1. Implement a phone book using a linked list structure. In this phone book, you are going to store a name, last name, email, and phone number. 2. Implement an insert operation to the phone book. With the insert operation, you're going to add the name, last
1. Implement a phone book using a linked list structure. In this phone book, you are going to store a name, last name, email, and phone number. 2. Implement an insert operation to the phone book. With the insert operation, you're going to add the name, last
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
Use Java

Transcribed Image Text:1
Week27Phonebook_Detailed_Exp X +
72°F
Cloudy
of 3
File | C:/Users/Igariok/Downloads/Week27
Q
Phonebook_Detailed_Explanation.pdf
CD Page view
●
Y
+
●
← →
●
A Read aloud
T Add text
Y
Phonebook with LinkedList
1. Implement a phone book using a linked list structure. In this phone book, you are going to store a name, last
name, email, and phone number.
Draw
2. Implement an insert operation to the phone book. With the insert operation, you're going to add the name, last
name, email, and phone number of the person.
3. Implement the following methods:
findByName: Will return the information of the first record matching the name you send.
findAllByLastName: Will return an ArrayList of all entries matching the last name you send.
deleteByName: Will delete the first record matching the name you send to this method.
deleteAll MatchingLastName: Will delete all entries matching the last name.
findAll: Return all records as an ArrayList.
printPhoneBook: Will print all entries in the phone book.
Highlight
Discussion: Let's discuss the following after you do the above task.
1. What is the time complexity of the above operations?
2. How can we come up with O(1) with the findByName operation? (lovely hash map)
Phonebook with LinkedList
Erase
1. Implement a phone book using a linked list structure. In this phone book, you are going
to store a name last name email and phone number
S
ENG
{"
60
@
0
X
05:14 PM
10/24/2022
A
![2
Week27Phonebook_Detailed_Exp X +
72°F
Cloudy
of 3
File | C:/Users/Igariok/Downloads/Week27
Q
Phonebook_Detailed_Explanation.pdf
CD Page view
+
public class Phone BookNode {
public Contact contact;
public PhoneBookNode next;
}
A Read aloud
}
Phonebook with LinkedList
1. Implement a phone book using a linked list structure. In this phone book, you are going
to store a name, last name, email, and phone number.
public Phone BookNode (Contact contact) {
this.contact = contact;
This Node class is designed for singly
linked list Node implementation, and
[T] Add text
contact object is responsible for holding the
value.
It is a different version of the Node class we
used before.
Draw
@NoArgsConstructor
@ALLArgsConstructor
@ToString
public class Contact {
}
public String firstName;
public String lastName;
public String email;
public String phoneNumber;
static class ListNode {
int value;
ListNode next;
ListNode(int x) {
value = x;
Highlight
Phonebook with LinkedList
This class is designed for singly linked list implementation. It has almost the same structure as the
Built-in LinkedList class of Java.
The most important difference is that this custom LinkedList implementation used
DhonoDookNodo olons co Nodo
Erase
S
ENG
{"
60
@
0
X
⠀
05:15 PM
10/24/2022](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F3225f6d7-278c-43ec-8bb1-b18ff563550d%2F52df48d3-1bce-4bef-b162-74c89b1bd2e0%2Fn72jcy_processed.png&w=3840&q=75)
Transcribed Image Text:2
Week27Phonebook_Detailed_Exp X +
72°F
Cloudy
of 3
File | C:/Users/Igariok/Downloads/Week27
Q
Phonebook_Detailed_Explanation.pdf
CD Page view
+
public class Phone BookNode {
public Contact contact;
public PhoneBookNode next;
}
A Read aloud
}
Phonebook with LinkedList
1. Implement a phone book using a linked list structure. In this phone book, you are going
to store a name, last name, email, and phone number.
public Phone BookNode (Contact contact) {
this.contact = contact;
This Node class is designed for singly
linked list Node implementation, and
[T] Add text
contact object is responsible for holding the
value.
It is a different version of the Node class we
used before.
Draw
@NoArgsConstructor
@ALLArgsConstructor
@ToString
public class Contact {
}
public String firstName;
public String lastName;
public String email;
public String phoneNumber;
static class ListNode {
int value;
ListNode next;
ListNode(int x) {
value = x;
Highlight
Phonebook with LinkedList
This class is designed for singly linked list implementation. It has almost the same structure as the
Built-in LinkedList class of Java.
The most important difference is that this custom LinkedList implementation used
DhonoDookNodo olons co Nodo
Erase
S
ENG
{"
60
@
0
X
⠀
05:15 PM
10/24/2022
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
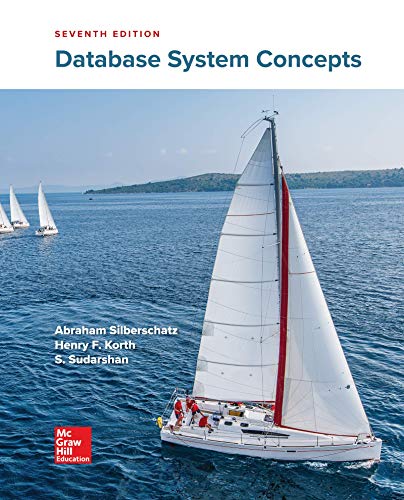
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
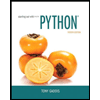
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
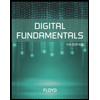
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
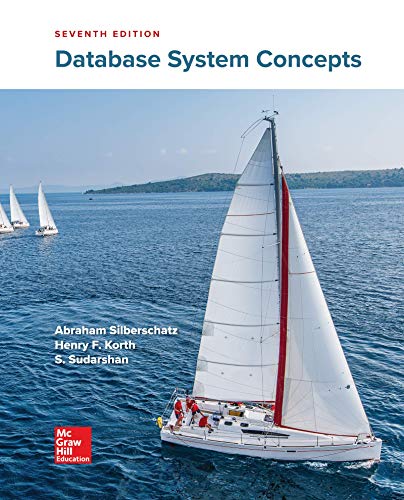
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
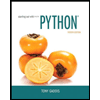
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
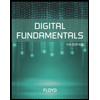
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
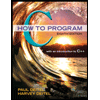
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
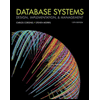
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
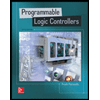
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education