Two strings, code1 and code2, are read from input as two states' codes. headObj has the default value of "code". Create a new node firstState with string code1 and insert firstState after headObj. Then, create a second node secondState with string code2 and insert secondState after firstState. Ex: If the input is MA IA, then the output is: code MA IA #include using namespace std; class StateNode { public: StateNode(string codeInit = "", StateNode* nextLoc = nullptr); void InsertAfter(StateNode* nodeLoc); StateNode* GetNext(); void PrintNodeData(); private: string codeVal; StateNode* nextNodePtr; }; StateNode::StateNode(string codeInit, StateNode* nextLoc) { this->codeVal = codeInit; this->nextNodePtr = nextLoc; } void StateNode::InsertAfter(StateNode* nodeLoc) { StateNode* tmpNext = nullptr; tmpNext = this->nextNodePtr; this->nextNodePtr = nodeLoc; nodeLoc->nextNodePtr = tmpNext; } StateNode* StateNode::GetNext() { return this->nextNodePtr; } void StateNode::PrintNodeData() { cout << this->codeVal << endl; } int main() { StateNode* headObj = nullptr; StateNode* firstState = nullptr; StateNode* secondState = nullptr; StateNode* currState = nullptr; string code1; string code2; cin >> code1; cin >> code2; headObj = new StateNode("code"); /* THE NEEDED CODE GOES HERE. THE REST OF THE CODE IS FINE. */ currState = headObj; while (currState != nullptr) { currState->PrintNodeData(); currState = currState->GetNext(); } return 0;
Types of Linked List
A sequence of data elements connected through links is called a linked list (LL). The elements of a linked list are nodes containing data and a reference to the next node in the list. In a linked list, the elements are stored in a non-contiguous manner and the linear order in maintained by means of a pointer associated with each node in the list which is used to point to the subsequent node in the list.
Linked List
When a set of items is organized sequentially, it is termed as list. Linked list is a list whose order is given by links from one item to the next. It contains a link to the structure containing the next item so we can say that it is a completely different way to represent a list. In linked list, each structure of the list is known as node and it consists of two fields (one for containing the item and other one is for containing the next item address).
C++ CODING PROBLEM WHERE I NEED CODE TO CREATE A LINKED LIST
(I also put a screen shot of the problem below.
Two strings, code1 and code2, are read from input as two states' codes.
headObj has the default value of "code".
Create a new node firstState with string code1 and insert firstState after headObj.
Then, create a second node secondState with string code2 and insert secondState after firstState.
Ex: If the input is MA IA, then the output is:
code
MA
IA
#include <iostream>
using namespace std;
class StateNode {
public:
StateNode(string codeInit = "", StateNode* nextLoc = nullptr);
void InsertAfter(StateNode* nodeLoc);
StateNode* GetNext();
void PrintNodeData();
private:
string codeVal;
StateNode* nextNodePtr;
};
StateNode::StateNode(string codeInit, StateNode* nextLoc) {
this->codeVal = codeInit;
this->nextNodePtr = nextLoc;
}
void StateNode::InsertAfter(StateNode* nodeLoc) {
StateNode* tmpNext = nullptr;
tmpNext = this->nextNodePtr;
this->nextNodePtr = nodeLoc;
nodeLoc->nextNodePtr = tmpNext;
}
StateNode* StateNode::GetNext() {
return this->nextNodePtr;
}
void StateNode::PrintNodeData() {
cout << this->codeVal << endl;
}
int main() {
StateNode* headObj = nullptr;
StateNode* firstState = nullptr;
StateNode* secondState = nullptr;
StateNode* currState = nullptr;
string code1;
string code2;
cin >> code1;
cin >> code2;
headObj = new StateNode("code");
/* THE NEEDED CODE GOES HERE. THE REST OF THE CODE IS FINE. */
currState = headObj;
while (currState != nullptr) {
currState->PrintNodeData();
currState = currState->GetNext();
}
return 0;


Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 4 images

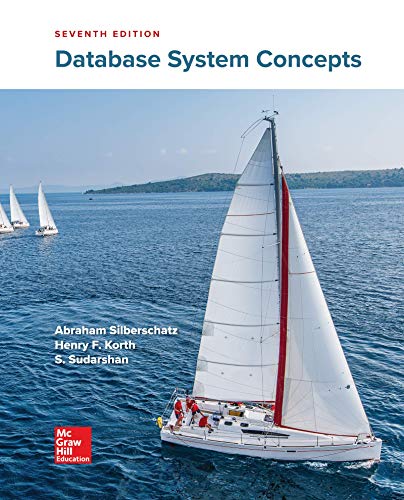
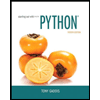
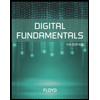
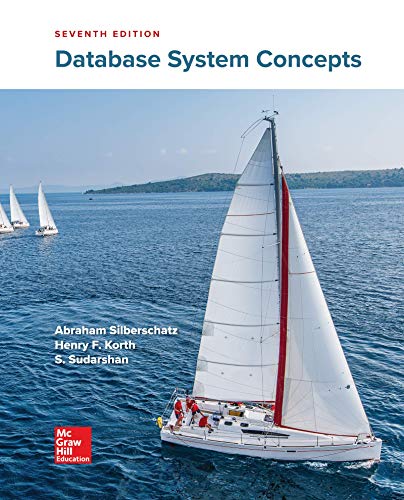
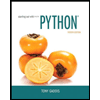
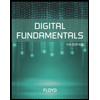
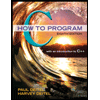
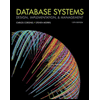
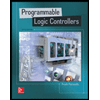