4. Consider two sequences X = ABCBDA and Y = CABBDA. Find LCS (Longest Common Subsequence) performing: 4.a Compute c[] and b[] (like we did in Lec9.1), draw the table. 4.b Print LCS with PrintLCSO method, describe the steps. 4.c Convert PrintLCSO from recursive into an iterative algorithm. Write your Pseudocode with commentaries. 4.d Modify the code (LCSLength() and PrintLCSO) adding a parameter I (length of a subsequence) s.t. it prints the first common subsequence of length / or prints "No common subsequence of length I" if there is no common subsequence of length 1.
4. Consider two sequences X = ABCBDA and Y = CABBDA. Find LCS (Longest Common Subsequence) performing: 4.a Compute c[] and b[] (like we did in Lec9.1), draw the table. 4.b Print LCS with PrintLCSO method, describe the steps. 4.c Convert PrintLCSO from recursive into an iterative algorithm. Write your Pseudocode with commentaries. 4.d Modify the code (LCSLength() and PrintLCSO) adding a parameter I (length of a subsequence) s.t. it prints the first common subsequence of length / or prints "No common subsequence of length I" if there is no common subsequence of length 1.
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
4. Consider two sequences X = ABCBDA and Y = CABBDA. Find LCS (Longest Common Subsequence) performing:
4.a Compute c[] and b[] (like we did in Lec9.1), draw the table.
4.b Print LCS with PrintLCSO method, describe the steps.
4.c Convert PrintLCSO from recursive into an iterative
4.d Modify the code (LCSLength() and PrintLCSO) adding a parameter I (length of a subsequence) s.t. it prints the first common subsequence of length / or prints "No common subsequence of length I" if there is no common subsequence of length 1.
![Computing the Length
LCSLength(X, Y, m, n)
let b[1..m, 1..n] and c[0..m, 0..n] be new tables (2d arrays)
for i = 1 tom
c[i, 0] = 0 // first column
jo 1 2 3 4 5 6
for j = 0 to n
y, BDCABA
x 0 0 0 00 0 0
i
В
c[0, j] = 0 // first row
for i = 1 tom
for j = 1 to n
if x, == y;
c[i, j] = c[i - 1, j - 1] + 1
bli, j] = "R"
else if c[i - 1, j] > c[i, j - 1]
c[i, j] = c[i - 1, j]
b[i, j] = “ ↑ "
1 0 1 0
10 k 1
+1 K 1
2
в о
+ 1
+ 1
↑ 1 R 2 + 2
↑ 1
↑ 1 k 2 +2 1 2 1 2
4
B 01 ↑ 1
↑ 2
1 2 K 3
+ 3
%3D
D
↑ 1 R 2
1 2 1 2 1 3 ↑ 3
А
↑ 1
↑ 2
1 2 K 3
↑ 3
else c[i, j] = c[i, j - 1]
b[i, j] = “+"
7
В
↑ 2
↑ 3
return c and b
4.
4.
A](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F527791e7-2974-4cd6-8277-94a9a88e897a%2F9b721487-56aa-41e0-89f4-844bd3983076%2F8q85ix_processed.png&w=3840&q=75)
Transcribed Image Text:Computing the Length
LCSLength(X, Y, m, n)
let b[1..m, 1..n] and c[0..m, 0..n] be new tables (2d arrays)
for i = 1 tom
c[i, 0] = 0 // first column
jo 1 2 3 4 5 6
for j = 0 to n
y, BDCABA
x 0 0 0 00 0 0
i
В
c[0, j] = 0 // first row
for i = 1 tom
for j = 1 to n
if x, == y;
c[i, j] = c[i - 1, j - 1] + 1
bli, j] = "R"
else if c[i - 1, j] > c[i, j - 1]
c[i, j] = c[i - 1, j]
b[i, j] = “ ↑ "
1 0 1 0
10 k 1
+1 K 1
2
в о
+ 1
+ 1
↑ 1 R 2 + 2
↑ 1
↑ 1 k 2 +2 1 2 1 2
4
B 01 ↑ 1
↑ 2
1 2 K 3
+ 3
%3D
D
↑ 1 R 2
1 2 1 2 1 3 ↑ 3
А
↑ 1
↑ 2
1 2 K 3
↑ 3
else c[i, j] = c[i, j - 1]
b[i, j] = “+"
7
В
↑ 2
↑ 3
return c and b
4.
4.
A
![Printing the LCS
PrintLCS(b,X, i, j)
if i == 0 or j ==0
return None
if b[i, j] == "R"
PrintLCS(b, X, i - 1, j - 1)
jo 1
2
3 4 5 6
x BD CABA
3 A
i
В
В
print x,
X 0 0 0
0 0 0 0
else if b[i, j] == "↑"
PrintLCS(b, X, i - 1, j)
1
A 0 10
10 k 1
+1R 1
2
B 0
+ 1
+ 1
↑ 1 k 2 + 2
else PrintLCS(b, X, i, j - 1)
co 11
↑ 1 k 2
↑ 2
+ 2 1 2
Initial call: PrintLCS(b, X, m, n)
4 B0k 1
↑ 1 12 1 2 K 3 + 3
Running time: 0(m+n).
D0 11 2
A 0 11
7 B01
1 2 1 2
↑ 3 1 3
Overall running time for LCS is
↑ 2
↑ 2 R 3 1 3 R 4
Ө (mn) + Ө(m+n) —
O(mn)
↑ 2 1 2 1 3 R 4
↑ 4](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F527791e7-2974-4cd6-8277-94a9a88e897a%2F9b721487-56aa-41e0-89f4-844bd3983076%2F1zdt2e_processed.png&w=3840&q=75)
Transcribed Image Text:Printing the LCS
PrintLCS(b,X, i, j)
if i == 0 or j ==0
return None
if b[i, j] == "R"
PrintLCS(b, X, i - 1, j - 1)
jo 1
2
3 4 5 6
x BD CABA
3 A
i
В
В
print x,
X 0 0 0
0 0 0 0
else if b[i, j] == "↑"
PrintLCS(b, X, i - 1, j)
1
A 0 10
10 k 1
+1R 1
2
B 0
+ 1
+ 1
↑ 1 k 2 + 2
else PrintLCS(b, X, i, j - 1)
co 11
↑ 1 k 2
↑ 2
+ 2 1 2
Initial call: PrintLCS(b, X, m, n)
4 B0k 1
↑ 1 12 1 2 K 3 + 3
Running time: 0(m+n).
D0 11 2
A 0 11
7 B01
1 2 1 2
↑ 3 1 3
Overall running time for LCS is
↑ 2
↑ 2 R 3 1 3 R 4
Ө (mn) + Ө(m+n) —
O(mn)
↑ 2 1 2 1 3 R 4
↑ 4
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 2 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
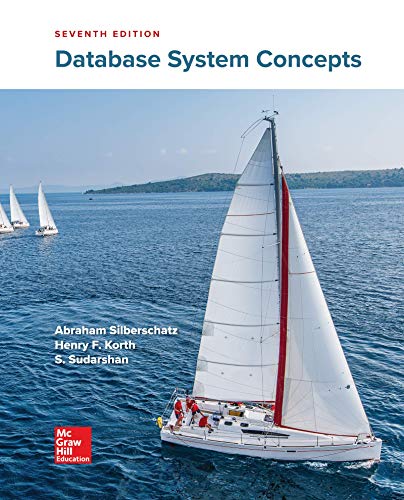
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
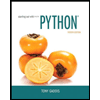
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
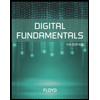
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
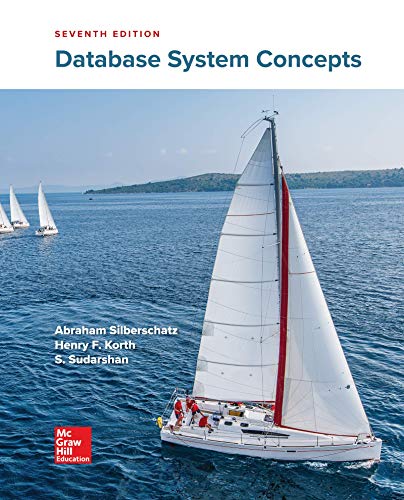
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
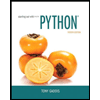
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
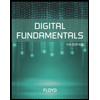
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
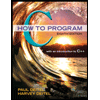
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
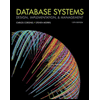
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
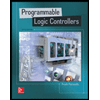
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education