are to write the code for the addLongInt function. Other parts of the program should not be changed. You should model your solution on the string adder program that you wrote for mp-0. Your changes will result from the fact that the string adder program uses strings to represent arbitrarily large integers and this program uses doubly linked lists and here you will be doing addition base ten.. MUST write the program by supplying the missing code to the partial program supplied. The only allowed change is that you
This program implements integer addition with arbitrarily large integers.
Integers are implemented as doubly linked lists where each link represents one
digit. You are to write the code for the addLongInt function. Other parts of
the program should not be changed. You should model your solution on the
string adder program that you wrote for mp-0. Your changes will result from
the fact that the string adder program uses strings to represent arbitrarily
large integers and this program uses doubly linked lists and here you will be
doing addition base ten.. MUST write the program by supplying the missing
code to the partial program supplied. The only allowed change is that you
may remove using namespace std;, replace cin with std::cin and replace
cout with std::cout.
Test data:
-> 99999999999999999999
-> 1
-> 1
-> 99999999999999999999
-> 123456789123456789
-> 111111111111111111
-> 88888888888888888888
-> 22222222222222222222
-> 9000000000000000000000009
-> 9999999999999999999999999
-> 9999999999999999999999999
-> 9999999999999999999999999

Step by step
Solved in 4 steps with 5 images

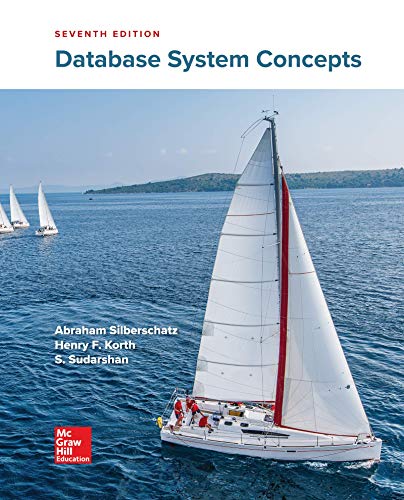
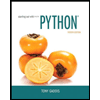
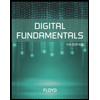
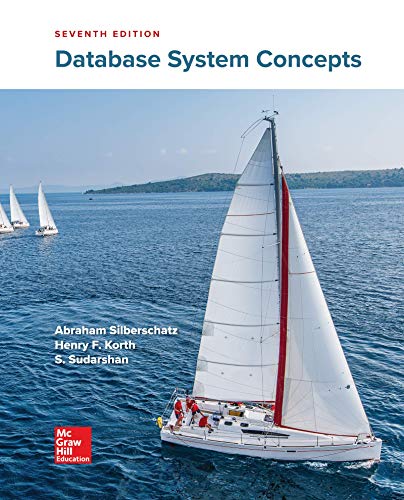
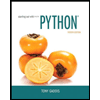
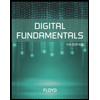
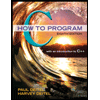
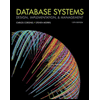
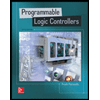