Ask the user for an account balance. Show, in descending order, all the accounts that have a balance greater than what the user input. Each entry is int, string, long, double, boolean (name length, name, credit card number, balance, cashback).
Ask the user for an account balance. Show, in descending order, all the accounts that have a balance greater than what the user input. Each entry is int, string, long, double, boolean (name length, name, credit card number, balance, cashback).
Chapter6: Looping
Section: Chapter Questions
Problem 5GZ
Related questions
Question
Question 4: Ask the user for an account balance. Show, in descending order, all the accounts that have a balance greater than what the user input.
Each entry is int, string, long, double, boolean (name length, name, credit card number, balance, cashback).
File that was given
https://bes.hypergrade.com/api/user/file/1923815
NOTE: It has to be printed and sorted by Balance (Higher value on top)
===========================================
File one
---------------------
public class Person implements Comparable<Person> {
private long account;
private String name;
private double balance;
private boolean YN;
public Person(String name, long account, double balance, boolean YN) {
this.account = account;
this.balance = balance;
this.YN = YN;
this.name = name;
}
@Override
public int compareTo(Person per) {
int compare = name.compareTo(per.name);
if (compare == 0) {
return 0;
} else if (compare < 1) {
return -1;
} else {
return 1;
}
}
/**
* @return the balance
*/
public double getBalance() {
return balance;
}
@Override
public String toString() {
String yes_no;
String bs = String.valueOf(balance);
int b = bs.length();
if (!YN) {
yes_no = "No";
} else {
yes_no = "Yes";
}
if (b < 7) {
bs = b + "0";
}
return String.format("%20s", name) + String.format("%20s", account) + String.format("%10s", bs)
+ String.format("%10s", yes_no);
}
private long account;
private String name;
private double balance;
private boolean YN;
public Person(String name, long account, double balance, boolean YN) {
this.account = account;
this.balance = balance;
this.YN = YN;
this.name = name;
}
@Override
public int compareTo(Person per) {
int compare = name.compareTo(per.name);
if (compare == 0) {
return 0;
} else if (compare < 1) {
return -1;
} else {
return 1;
}
}
/**
* @return the balance
*/
public double getBalance() {
return balance;
}
@Override
public String toString() {
String yes_no;
String bs = String.valueOf(balance);
int b = bs.length();
if (!YN) {
yes_no = "No";
} else {
yes_no = "Yes";
}
if (b < 7) {
bs = b + "0";
}
return String.format("%20s", name) + String.format("%20s", account) + String.format("%10s", bs)
+ String.format("%10s", yes_no);
}
============================================
File 2
--------------------------------------------
import java.io.File;
import java.io.FileNotFoundException;
import java.io.FileWriter;
import java.io.IOException;
import java.util.ArrayList;
import java.util.Scanner;
public class PersonMain {
public static void main(String[] args) throws FileNotFoundException {
//Write
writedatFile();
//Create an arraylist to hold person objects
ArrayList<Person> personList = new ArrayList<Person>();
//Read file
File f = new File("accounts-with-names.dat");
Scanner sc = new Scanner(f);
//Loop until file has data
while (sc.hasNext()) {
//Read every line
String line = sc.nextLine();
//Split fields using comma
String[] lineArr = line.split(",");
int nameLength = Integer.parseInt(lineArr[0]);
String name = lineArr[1];
long account = Long.parseLong(lineArr[2]);
double balance = Double.parseDouble(lineArr[3]);
boolean YN = lineArr[4].equalsIgnoreCase("true");
personList.add(new Person(name, account, balance, YN));
}
sc = new Scanner(System.in);
System.out.println("Enter a balance");
double userEnteredBalance = sc.nextDouble();
System.out.println("Accounts with a balance of at least "+userEnteredBalance+" (sorted by balance)");
System.out.printf("%20s%20s%10s%10s\n", "Name", "Account Number", "Balance", "Cash Back");
int count = 0;
//Iterate and print details
for (Person p : personList) {
if (p.getBalance() >= userEnteredBalance) {
System.out.println(p.toString());
count++;
}
}
System.out.println(count +" results\n");
}
private static void writedatFile() {
try {
FileWriter fileStream = null;
fileStream = new FileWriter("accounts-with-names.dat");
} catch (IOException ex) {
ex.getMessage();
}
}
}
import java.io.FileNotFoundException;
import java.io.FileWriter;
import java.io.IOException;
import java.util.ArrayList;
import java.util.Scanner;
public class PersonMain {
public static void main(String[] args) throws FileNotFoundException {
//Write
writedatFile();
//Create an arraylist to hold person objects
ArrayList<Person> personList = new ArrayList<Person>();
//Read file
File f = new File("accounts-with-names.dat");
Scanner sc = new Scanner(f);
//Loop until file has data
while (sc.hasNext()) {
//Read every line
String line = sc.nextLine();
//Split fields using comma
String[] lineArr = line.split(",");
int nameLength = Integer.parseInt(lineArr[0]);
String name = lineArr[1];
long account = Long.parseLong(lineArr[2]);
double balance = Double.parseDouble(lineArr[3]);
boolean YN = lineArr[4].equalsIgnoreCase("true");
personList.add(new Person(name, account, balance, YN));
}
sc = new Scanner(System.in);
System.out.println("Enter a balance");
double userEnteredBalance = sc.nextDouble();
System.out.println("Accounts with a balance of at least "+userEnteredBalance+" (sorted by balance)");
System.out.printf("%20s%20s%10s%10s\n", "Name", "Account Number", "Balance", "Cash Back");
int count = 0;
//Iterate and print details
for (Person p : personList) {
if (p.getBalance() >= userEnteredBalance) {
System.out.println(p.toString());
count++;
}
}
System.out.println(count +" results\n");
}
private static void writedatFile() {
try {
FileWriter fileStream = null;
fileStream = new FileWriter("accounts-with-names.dat");
} catch (IOException ex) {
ex.getMessage();
}
}
}
=====================================================
The issue with my code is that it will not output the information needed.
NOTE: It has to be sorted by Balance (Higher value on top)
The CURRENT output is-
Accounts with a balance of at least 9000.0 (sorted by balance)
Name Account Number Balance Cash Back
0 results
Name Account Number Balance Cash Back
0 results

Transcribed Image Text:Test Case 1
Enter a balance \n
9000 ENTER
Accounts with a balance of at least $9000.00 (sorted by balance) \n
Name
Account Number Balance Cash Back \n
Brand Hallam
3573877643495486
9985.21
No \n
Paco Verty
4508271490627227
9890.51
No \n
Stanislaw Dhenin
4405942746261912
9869.27
No \n
Eachelle Balderstone
30526110612015
9866.30
No \n
Reube Worsnop
3551244602153760 9409.97
Yes In
5100172198301454 9315.15
No \n
Tiphanie Oland
Jordan Rylstone
201715141501700
9135.90
Yes In
7 results \n
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
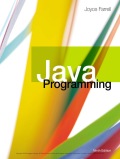
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
Np Ms Office 365/Excel 2016 I Ntermed
Computer Science
ISBN:
9781337508841
Author:
Carey
Publisher:
Cengage
COMPREHENSIVE MICROSOFT OFFICE 365 EXCE
Computer Science
ISBN:
9780357392676
Author:
FREUND, Steven
Publisher:
CENGAGE L
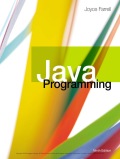
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
Np Ms Office 365/Excel 2016 I Ntermed
Computer Science
ISBN:
9781337508841
Author:
Carey
Publisher:
Cengage
COMPREHENSIVE MICROSOFT OFFICE 365 EXCE
Computer Science
ISBN:
9780357392676
Author:
FREUND, Steven
Publisher:
CENGAGE L