c++ code Also in code i am getting "sh: 1: PAUSE: not found" Write a program that reads in a line consisting of a student’s name, Social Security number, user ID, and password. The program outputs the string in which all the digits of the Social Security number and all the characters in the password are replaced by x. (The Social Security number is in the form 000-00-0000, and the user ID and the password do not contain any spaces.) Your program should not use the operator [] to access a string element. Use the appropriate functions described in Table 7-1 below. #include //include statement(s) #include #include using namespace std; //using namespace statement(s) void getInfo(string info); //void function header to get info int main() { string k; //variable declaration(s) cout << "Enter your Name, Social Security number, User ID, and Passord - separated\nby commas: " << endl; cout << endl; getline(cin, k); //reads the values the user inputs cout << endl; getInfo(k); //void function call to get info cout << endl; system ("PAUSE"); //black box appears return 0; //return statement(s) } void getInfo(string info) { int pos1 = info.find(",", 0); //finds the position(s) after each comma int pos2 = info.find(",", pos1 + 1); int pos3 = info.find(",", pos2 + 1); int lastPos = info.length() - 1; //finds the last position int nameLength = pos1 - 0; //finds the length for each position(s) int ssnLength = pos2 - (pos1 + 1); int idLength = pos3 - (pos2 + 1); int passLength = lastPos - (pos3 + 1) + 1; string passwordMask = info.substr(pos3 + 1, passLength); //passwordMask is a substring to convert the for (int i = 0; i < passLength; i++) //password characters into X's { passwordMask.erase(i, 1); passwordMask.insert(i, "X"); } string ssnMask = info.substr(pos1 + 1, ssnLength); //ssnMask is a substring to convert the social security for (int i = 0; i < ssnLength; i++) //numbers into X's, added condition if there are '-' { //to NOT make them X's if (ssnMask.at(i) != '-') { ssnMask.erase(i, 1); ssnMask.insert(i, "X"); } } cout << "Your name is: " << info.substr(0, nameLength) << endl; cout << "Social Security Number is: " << ssnMask << endl; cout << "Your ID is: " << info.substr(pos2 + 1, idLength) << endl; cout << "Your password is: " << passwordMask << endl; return; } CODE WORKS FINE, BUT THE OUTPUT NEEDS TO BE ONLY IN ONE LINE! Not as pictured in the attachment. Below is how the output should read, it does need to be like the attachment, just one line with the output below. John Doe xxxxxxxxx DoeJ xxxxxxxxxxx
Max Function
Statistical function is of many categories. One of them is a MAX function. The MAX function returns the largest value from the list of arguments passed to it. MAX function always ignores the empty cells when performing the calculation.
Power Function
A power function is a type of single-term function. Its definition states that it is a variable containing a base value raised to a constant value acting as an exponent. This variable may also have a coefficient. For instance, the area of a circle can be given as:
c++ code
Also in code i am getting "sh: 1: PAUSE: not found"
Write a program that reads in a line consisting of a student’s name, Social Security number, user ID, and password. The program outputs the string in which all the digits of the Social Security number and all the characters in the password are replaced by x. (The Social Security number is in the form 000-00-0000, and the user ID and the password do not contain any spaces.) Your program should not use the operator [] to access a string element. Use the appropriate functions described in Table 7-1 below.
#include <iostream> //include statement(s)
#include <iomanip>
#include <string>
using namespace std; //using namespace statement(s)
void getInfo(string info); //void function header to get info
int main()
{
string k; //variable declaration(s)
cout << "Enter your Name, Social Security number, User ID, and Passord - separated\nby commas: " << endl;
cout << endl;
getline(cin, k); //reads the values the user inputs
cout << endl;
getInfo(k); //void function call to get info
cout << endl;
system ("PAUSE"); //black box appears
return 0; //return statement(s)
}
void getInfo(string info)
{
int pos1 = info.find(",", 0); //finds the position(s) after each comma
int pos2 = info.find(",", pos1 + 1);
int pos3 = info.find(",", pos2 + 1);
int lastPos = info.length() - 1; //finds the last position
int nameLength = pos1 - 0; //finds the length for each position(s)
int ssnLength = pos2 - (pos1 + 1);
int idLength = pos3 - (pos2 + 1);
int passLength = lastPos - (pos3 + 1) + 1;
string passwordMask = info.substr(pos3 + 1, passLength); //passwordMask is a substring to convert the
for (int i = 0; i < passLength; i++) //password characters into X's
{
passwordMask.erase(i, 1);
passwordMask.insert(i, "X");
}
string ssnMask = info.substr(pos1 + 1, ssnLength); //ssnMask is a substring to convert the social security
for (int i = 0; i < ssnLength; i++) //numbers into X's, added condition if there are '-'
{ //to NOT make them X's
if (ssnMask.at(i) != '-')
{
ssnMask.erase(i, 1);
ssnMask.insert(i, "X");
}
}
cout << "Your name is: " << info.substr(0, nameLength) << endl;
cout << "Social Security Number is: " << ssnMask << endl;
cout << "Your ID is: " << info.substr(pos2 + 1, idLength) << endl;
cout << "Your password is: " << passwordMask << endl;
return;
}
CODE WORKS FINE, BUT THE OUTPUT NEEDS TO BE ONLY IN ONE LINE!
Not as pictured in the attachment.
Below is how the output should read, it does need to be like the attachment, just one line with the output below.
John Doe xxxxxxxxx DoeJ xxxxxxxxxxx
![Results
John Doe xхххххххх Doе] ххххххXхх
Expected Output ©
John Doe XXXXXXXXX DoeJ XXXXXXXXXXX
Run Checks
Submit 0%](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fd248ad0b-fe53-4ae5-b8b2-1d06d59be2e8%2F37717c11-8bc4-4547-96b0-a3320e6fb735%2Fgy06wv8_processed.png&w=3840&q=75)


Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

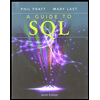
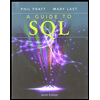
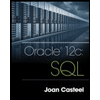
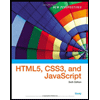