Answer in Java please and name the main class HW3 In this program, we will build a class that will simulate a baseball game. We are going to consider a double-header so we will only have 7 innings. 1. Our baseball game will have 7 innings. We are not going to consider ties and extra innings. You must declare a constant for the number of innings and this constant must be used to instantiate your arrays. Remember, in baseball, the visiting team bats in the top half of the inning. 2. You must have a constructor to initialize arrays and your Scanner object. You are only allowed to have one Scanner object. 3. The program will prompt the user to enter the names for two teams. a. The names must be entered from the same method. The name cannot be blank. 4. The user will be asked the score for the top and bottom of the inning. This must be done in a method, the same method. Your prompt from the method should be specific to the teams entered and which half of the inning you are in. Valid scores are 0 to 10, inclusive. This method should return an int, the score for that half of the inning 5. After the bottom of the 7th inning, you should not ask for more scores. 6. Scores must be entered in a method and the user should be prompted with the team's name and the inning. 7. At the end of the 7th inning, the scores for each inning should be printed, these will be totaled and displayed to the user. a. You must use a method to print and total the scores to determine the winner. 8. The winning team will then be printed along with the scores. This must be done in a separate method. If there is a tie, print that there is a tie with the scores. 9. Your program must provide meaningful error messages for: a. A blank team name. b. An invalid score This program requires the use instance variables. You will have at least 2 String variables for team names or an array to hold the names of the teams, one or two int arrays to hold the inning scores. You are allowed to use the key word static for the main method and for your constant only. Sample output: Please enter the name for Visitor Hawks Please enter the name for Home Please enter the name for Home Each team must have at least one character in their name. Grizzlies In the top half of inning 1, how many runs did Hawks score? 0 In the bottom half of inning 1, how many runs did Grizzlies score? 1 In the top half of inning 2, how many runs did Hawks score? 2 In the bottom half of inning 2, how many runs did Grizzlies score? 0 In the top half of inning 3, how many runs did Hawks score? -1 The score must be from 0 to 10. In the top half of inning 3, how many runs did Hawks score? 11 The score must be from 0 to 10. In the top half of inning 3, how many runs did Hawks score? 3 In the bottom half of inning 3, how many runs did Grizzlies score? 5 In the top half of inning 4, how many runs did Hawks score? 2
Answer in Java please and name the main class HW3 In this program, we will build a class that will simulate a baseball game. We are going to consider a double-header so we will only have 7 innings. 1. Our baseball game will have 7 innings. We are not going to consider ties and extra innings. You must declare a constant for the number of innings and this constant must be used to instantiate your arrays. Remember, in baseball, the visiting team bats in the top half of the inning. 2. You must have a constructor to initialize arrays and your Scanner object. You are only allowed to have one Scanner object. 3. The program will prompt the user to enter the names for two teams. a. The names must be entered from the same method. The name cannot be blank. 4. The user will be asked the score for the top and bottom of the inning. This must be done in a method, the same method. Your prompt from the method should be specific to the teams entered and which half of the inning you are in. Valid scores are 0 to 10, inclusive. This method should return an int, the score for that half of the inning 5. After the bottom of the 7th inning, you should not ask for more scores. 6. Scores must be entered in a method and the user should be prompted with the team's name and the inning. 7. At the end of the 7th inning, the scores for each inning should be printed, these will be totaled and displayed to the user. a. You must use a method to print and total the scores to determine the winner. 8. The winning team will then be printed along with the scores. This must be done in a separate method. If there is a tie, print that there is a tie with the scores. 9. Your program must provide meaningful error messages for: a. A blank team name. b. An invalid score This program requires the use instance variables. You will have at least 2 String variables for team names or an array to hold the names of the teams, one or two int arrays to hold the inning scores. You are allowed to use the key word static for the main method and for your constant only. Sample output: Please enter the name for Visitor Hawks Please enter the name for Home Please enter the name for Home Each team must have at least one character in their name. Grizzlies In the top half of inning 1, how many runs did Hawks score? 0 In the bottom half of inning 1, how many runs did Grizzlies score? 1 In the top half of inning 2, how many runs did Hawks score? 2 In the bottom half of inning 2, how many runs did Grizzlies score? 0 In the top half of inning 3, how many runs did Hawks score? -1 The score must be from 0 to 10. In the top half of inning 3, how many runs did Hawks score? 11 The score must be from 0 to 10. In the top half of inning 3, how many runs did Hawks score? 3 In the bottom half of inning 3, how many runs did Grizzlies score? 5 In the top half of inning 4, how many runs did Hawks score? 2
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question

Transcribed Image Text:Answer in Java please and name the main class HW3
In this program, we will build a class that will simulate a baseball game. We are going to consider a
double-header so we will only have 7 innings.
1. Our baseball game will have 7 innings. We are not going to consider ties and extra innings. You
must declare a constant for the number of innings and this constant must be used to instantiate
your arrays. Remember, in baseball, the visiting team bats in the top half of the inning.
2.
You must have a constructor to initialize arrays and your Scanner object. You are only allowed to
have one Scanner object.
3.
The program will prompt the user to enter the names for two teams.
4.
a. The names must be entered from the same method. The name cannot be blank.
The user will be asked the score for the top and bottom of the inning. This must be done in a
method, the same method. Your prompt from the method should be specific to the teams
entered and which half of the inning you are in. Valid scores are 0 to 10, inclusive. This method.
should return an int, the score for that half of the inning
After the bottom of the 7th inning, you should not ask for more scores.
Scores must be entered in a method and the user should be prompted with the team's name
and the inning.
7.
At the end of the 7th inning, the scores for each inning should be printed, these will be totaled
and displayed to the user.
a. You must use a method to print and total the scores to determine the winner.
8.
The winning team will then be printed along with the scores. This must be done in a separate
method. If there is a tie, print that there is a tie with the scores.
9. Your program must provide meaningful error messages for:
a. A blank team name.
b. An invalid score
5.
6.
This program requires the use instance variables. You will have at least 2 String variables for team
names or an array to hold the names of the teams, one or two int arrays to hold the inning scores.
You are allowed to use the key word static for the main method and for your constant only.
Sample output:
Please enter the name for Visitor
Hawks
Please enter the name for Home
Please enter the name for Home
Each team must have at least one character in their name.
Grizzlies
In the top half of inning 1, how many runs did Hawks score?
0
In the bottom half of inning 1, how many runs did Grizzlies score?
1
In the top half of inning 2, how many runs did Hawks score?
2
In the bottom half of inning 2, how many runs did Grizzlies score?
0
In the top half of inning 3, how many runs did Hawks score?
-1
The score must be from 0 to 10.
In the top half of inning 3, how many runs did Hawks score?
11
The score must be from 0 to 10.
In the top half of inning 3, how many runs did Hawks score?
3
In the bottom half of inning 3, how many runs did Grizzlies score?
5
In the top half of inning 4, how many runs did Hawks score?
2

Transcribed Image Text:In the bottom half of inning 2, how many runs did Grizzlies score?
0
In the top half of inning 3, how many runs did Hawks score?
-1
The score must be from 0 to 10.
In the top half of inning 3, how many runs did Hawks score?
11
The score must be from 0 to 10.
In the top half of inning 3, how many runs did Hawks score?
3
In the bottom half of inning 3, how many runs did Grizzlies score?
5
In the top half of inning 4, how many runs did Hawks score?
2
In the bottom half of inning 4, how many runs did Grizzlies score?
1
In the top half of inning 5, how many runs did Hawks score?
4
In the bottom half of inning 5, how many runs did Grizzlies score?
3
In the top half of inning 6, how many runs did Hawks score?
0
In the bottom half of inning 6, how many runs did Grizzlies score?
5
In the top half of inning 7, how many runs did Hawks score?
1
In the bottom half of inning 7, how many runs did Grizzlies score?
3
The game between Visitor and Home is over.
A recap of the scoring:
Visitor 0
2
Home 1
1
Grizzlies defeated the visiting team, Hawks, by a score of 18 to 12.
Thank you for playing baseball.
2
0
3
5
4
3
0
5
1
3
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 5 steps with 2 images

Recommended textbooks for you
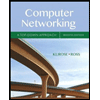
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
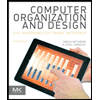
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
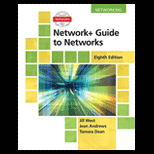
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
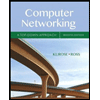
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
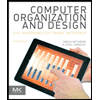
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
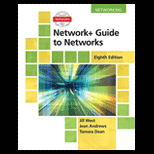
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
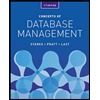
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
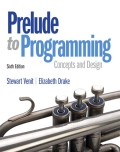
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
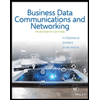
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY