Coaster.java This class represents a roller coaster in the amusement park. It must be a subclass of Ride. Variables: double price the cost of one run double photoCost cost to purchase the mandatory photo package A passenger may ride the roller coaster multiple times and the cost must only be paid once. int maxNumRuns - the number of runs that the ride can complete before it must be inspected Constructors: a) A constructor that takes in name, lastInspection, passengers, price, photoCosts and maxNumRuns. b) A constructor that takes in name, lastInspection, and maxNumRuns. i) Defaults passengers to an empty String array of length 4, price to 10, and photoCost to 15. c) A constructor that takes in name. i) Methods: ii) iii) a) able ToRun i) ii) iii) iii) defaults lastInspection to 0, passengers to an empty String array of length 4, price to 10, photoCosts to 15, and maxNumRuns to 200. Remember that any mutable arguments should be deep copied. Use constructor chaining to minimize code repetition b) checkRide i) v) vi) vii) Takes in an int number of runs and returns a boolean representing whether the rollercoaster can complete that number of runs without needing an inspection. Make sure to factor in the number of times the roller coaster has run already. A ride cannot complete a negative number of runs. ii) Takes in a String[] that represents different components of the ride. Assume that the input array is not null and does not contain null elements. The array must contain "Tracks Clear" and "Brakes Ok" for the roller coaster to pass inspection. The input array may also contain other strings in any order. String comparisons should be case-insensitive. Returns true and resets lastInspection to 0 if the roller coaster passes inspection. Returns false if the roller coaster does not pass inspection. c) costPerPassenger i) Takes in an int number of stops and returns a double representing the cost for the passenger to ride the specified number of stops. Use the descriptions for the price and photoCost fields to determine the cost per passenger.
Coaster.java This class represents a roller coaster in the amusement park. It must be a subclass of Ride. Variables: double price the cost of one run double photoCost cost to purchase the mandatory photo package A passenger may ride the roller coaster multiple times and the cost must only be paid once. int maxNumRuns - the number of runs that the ride can complete before it must be inspected Constructors: a) A constructor that takes in name, lastInspection, passengers, price, photoCosts and maxNumRuns. b) A constructor that takes in name, lastInspection, and maxNumRuns. i) Defaults passengers to an empty String array of length 4, price to 10, and photoCost to 15. c) A constructor that takes in name. i) Methods: ii) iii) a) able ToRun i) ii) iii) iii) defaults lastInspection to 0, passengers to an empty String array of length 4, price to 10, photoCosts to 15, and maxNumRuns to 200. Remember that any mutable arguments should be deep copied. Use constructor chaining to minimize code repetition b) checkRide i) v) vi) vii) Takes in an int number of runs and returns a boolean representing whether the rollercoaster can complete that number of runs without needing an inspection. Make sure to factor in the number of times the roller coaster has run already. A ride cannot complete a negative number of runs. ii) Takes in a String[] that represents different components of the ride. Assume that the input array is not null and does not contain null elements. The array must contain "Tracks Clear" and "Brakes Ok" for the roller coaster to pass inspection. The input array may also contain other strings in any order. String comparisons should be case-insensitive. Returns true and resets lastInspection to 0 if the roller coaster passes inspection. Returns false if the roller coaster does not pass inspection. c) costPerPassenger i) Takes in an int number of stops and returns a double representing the cost for the passenger to ride the specified number of stops. Use the descriptions for the price and photoCost fields to determine the cost per passenger.
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
Given the following code and instructions:
Is the provided implemented correctly for said instructions?
The main class Ride contains:
public abstract boolean ableToRun(int numberofRuns);
public abstract boolean checkRide(String[] components);
public abstract double costPerPassenger(int numberOfStops);
public abstract boolean checkRide(String[] components);
public abstract double costPerPassenger(int numberOfStops);
![public class Coaster extends Ride {
private double price;
private double photoCosts;
private int maxNumRuns;
public Coaster (String name, int lastInspection, String[] passengers, double price, double photoCost, int maxNumRuns) {
super(name, lastInspection, passengers);
this.price = price;
this.photoCost = photoCost;
this.maxNumRuns = maxNumRuns;
}
public Coaster(String name, int lastInspection, int maxNumRuns) {
this (name, lastInspection, new String[4], 10.0, 15.0, maxNumRuns);
}
public Coaster(String name) {
this (name 0, new String[4], 10.0, 15.0, 200);
}
@Override
public boolean ableToRun(int numberOfRuns) {
return numberOfRuns >= 0 && (lastInspection + numberOfRuns) <= maxNumRuns;
}
@Override
public double costPerPassenger(int numberOfStops) {
return numberOfStops * (price + photoCost);
}
@Override
public boolean checkRide(String[] components) {
// Check if "Tracks Clear" and "Brakes Ok" are present
}
boolean tracksClear = false;
boolean brakes0k = false;
for (String component : components) {
if (component.equalsIgnoreCase("Tracks Clear")) {
tracksClear = true;
} else if (component.equalsIgnoreCase("Brakes Ok")) {
brakes0k = true;
}
}
// Return true if both conditions are met, reset runsSinceInspection to
if (tracksClear && brakes0k) {
runsSince Inspection = 0;
return true;
} else {
}
return false;](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F295c436f-1881-4143-81a2-89f6f40be87f%2Fdf7e9f98-6719-42ea-8617-2de64ab5aeff%2Fuibvpam_processed.png&w=3840&q=75)
Transcribed Image Text:public class Coaster extends Ride {
private double price;
private double photoCosts;
private int maxNumRuns;
public Coaster (String name, int lastInspection, String[] passengers, double price, double photoCost, int maxNumRuns) {
super(name, lastInspection, passengers);
this.price = price;
this.photoCost = photoCost;
this.maxNumRuns = maxNumRuns;
}
public Coaster(String name, int lastInspection, int maxNumRuns) {
this (name, lastInspection, new String[4], 10.0, 15.0, maxNumRuns);
}
public Coaster(String name) {
this (name 0, new String[4], 10.0, 15.0, 200);
}
@Override
public boolean ableToRun(int numberOfRuns) {
return numberOfRuns >= 0 && (lastInspection + numberOfRuns) <= maxNumRuns;
}
@Override
public double costPerPassenger(int numberOfStops) {
return numberOfStops * (price + photoCost);
}
@Override
public boolean checkRide(String[] components) {
// Check if "Tracks Clear" and "Brakes Ok" are present
}
boolean tracksClear = false;
boolean brakes0k = false;
for (String component : components) {
if (component.equalsIgnoreCase("Tracks Clear")) {
tracksClear = true;
} else if (component.equalsIgnoreCase("Brakes Ok")) {
brakes0k = true;
}
}
// Return true if both conditions are met, reset runsSinceInspection to
if (tracksClear && brakes0k) {
runsSince Inspection = 0;
return true;
} else {
}
return false;
![Coaster.java
This class represents a roller coaster in the amusement park. It must be a subclass of Ride.
Variables:
double price
the cost of one run
double photoCost
cost to purchase the mandatory photo package
A passenger may ride the roller coaster multiple times and the cost must only be paid once.
int maxNumRuns - the number of runs that the ride can complete before it must be inspected
Constructors:
a) A constructor that takes in name, lastInspection, passengers, price, photoCosts and maxNumRuns.
b) A constructor that takes in name, lastInspection, and maxNumRuns.
i) Defaults passengers to an empty String array of length 4, price to 10, and photoCost to 15.
c) A constructor that takes in name.
i)
Methods:
ii)
iii)
a) able ToRun
i)
ii)
iii)
defaults lastInspection to 0, passengers to an empty String array of length 4, price to 10,
photoCosts to 15, and maxNumRuns to 200.
Remember that any mutable arguments should be deep copied.
Use constructor chaining to minimize code repetition
b) checkRide
i)
ii)
iii)
iv)
v)
vi)
vii)
i)
Takes in an int number of runs and returns a boolean representing whether the rollercoaster can
complete that number of runs without needing an inspection.
Make sure to factor in the number of times the roller coaster has run already.
A ride cannot complete a negative number of runs.
ii)
Takes in a String[] that represents different components of the ride.
Assume that the input array is not null and does not contain null elements.
The array must contain "Tracks Clear" and "Brakes Ok" for the roller coaster to pass inspection.
The input array may also contain other strings in any order.
String comparisons should be case-insensitive.
c) costPerPassenger
Returns true and resets lastInspection to 0 if the roller coaster passes inspection.
Returns false if the roller coaster does not pass inspection.
Takes in an int number of stops and returns a double representing the cost for the passenger to ride
the specified number of stops.
Use the descriptions for the price and photoCost fields to determine the cost per passenger.](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F295c436f-1881-4143-81a2-89f6f40be87f%2Fdf7e9f98-6719-42ea-8617-2de64ab5aeff%2Fax0ce4l_processed.png&w=3840&q=75)
Transcribed Image Text:Coaster.java
This class represents a roller coaster in the amusement park. It must be a subclass of Ride.
Variables:
double price
the cost of one run
double photoCost
cost to purchase the mandatory photo package
A passenger may ride the roller coaster multiple times and the cost must only be paid once.
int maxNumRuns - the number of runs that the ride can complete before it must be inspected
Constructors:
a) A constructor that takes in name, lastInspection, passengers, price, photoCosts and maxNumRuns.
b) A constructor that takes in name, lastInspection, and maxNumRuns.
i) Defaults passengers to an empty String array of length 4, price to 10, and photoCost to 15.
c) A constructor that takes in name.
i)
Methods:
ii)
iii)
a) able ToRun
i)
ii)
iii)
defaults lastInspection to 0, passengers to an empty String array of length 4, price to 10,
photoCosts to 15, and maxNumRuns to 200.
Remember that any mutable arguments should be deep copied.
Use constructor chaining to minimize code repetition
b) checkRide
i)
ii)
iii)
iv)
v)
vi)
vii)
i)
Takes in an int number of runs and returns a boolean representing whether the rollercoaster can
complete that number of runs without needing an inspection.
Make sure to factor in the number of times the roller coaster has run already.
A ride cannot complete a negative number of runs.
ii)
Takes in a String[] that represents different components of the ride.
Assume that the input array is not null and does not contain null elements.
The array must contain "Tracks Clear" and "Brakes Ok" for the roller coaster to pass inspection.
The input array may also contain other strings in any order.
String comparisons should be case-insensitive.
c) costPerPassenger
Returns true and resets lastInspection to 0 if the roller coaster passes inspection.
Returns false if the roller coaster does not pass inspection.
Takes in an int number of stops and returns a double representing the cost for the passenger to ride
the specified number of stops.
Use the descriptions for the price and photoCost fields to determine the cost per passenger.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 4 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
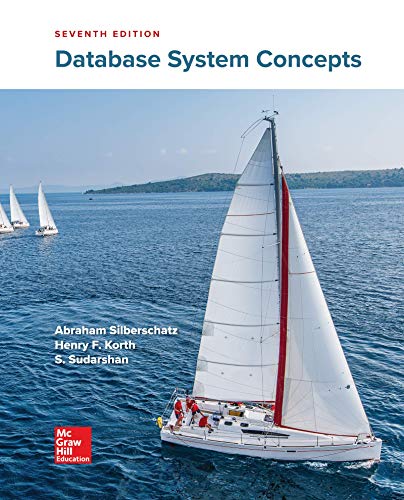
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
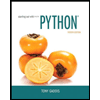
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
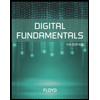
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
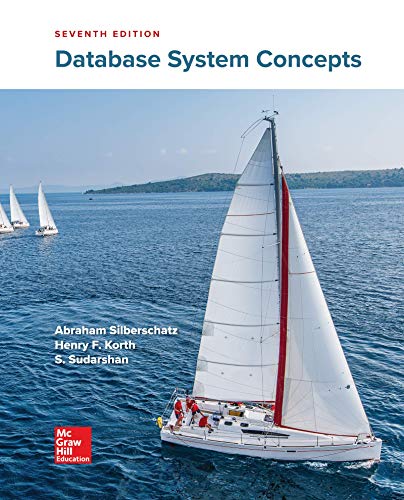
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
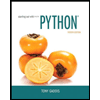
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
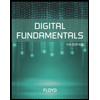
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
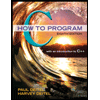
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
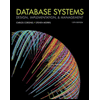
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
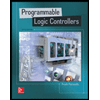
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education