Any chance someone can give me some guidance on what to do here? I just need some advice on where I should start and how. Test this regular expression : ^(?!666|000)(?P<area>[0-8][0-9]{2})[- ]?(?!00)(?P<group>\d{2})[- ]?(?!0000)(?P<serial>\d{4})$ You should test all these cases: - all valid SSNs constructed with - connectors: 001-01-0001, 001-01-0002, ... 899-99-9999 - all invalid SSNs beginning with 666- - all invalid SSN's in the range of 900- to 999- - representative invalid samples equaling 0 in each SSN section: 000-xx-xxxx, xxx-00-xxxx and xxx-xx-0000 You should count and report the number of positive assertions performed. This number should be greater than 98M. Submit your python source file containing the tests. Submit the console output generated when your tests are run (Screen shot or text in a file) You can start with: import reclass SSN:debug = Falsepattern = r"^(?!666|000)(?P<area>[0-8][0-9]{2})[- ]?(?!00)(?P<group>\d{2})[- ]?(?!0000)(?P<serial>\d{4})$"ssn_regex = re.compile(pattern)@staticmethoddef is_valid(number):match = SSN.ssn_regex.match(number)if match == None:return Falsereturn match.group() == number Also, you may want to refer to an example we did in class: import unittestfrom dice_notation import DiceNotationclass TestDiceNotation(unittest.TestCase): def test_is_valid_3d6(self): self.assertTrue(DiceNotation.is_valid('3d6')) def test_is_valid_3d16(self): self.assertTrue(DiceNotation.is_valid('3d16')) def test_is_valid_potato(self): self.assertFalse(DiceNotation.is_valid('potato')) def test_is_valid_1d6_plus_plus_5(self): self.assertFalse(DiceNotation.is_valid('1d6++5')) def test_dice_notation_1d12_div_2_minus_1_roll100(self): notation = DiceNotation('1d12/2-1') for _ in range(100): roll = notation.roll() self.assertTrue(roll >= -1 and roll <= 5) if __name__ == '__main__': unittest.main()
Any chance someone can give me some guidance on what to do here? I just need some advice on where I should start and how.
Test this regular expression :
class SSN:
debug = False
pattern = r"^(?!666|000)(?P<area>[0-8][0-9]{2})[- ]?(?!00)(?P<group>\d{2})[- ]?(?!0000)(?P<serial>\d{4})$"
ssn_regex = re.compile(pattern)
@staticmethod
def is_valid(number):
match = SSN.ssn_regex.match(number)
if match == None:
return False
return match.group() == number
Also, you may want to refer to an example we did in class:
import unittest
from dice_notation import DiceNotation
class TestDiceNotation(unittest.TestCase):
def test_is_valid_3d6(self):
self.assertTrue(DiceNotation.is_valid('3d6'))
def test_is_valid_3d16(self):
self.assertTrue(DiceNotation.is_valid('3d16'))
def test_is_valid_potato(self):
self.assertFalse(DiceNotation.is_valid('potato'))
def test_is_valid_1d6_plus_plus_5(self):
self.assertFalse(DiceNotation.is_valid('1d6++5'))
def test_dice_notation_1d12_div_2_minus_1_roll100(self):
notation = DiceNotation('1d12/2-1')
for _ in range(100):
roll = notation.roll()
self.assertTrue(roll >= -1 and roll <= 5)
if __name__ == '__main__':
unittest.main()

Step by step
Solved in 2 steps

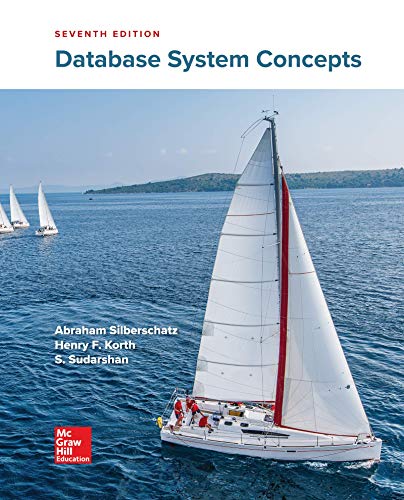
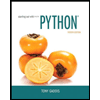
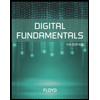
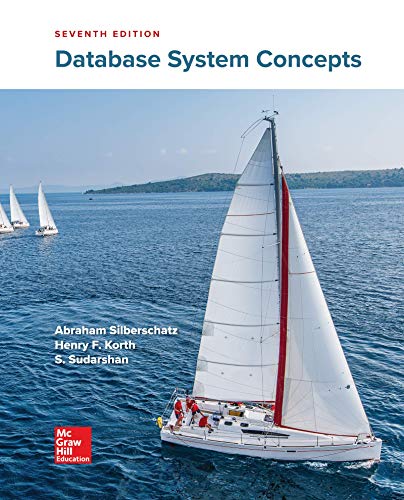
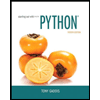
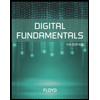
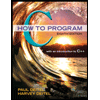
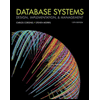
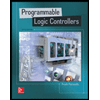