Activity 1: Consider the following two programs. Write and execute them. (You can use line commands to execute the programs or run two Eclipse programs). import java.io.*; import java.net.*; import java.util.Scanner; public class Server{ public static void main(String argv[]) throws Exception{ /*1.connection*/ ServerSocket serverSocket = new ServerSocket(6789); Socket connectionSocket = serverSocket.accept(); /*2. A scanner or a BufferedReader for reading from the socket */ Scanner scanner = new Scanner(connectionSocket.getInputStream()); /*3. A DataOutputStream object to write in the socket*/ DataOutputStream outToClient=new DataOutputStream(connectionSocket.getOutputStream()); /*4. reading from the socket*/ String clientSentence = scanner.nextLine(); System.out.println("Received: " + clientSentence); /*5. modification of the sentence*/ String modifiedSentence =clientSentence.toUpperCase(); /*6. writing in the socket*/ outToClient.writeBytes(modifiedSentence+ '\n'); /*7.closing connections*/ scanner.close(); connectionSocket.close();
Activity 1: Consider the following two programs. Write and execute them. (You can use line commands to execute the programs or run two Eclipse programs). import java.io.*; import java.net.*; import java.util.Scanner; public class Server{ public static void main(String argv[]) throws Exception{ /*1.connection*/ ServerSocket serverSocket = new ServerSocket(6789); Socket connectionSocket = serverSocket.accept(); /*2. A scanner or a BufferedReader for reading from the socket */ Scanner scanner = new Scanner(connectionSocket.getInputStream()); /*3. A DataOutputStream object to write in the socket*/ DataOutputStream outToClient=new DataOutputStream(connectionSocket.getOutputStream()); /*4. reading from the socket*/ String clientSentence = scanner.nextLine(); System.out.println("Received: " + clientSentence); /*5. modification of the sentence*/ String modifiedSentence =clientSentence.toUpperCase(); /*6. writing in the socket*/ outToClient.writeBytes(modifiedSentence+ '\n'); /*7.closing connections*/ scanner.close(); connectionSocket.close();
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
Solution question number 2
![Lab Activities:
Activity 1:
Consider the following two programs. Write and execute them. (You can use line commands to
execute the programs or run two Eclipse programs).
import java.io.*; import java.net.*;
import java.util.Scanner;
public class Server{
public static void main(String argv[]) throws Exception{
/*1.connection*/
ServerSocket serverSocket = new ServerSocket(6789);
Socket connectionSocket = serverSocket.accept();
/*2. A scanner or a BufferedReader for reading from the socket */
Scanner scanner = new Scanner(connectionSocket.getInputStream());
| /*3. A DataOutputStream object to write in the socket*/
DataOutputStream outToClient=new DataOutputStream(connectionSocket.getOutputStream());
/*4. reading from the socket*/
String clientSentence = scanner.nextLine();
System.out.println("Received: " + clientSentence);
/*5. modification of the sentence*/
String modifiedSentence =clientSentence.toUpperCase();
/*6. writing in the socket*/
outToClient.writeBytes(modifiedSentence+ '\n');
/*7.closing connections*/
scanner.close();
connectionSocket.close();
}}
Omer Bin Hussain
18 | P age](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F30d90cb2-5a82-47cb-ae84-879577ccd5e8%2Fdb48080c-a9ae-4c2b-b8f2-397df9e2387c%2Fapancxp_processed.jpeg&w=3840&q=75)
Transcribed Image Text:Lab Activities:
Activity 1:
Consider the following two programs. Write and execute them. (You can use line commands to
execute the programs or run two Eclipse programs).
import java.io.*; import java.net.*;
import java.util.Scanner;
public class Server{
public static void main(String argv[]) throws Exception{
/*1.connection*/
ServerSocket serverSocket = new ServerSocket(6789);
Socket connectionSocket = serverSocket.accept();
/*2. A scanner or a BufferedReader for reading from the socket */
Scanner scanner = new Scanner(connectionSocket.getInputStream());
| /*3. A DataOutputStream object to write in the socket*/
DataOutputStream outToClient=new DataOutputStream(connectionSocket.getOutputStream());
/*4. reading from the socket*/
String clientSentence = scanner.nextLine();
System.out.println("Received: " + clientSentence);
/*5. modification of the sentence*/
String modifiedSentence =clientSentence.toUpperCase();
/*6. writing in the socket*/
outToClient.writeBytes(modifiedSentence+ '\n');
/*7.closing connections*/
scanner.close();
connectionSocket.close();
}}
Omer Bin Hussain
18 | P age
![import java.io.*;
import java.net.*;
import java.util.Scanner;
public class Client{
public static void main(String argv[]) throws Exception {
/*1. Connection*/
Socket connectionSocket = new Socket ("localhost",6789);
Scanner scanner, scannerFromSocket;
DataOutputStream outToServer;
String sentenceFromServer;
String clientSentence;
/*2. Scanner to read from keyboard */
scanner = new Scanner(System.in);
/*3. Scanner to read from the socket */
scannerFromSocket = new Scanner(connectionSocket.getInputStream0);
/*4. DataOutputStream to write in the socket */
outToServer=new DataOutputStream(connectionSocket.getOutputStream());
/*5. receiving and sending messages*/
clientSentence =scanner.nextLine();
outToServer.writeBytes(clientSentence + '\n');
sentenceFromServer=scannerFromSocket.nextLine();
| System.out.println(sentenceFromServer);
scannerFromSocket.close();
scanner.close();
connectionSocket.close();
}}
1. What happens if you execute the client program before the server one?
2. Add a loop to the programs to make it repeat the task many times.
3. Modify the programs to create a chat between the server and the client: The client sends a text
to the server. The server prints the received text and sends an answer to the client. Use a loop to
repeat the task.](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F30d90cb2-5a82-47cb-ae84-879577ccd5e8%2Fdb48080c-a9ae-4c2b-b8f2-397df9e2387c%2F3zxg3ua_processed.jpeg&w=3840&q=75)
Transcribed Image Text:import java.io.*;
import java.net.*;
import java.util.Scanner;
public class Client{
public static void main(String argv[]) throws Exception {
/*1. Connection*/
Socket connectionSocket = new Socket ("localhost",6789);
Scanner scanner, scannerFromSocket;
DataOutputStream outToServer;
String sentenceFromServer;
String clientSentence;
/*2. Scanner to read from keyboard */
scanner = new Scanner(System.in);
/*3. Scanner to read from the socket */
scannerFromSocket = new Scanner(connectionSocket.getInputStream0);
/*4. DataOutputStream to write in the socket */
outToServer=new DataOutputStream(connectionSocket.getOutputStream());
/*5. receiving and sending messages*/
clientSentence =scanner.nextLine();
outToServer.writeBytes(clientSentence + '\n');
sentenceFromServer=scannerFromSocket.nextLine();
| System.out.println(sentenceFromServer);
scannerFromSocket.close();
scanner.close();
connectionSocket.close();
}}
1. What happens if you execute the client program before the server one?
2. Add a loop to the programs to make it repeat the task many times.
3. Modify the programs to create a chat between the server and the client: The client sends a text
to the server. The server prints the received text and sends an answer to the client. Use a loop to
repeat the task.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 2 steps

Recommended textbooks for you
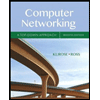
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
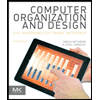
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
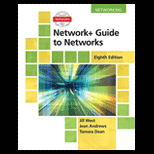
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
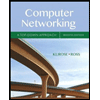
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
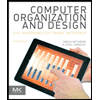
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
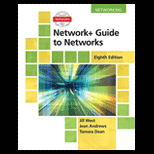
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
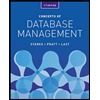
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
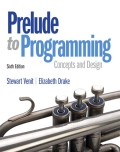
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
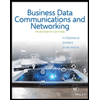
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY