Bob the Porcupine wishes to buy some decorative beads for his quills. He heads to the bead shop where the owner, Arnold the Aardvark, Is struggling to write a Python program to compute the cost of a customer's bead order from some files that have the pertinent information. Arnold has a comma-separated file contalining the ist of beads he has (actually It's a list of codes which represent each Individual type of bead), along with the quantity-on-hand of each type of bead and the corresponding unit price (the price for a single bead of each ype). Amold also has the customer's bead order in another file and he's wondering how to quickly print up an Itemized recelpt for the customer and show them the total price. He needs to be able to repeat this process by running the program again with different Input files. Siven two Input files, with user-entered filenames (e.g., bead pricelist.txt and bead_order. txt), where each line of the bead pricellst file includes the bead code (e.g., "BLUPL" for a blue plastic bead), the quantity- on-hand (e.g., 500) and the unit price of the bead (eg., 0.15), and each line of the bead order file Includes the bead code for each type of bead ordered and the quantity of that type of bead ordered by the customer Help Amold write a Python program to compute and print the customer's recelpt, Including the bead code, the quantity ordered and the total price of that many of those types of beads. In addition, your program should also compute and print the total price of all beads ordered for that customer's order. Remember to use
Bob the Porcupine wishes to buy some decorative beads for his quills. He heads to the bead shop where the owner, Arnold the Aardvark, Is struggling to write a Python program to compute the cost of a customer's bead order from some files that have the pertinent information. Arnold has a comma-separated file contalining the ist of beads he has (actually It's a list of codes which represent each Individual type of bead), along with the quantity-on-hand of each type of bead and the corresponding unit price (the price for a single bead of each ype). Amold also has the customer's bead order in another file and he's wondering how to quickly print up an Itemized recelpt for the customer and show them the total price. He needs to be able to repeat this process by running the program again with different Input files. Siven two Input files, with user-entered filenames (e.g., bead pricelist.txt and bead_order. txt), where each line of the bead pricellst file includes the bead code (e.g., "BLUPL" for a blue plastic bead), the quantity- on-hand (e.g., 500) and the unit price of the bead (eg., 0.15), and each line of the bead order file Includes the bead code for each type of bead ordered and the quantity of that type of bead ordered by the customer Help Amold write a Python program to compute and print the customer's recelpt, Including the bead code, the quantity ordered and the total price of that many of those types of beads. In addition, your program should also compute and print the total price of all beads ordered for that customer's order. Remember to use
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question

Transcribed Image Text:Remember: The rstrip () or strip () methods can be used to get rid of unwanted newlines on the Input
lines from the Input fles, and "\n" can be appended to a line, when writing to a file, If you want to begin on a
new line.
Hint: The bead price list can be read Into a two-dimenslonal list to make looking up the price of the beads
easler while processing the order, and also to facilitate the updates of the quantitles. Once the order Is
processed, the two-dimensional list can be used to write Infomation to the new updated bead list file.

Transcribed Image Text:Write a Python program to solve the following problem:
PURM, 180, 0.59
PNKG, 153,0.39
Bob the Porcupine wishes to buy some decorative beads for his quills. He heads to the bead shop where the
owner, Arnold the Aardvark, Is struggling to write a Python program to compute the cost of a customer's bead
order from some files that have the pertinent Information. Arnold has a comma-separated file contalning the
ist of beads he has (actually It's a list of codes which represent each Individual type of bead), along with the
quantity-on-hand of each type of bead and the corresponding unit price (the price for a single bead of each
type). Amold also has the customer's bead order In another file and he's wondering how to qulckly print up
an Itemized recelpt for the customer and show them the total price. He needs to be able to repeat this
process by runnling the program again with different Input files.
CLRP, 206, 0.10
CLRM, 279,0.15
and the glven Input file bead_order.txt as follows:
GRNE, 30
GRNG, 50
PURM, 180
PNKG, 150
BLUP, 25
REDG, 100
CLRM, 60
Given two Input filles, with user-entered filenames (e.g. bead_pricelist.txt and bead_order.txt).
where
• each line of the bead pricelist file Includes the bead code (e.g. "BLUPL" for a blue plastic bead), the quantity-
on-hand (e.g., 50) and the unit price of the bead (e.g. 0.15), and
• each line of the bead order file Includes the bead code for each type of bead ordered and the quantity of
that type of bead ordered by the customer
BLUG, 200
The sample Input/output should look like:
Enter bead price list filename: bead_pricelist.txt
Enter bead order filename: bead_order.txt
Quant Ordered
30
Bead Code
Total Frice
Help Amold write a Python program to compute and print the customer's recelpt, Including the bead code, the
quantity ordered and the total price of that many of those types of beads. In addition, your program should
also compute and print the total price of all beads ordered for that customer's order. Remember to use
formatting and descriptive labels as shown in the sample output.
$27.00
$32.50
$106.20
$58.50
$12.25
$29.00
$9.00
GRNE
GRNG
50
PURM
180
PNKG
150
BLUP
25
REDG
100
Besides printing the required output, your program must also write the bead code, the new quantity-on-hand
(the number of beads remalning after the order has been filled), and the unit price of the bead, to a new
output file called result_bead_list.txt. For each type of bead, If the quantity on hand Is less than 5,
then the message "ORDER MORE" should be added to the end of the line In the file. NOTE: You may assume
that the customer's order will never result in a negative number of beads remalning, I.e., the order can always
be completely filled).
CLRM
60
BLUG
200
$70.00
Total Frice: $344.45
And the contents of the output file, zesult_bead_list.txt should be:
BLUF, 133, 0.49
REDG, 3,0.29 ORDER MORE
Include exception handling In your program so that an error Is produced and your program exits cleanly If any
files are not avallable to be opened.
YELG, 220,0.99
YELM, 109,0.89
GRNP, 287,0.90
Call your program file bead_purchase.py and test your program with the glven files,
bead_pricelist.ext and bead_order.txt, as shown below, and your own test data as well.
GRNG, 474,0.65
BLUG, 5,0.35
REDM, 325,0.25
PURM, 0,0.59 ORDER MORE
Note: The Input files should be placed In the same directory as the .py file for testing (or the pathname to the
Input files would need to be specified).
PNKG, 3,0.39 ORDER MORE
CLRE, 206, 0.10
CLRM, 219,0.15
For example, with the glven Input file bead_pricelist.txt as follows:
ELUF, 158,0.49
REDG, 103,0.29
YELG, 220,0.99
If the program trles to open a file that does not exist (e.g., bead_price.txt), then the following Input
prompt, Input and output should be generated:
YELM, 109, 0.89
GRNE, 317,0.90
Enter bead price list filename: bead_price.-txt
Enter bead order filename: bead_order.txt
GRNG, 524, 0. 65
Error: File not fouund.
ELUG, 205, 0.35
REDM, 325, 0.25
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 3 steps

Recommended textbooks for you
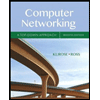
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
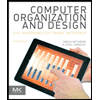
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
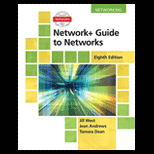
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
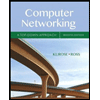
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
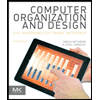
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
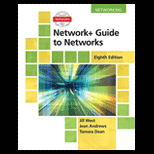
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
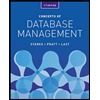
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
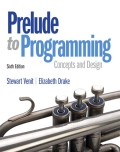
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
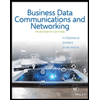
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY