C++ code: Suppose you are creating a fantasy role-playing game. In this game we have four different types of Creatures: Humans, Cyberdemons, Balrogs, and elves. To represent one of these Creatures we might define a Creature class as follows: class Creature { private: int type; // 0 Human, 1 Cyberdemon, 2 Balrog, 3 elf int strength; // how much damage this Creature inflicts int hitpoints; // how much damage this Creature can sustain string getSpecies() const; // returns the type of the species public: Creature(); // initialize to Human, 10 strength, 10 hitpoints Creature(int newType, int newStrength, int newHitpoints); int getDamage() const; // returns the amount of damage this Creature // inflicts in one round of combat // also include appropriate accessors and mutators }; Here is an implementation of the getSpecies() function: string Creature::getSpecies() const { switch (type) { case 0: return "Human"; case 1: return "Cyberdemon"; case 2: return "Balrog"; case 3: return "Elf"; } return "unknown"; } The getDamage() function outputs and returns the damage this Creature can inflict in one round of combat. The rules for determining the damage are as follows: Every Creature inflicts damage that is a random number r, where 0 < r <= strength. Demons have a 25% chance of inflicting a demonic attack which is an additional 50 damage points. Balrogs and Cyberdemons are Demons. With a 50% chance elves inflict a magical attack that doubles the normal amount of damage. Balrogs are very fast, so they get to attack twice An implementation of getDamage() is given below: int Creature::getDamage() const { int damage; // All Creatures inflict damage which is a random number up to their strength damage = (rand() % strength) + 1; cout << getSpecies() << " attacks for " << damage << " points!" << endl; // Demons can inflict damage of 50 with a 25% chance if (type == 2 || type == 1){ if (rand() % 4 == 0) { damage = damage + 50; cout << "Demonic attack inflicts 50 additional damage points!" << endl; } } // Elves inflict double magical damage with a 50% chance if (type == 3) { if ((rand() % 2) == 0) { cout << "Magical attack inflicts " << damage << " additional damage points!" << endl; damage *= 2; } } // Balrogs are so fast they get to attack twice if (type == 2) { int damage2 = (rand() % strength) + 1; cout << "Balrog speed attack inflicts " << damage2 << " additional damage points!" << endl; damage += damage2; } return damage; } One problem with this implementation is that it is unwieldy to add new Creatures. Rewrite the class to use inheritance, which will eliminate the need for the variable "type". The Creature class should be the base class. The classes Demon, Elf, and Human should be derived from Creature. The classes Cyberdemon and Balrog should be derived from Demon. You will need to rewrite the getSpecies() and getDamage() functions so they are appropriate for each class. For example, the getDamage() function in each class should only compute the damage appropriate for that specific class. The total damage is then calculated by combining that damage with the results when getDamage() is called on the class's parent class. As an example, Balrog inherits from Demon, and Demon inherits from Creature. So invoking getDamage() for a Balrog object invokes getDamage() for a Demon object, which should invoke getDamage() for the Creature object. This will compute the basic damage that all Creatures inflict, followed by the random 25% damage that Demons inflict, followed by the double damage that Balrogs inflict. Also include mutator and accessor functions for the private variables.
C++ code: Suppose you are creating a fantasy role-playing game. In this game we have four different types of Creatures: Humans, Cyberdemons, Balrogs, and elves. To represent one of these Creatures we might define a Creature class as follows:
class Creature {
private:
int type; // 0 Human, 1 Cyberdemon, 2 Balrog, 3 elf
int strength; // how much damage this Creature inflicts
int hitpoints; // how much damage this Creature can sustain
string getSpecies() const; // returns the type of the species
public:
Creature(); // initialize to Human, 10 strength, 10 hitpoints
Creature(int newType, int newStrength, int newHitpoints);
int getDamage() const; // returns the amount of damage this Creature
// inflicts in one round of combat
// also include appropriate accessors and mutators
};
Here is an implementation of the getSpecies() function:
string Creature::getSpecies() const {
switch (type) {
case 0: return "Human";
case 1: return "Cyberdemon";
case 2: return "Balrog";
case 3: return "Elf";
}
return "unknown";
}
The getDamage() function outputs and returns the damage this Creature can inflict in one round of combat. The rules for determining the damage are as follows:
- Every Creature inflicts damage that is a random number r, where 0 < r <= strength.
- Demons have a 25% chance of inflicting a demonic attack which is an additional 50 damage points. Balrogs and Cyberdemons are Demons.
- With a 50% chance elves inflict a magical attack that doubles the normal amount of damage.
- Balrogs are very fast, so they get to attack twice
An implementation of getDamage() is given below:
int Creature::getDamage() const {
int damage;
// All Creatures inflict damage which is a random number up to their strength
damage = (rand() % strength) + 1;
cout << getSpecies() << " attacks for " << damage << " points!" << endl;
// Demons can inflict damage of 50 with a 25% chance
if (type == 2 || type == 1){
if (rand() % 4 == 0) {
damage = damage + 50;
cout << "Demonic attack inflicts 50 additional damage points!" << endl;
}
}
// Elves inflict double magical damage with a 50% chance
if (type == 3) {
if ((rand() % 2) == 0) {
cout << "Magical attack inflicts " << damage << " additional damage points!" << endl;
damage *= 2;
}
}
// Balrogs are so fast they get to attack twice
if (type == 2) {
int damage2 = (rand() % strength) + 1;
cout << "Balrog speed attack inflicts " << damage2 << " additional damage points!" << endl;
damage += damage2;
}
return damage;
}
One problem with this implementation is that it is unwieldy to add new Creatures. Rewrite the class to use inheritance, which will eliminate the need for the variable "type". The Creature class should be the base class. The classes Demon, Elf, and Human should be derived from Creature. The classes Cyberdemon and Balrog should be derived from Demon. You will need to rewrite the getSpecies() and getDamage() functions so they are appropriate for each class.
For example, the getDamage() function in each class should only compute the damage appropriate for that specific class. The total damage is then calculated by combining that damage with the results when getDamage() is called on the class's parent class. As an example, Balrog inherits from Demon, and Demon inherits from Creature. So invoking getDamage() for a Balrog object invokes getDamage() for a Demon object, which should invoke getDamage() for the Creature object. This will compute the basic damage that all Creatures inflict, followed by the random 25% damage that Demons inflict, followed by the double damage that Balrogs inflict. Also include mutator and accessor functions for the private variables.

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

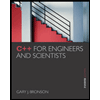
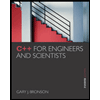