot convert from int to String
The field RescueAnimal.name is not visible
The field RescueAnimal.gender is not visible
The field RescueAnimal.age is not visible
The field RescueAnimal.weight is not visible
The field RescueAnimal.acquisitionDate is not visible
The field RescueAnimal.acquisitionCountry is not visible
The field RescueAnimal.trainingStatus is not visible
The field RescueAnimal.reserved is not visible
The field RescueAnimal.inServiceCountry is not visible
at grazioso.Monkey.<init>(Monkey.java:40)
at grazioso.Driver.initializeMonkeyList(Driver.java:90)
at grazioso.Driver.main(Driver.java:26)
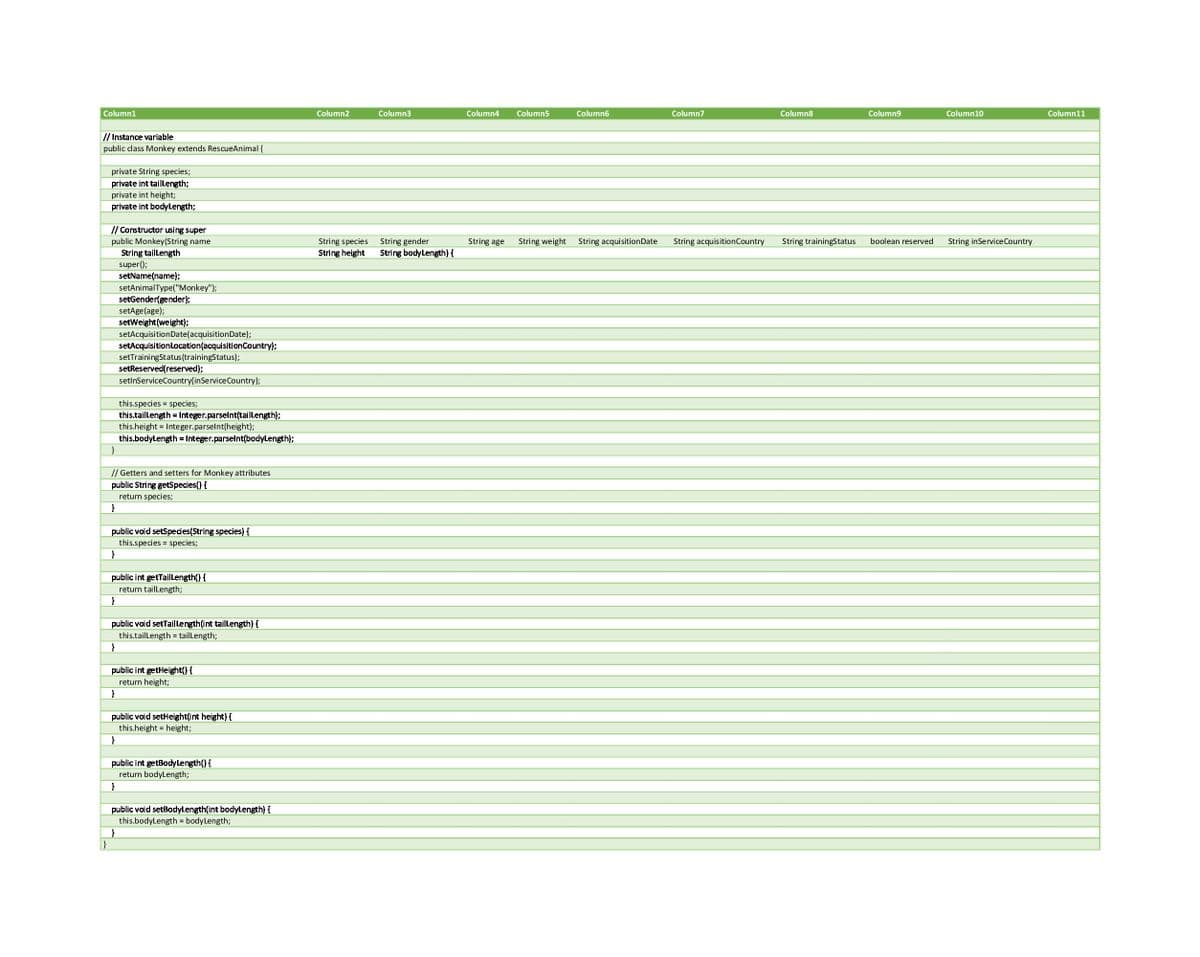
![Column1
public class Driver {
// Array list for Dogs
private static ArrayList<Dog> dogList = new ArrayList<Dog>();
// Array list for Monkeys
private static ArrayList<Monkey> monkeyList = new ArrayList<Monkey>();
public static void main(String[] args) {
// Instance variables
initializeDogList();
initialize MonkeyList();
// Loop that displays the menu
// appropriate action.
Scanner scanner = new Scanner(System.in);
String choice;
do {
displayMenu();
choice = scanner.nextLine();
switch (choice) {
case "1":
intakeNewDog(scanner);
break;
case "2":
intakeNewMonkey(scanner);
break;
case "3":
reserveAnimal(scanner);
break;
case "4":
printAnimals('4');
break;
case "5":
printAnimals('5');
break;
case "6":
printAnimals('6');
break;
case "q":
System.out.println("Exiting the program.");
break;
default:
System.out.println("Invalid choice. Please try again.");
}
break;
}
} while (!choice.equalsIgnoreCase("q"));
scanner.close();
// This method checks if the menu choice is valid (input validation)
public static boolean isValid MenuChoice(String choice) {
return choice.equals("1") || choice.equals("2") || choice.equals("3") || choice.equals("4")
|| choice.equals("5") || choice.equals("6") || choice.equalsIgnoreCase("q");
}
// This method prints the menu options
public static void displayMenu() {
System.out.println("\n\n");
System.out.println("\t\t\t\tRescue Animal System Menu");
System.out.println("[1] Intake a new dog");
System.out.println("[2] Intake a new monkey");
System.out.println("[3] Reserve an animal");
System.out.println("[4] Print a list of all dogs");
System.out.println("[5] Print a list of all monkeys");
System.out.println("[6] Print a list of all animals that are not reserved");
System.out.println("[q] Quit application");
System.out.println();
System.out.println("Enter a menu selection");
}
// Adds dogs to a list for testing
public static void initialize DogList() {
Dog dog1 = new Dog("Spot"
false
Dog dog2 = new Dog("Rex"
"United States");
Dog dog3 = new Dog("Bella"
"Canada");
dogList.add(dog1);
dogList.add(dog2);
dogList.add(dog3);
// Adds monkeys to a list for testing
public static void initialize MonkeyList() {
// Add monkeys to the list
Monkey monkey1 = new Monkey("Max"
"intake"
Monkey monkey2 = new Monkey("Ross"
false
Monkey monkey3 = new Monkey("Bella"
true
Monkey monkey4 = new Monkey("Marcelle"
"intake"
Monkey monkey5 = new Monkey("Rex"
"Phase I"
Monkey monkey6 = new Monkey("Andie"
true
}
monkeyList.add(monkey1);
monkeyList.add(monkey2);
monkeyList.add(monkey3);
monkeyList.add(monkey4);
monkeyList.add(monkey5);
monkeyList.add(monkey6);
// IntakeNewDog method with input validation
public static void intakeNewDog(Scanner scanner) {
System.out.println("What is the dog's name?");
String name = scanner.nextLine();
for (Dog dog dogList) {
if (dog.getName().equalsIgnoreCase(name}} {
System.out.println("\n\nThis dog is already in our system\n\n");
return; // returns to menu
}
}
}
// Instantiate a new dog and add it to the appropriate list
System.out.print("Enter the dog's breed: ");
String breed = scanner.nextLine();
System.out.print("Enter the dog's gender: ");
String gender = scanner.nextLine();
System.out.print("Enter the dog's age: ");
int age = Integer.parseInt(scanner.nextLine());
System.out.print("Enter the dog's weight: ");
double weight = Double.parseDouble(scanner.nextLine());
System.out.print("Enter the date the dog was acquired (MM-DD-YYYY):");
String acquisitionDate = scanner.nextLine();
System.out.print("Enter the country where the dog was acquired: ");
String acquisitionCountry = scanner.nextLine();
System.out.print("Enter the dog's training status: ");
String trainingStatus = scanner.nextLine();
System.out.print("Is this dog reserved? (true/false): ");
boolean reserved = Boolean.parseBoolean[scanner.nextLine());
System.out.print("Enter the country where the dog is in service: ");
String inService Country = scanner.nextLine();
// Create a new Dog object
Dog newDog = new Dog(name
reserved
// Add the new dog to the dogList
dogList.add(newDog);
System.out.println("New dog added successfully!");
// IntakeNewMonkey with input validation
public static void intakeNewMonkey(Scanner scanner) {
System.out.println("What is the monkey's name?");
String name = scanner.nextLine();
for (Monkey monkey : monkeyList) {
if (monkey.getName().equalsIgnoreCase(name)) {
System.out.println("\nThis monkey is already in our system.\n");
return; // Return to the menu
}
}
String species = "";
boolean validSpecies = false;
while (!validSpecies) {
System.out.println(
"Enter monkey species (Capuchin
species = scanner.nextLine();
// Perform input validation for species type
if (species.equalsIgnoreCase("Capuchin") || species.equalsIgnoreCase("Guenon")
|| species.equalsIgnoreCase("Macaque") || species.equalsIgnoreCase("Marmaset")
|| species.equalsIgnoreCase("Squirrel monkey") || species.equalsIgnoreCase("Tamarin")
|| species.equalsIgnoreCase("other valid species")) {
validSpecies = true;
} else {
System.out.println("Invalid species type. Please enter a valid species.");
}
}
System.out.print("Enter the monkey's gender: ");
String gender = scanner.nextLine();
System.out.print("Enter the monkey's age: ");
int age = Integer.parseInt(scanner.nextLine());
System.out.print("Enter the monkey's weight: ");
double weight = Double.parseDouble(scanner.nextLine());
System.out.print("Enter the date the monkey was acquired (MM-DD-YYYY): ");
String acquisitionDate = scanner.nextLine();
System.out.print("Enter the country where the monkey was acquired: ");
String acquisitionCountry = scanner.nextLine();
System.out.print("Enter the monkey's training status: ");
String trainingStatus = scanner.nextLine();
System.out.print("Is this monkey reserved? (true/false): ");
boolean reserved = Boolean.parseBoolean(scanner.nextLine());
System.out.print("Enter the country where the monkey is in service: ");
String inServiceCountry = scanner.nextLine();
// Create a new Monkey object
Monkey newMonkey = new Monkey(name
trainingStatus
// Add the new monkey to the monkeyList
monkeyList.add(newMonkey);
System.out.println("New monkey added successfully!");
// ReserveAnimal
public static void reserve Animal(Scanner scanner) {
// Prompt user for animal type and in service country
System.out.println("What is the animal type? Dog or Monkey?");
String animalType = scanner.nextLine();
System.out.println("What is the animal's in service country?");
String inServiceCountry = scanner.nextLine();
// Check for Monkey availability and reserve first available
boolean reservation = false;
if (animalType.equalsIgnoreCase("Monkey")) {
for (Monkey monkey: monkeyList) {
f (monkey.getInServiceLocation().equalsIgnoreCase(inServiceCountry) && monkey.getReser
&& monkey.getTrainingStatus() == "in service") {
monkey.setReserved(true);
System.out.println("You have reserved" + monkey.toString());
reservation = true;
return;
}
}
if (reservation = false) {
System.out.println("No Monkey is available to reserve at this service location.");
}
}
// Check for Dog availability and reserve first available
if (animalType.equalsIgnoreCase("Dog"}} {
for (Dog dog dogList) {
if (dog.getInServiceLocation().equalsIgnoreCase(inServiceCountry) && dog.getReserved() ==
&& dog.getTrainingStatus() == "in service") {
dog.setReserved(true);
System.out.println("You have reserved" + dog.toString());
reservation = true;
return;
}
}
if (reservation == false) {
System.out.println("No Dog is available to reserve at this service location.");
}
} else {
System.out.println("Invalid Animal Type. Please reselect from main menu.");
}
}
public static void printAnimals(char menuChoice) {
// Prints list of all dogs
if (menuChoice = '4') {
for (int i = 0; i < dogList.size(); i++) {
System.out.println(dogList.get(i).toString());
}
}
// Prints list of all monkeys
else if (menuChoice == '5') {
for (int i = 0; i < monkeyList.size(); i++) {
System.out.println(monkeyList.get(i).toString());
}
}
// Prints a list of all animals that are in service and not reserved
else if (menuChoice == '6') {
for (int i = 0; i < dogList.size(); i++) {
// Iterates through dog list to find dogs who are in service and available
if (dogList.get(i).getTrainingStatus().equals("in service") && dogList.get(i).getReserved() == fa
// Prints dogs that are in service and available
System.out.println(dogList.get(i).toString());
}
}
// Iterates through monkey list to find monkeys who are in service and available
for (int i = 0; i < monkeyList.size(); i++) {
if (monkeyList.get(i).getTrainingStatus().equalsIgnoreCase("in service")
&& monkeyList.get(i).getReserved() == false) {
// Prints monkeys that are in service and available
System.out.println("The method printAnimals needs to be implemented");
}
}
}
}
Column2
Column 3
Column4
Column5
Column6
Column7
Column8
Column9
Column10
accepts the users input and takes the
"German Shepherd"
"male"
"1"
"25.6"
"05-12-2019"
"United States"
"intake"
"United States");
"Great Dane"
"Chihuahua"
"male"
"3"
"35.2"
"02-03-2020"
"United States"
"Phase I"
FALSE
"female"
"4"
"25.6"
"12-12-2019"
"Canada"
"in service"
TRUE
"Capuchin"
"male"
"2"
"24.2"
"20.2"
"05-05-2021"
"United States"
false
"Guenon"
"United States");
"male"
יידיי
"20.2"
"02-03-2020"
"United States"
"Phase I"
"United States");
"Macaque"
"female"
"4"
"20.3"
"12-12-2019"
"Canada"
"in service"
"Canada");
"Marmaset"
"male"
"1"
"25.6"
"05-12-2019"
"United States"
false
"Squirrel monkey"
false
"United States");
"male"
"United States");
"3"
"25.2"
"02-03-2020"
"United States"
"Tamarin"
"female"
"4"
"25.6"
"12-12-2019"
"Canada"
"in service"
"Canada");
breed
gender
age
weight
acquisitionDate acquisitionCountry
trainingStatus
inServiceCountry);
Guenon
Macaque
Marmaset
Squirrel monkey
Tamarin
or another valid species): ");
species
gender
age
weight
acquisitionDate
acquisitionCountry
reserved
inServiceCountry);](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F1efed28f-8127-489a-8d3a-914c82b00fa5%2Fc8e34101-78dc-4e6f-b64f-15c27c1aedf5%2Fqpeb3i6_processed.jpeg&w=3840&q=75)

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

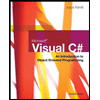
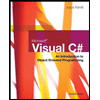