1. Deck.java The Deck class represents a deck of cards. It starts off with 52 cards, but as cards are dealt from the deck. the number of cards becomes smaller. The class has one private instance variablo that stores the Carde that are currently in the Deck: private Card] cards; Important Note: The first Card in the array will be considered the card that is at the top of the deck. When cards aro removed from the deck they will be removed from the front of the array. You must implement the following methods for the Deck class: • public Deck0- This constructor initializes the Deck with 52 card objects, representing the 52 cards that are in a standard deck. The cards must be ordered as in the diagram below: Important: The FIRST card in your array should be the Ace of Spades, and the LAST card should be the King of Diamonds. Note that in the picture below, the Ace of Spades is at the TOP of the deck, which is to the left. A234567890J 567890 • public Deck(Deck other)- Standard copy constructor. Note that it is okay to make a shallow copy of the array of cards. (The Card class is immutable, so alinsing Cards is not a problem.) • public Card getCardAt(int position) - Returns the card that is at the specified position in the array (Uses the usual 0-based indexing.) • public int getNumCards)- Returns the sizo of the array of Cards. (It starts off equal to 52, but becomes smaller as cards are dealt from the deck.) • public void shuffle()- This method will re-arrange the cards that are in the deck. The idea is that the deck will be divided into two "packets the top half and the bottom half. The new array of cards will consist of: the first card from the top packet, followed by the first card from the bottom packet, followed by the second card from the top packet, followed by the second card from the bottom packet, etc. Important: If there are an odd number of cards, the top packet should have one more card than the bottom packet. Remember that the top of the deck is considered to be the front of the array. In the pictures below, remember that the top of the deck appears at the left side. Below are two examples of how the shuffle should work Example 1 (even number of cards): Before Shuffling A234567890 5456
1. Deck.java The Deck class represents a deck of cards. It starts off with 52 cards, but as cards are dealt from the deck. the number of cards becomes smaller. The class has one private instance variablo that stores the Carde that are currently in the Deck: private Card] cards; Important Note: The first Card in the array will be considered the card that is at the top of the deck. When cards aro removed from the deck they will be removed from the front of the array. You must implement the following methods for the Deck class: • public Deck0- This constructor initializes the Deck with 52 card objects, representing the 52 cards that are in a standard deck. The cards must be ordered as in the diagram below: Important: The FIRST card in your array should be the Ace of Spades, and the LAST card should be the King of Diamonds. Note that in the picture below, the Ace of Spades is at the TOP of the deck, which is to the left. A234567890J 567890 • public Deck(Deck other)- Standard copy constructor. Note that it is okay to make a shallow copy of the array of cards. (The Card class is immutable, so alinsing Cards is not a problem.) • public Card getCardAt(int position) - Returns the card that is at the specified position in the array (Uses the usual 0-based indexing.) • public int getNumCards)- Returns the sizo of the array of Cards. (It starts off equal to 52, but becomes smaller as cards are dealt from the deck.) • public void shuffle()- This method will re-arrange the cards that are in the deck. The idea is that the deck will be divided into two "packets the top half and the bottom half. The new array of cards will consist of: the first card from the top packet, followed by the first card from the bottom packet, followed by the second card from the top packet, followed by the second card from the bottom packet, etc. Important: If there are an odd number of cards, the top packet should have one more card than the bottom packet. Remember that the top of the deck is considered to be the front of the array. In the pictures below, remember that the top of the deck appears at the left side. Below are two examples of how the shuffle should work Example 1 (even number of cards): Before Shuffling A234567890 5456
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
![1. Deck.java
The Deck class represents a deck of cards. It starts off with 52 cards, but as cards are dealt from the deck.
the number of cards becomes smaller. The class has one private instance variable that stores the Cards
that are currently in the Deck:
private Card] carde;
Important Note: The first Card in the array will be considered the card that Is at the top of the deck.
Whon cards aro removed from the deck they will be removed from the front of the array. You must
implement the flollowing methods for the Deck class:
• public Dock) This constructor initializes the Deck with 52 card objacts, representing the 52 cards
that are in a standard deck. The cards must be ordered as in the diagram below: Important: The
FIRST card in your array should be the Ace of Spades, and the LAST card should be the King of
Diamonds. Note that in the picture below, the Ace of Spades is at the TOP of the deck, which is to
the left.
A234567890 J.0KA234567
25456
10KA2 S45 57|8|90U.0高
• public Deck(Deck other) Standard copy constructor. Note that it is okay to make a shallow copy
of the array of cards. (The Card class is immutable, so alineng Cards is not a problem.)
• public Card getCardAt(int position)- Returns the card that is at the specified position in the array
(Uses the usual 0-based indexing.)
• public int getNumCards)- Returns the szo of the array of Cards. (It starts off equal to 52, but
becomes smaller as cards are dealt from the deck.)
• public vold shuffle() - This method will re-arrange the cards that are in the deck. The idea is that the
deck will be divided into two packets" the top half and the bottom half, The new array of cards
will consist of: the first card from the top packet, followed by the first card from the bottom packet,
followed by the second card from the top packet, followed by the second card from the bottom
packet, etc. Important: If there are an odd number of cards, the top packet should have one more
card than the bottom packet. Remember that the top of the deck is considered to be the front of the
array. In the pictures below, remember that the top of the deck appears at the left side. Below
are two examples of how the shuffle should work.
Example 1 (even number of cards):
Before Shuffling
A2G4507890
455
1900](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Ff24cd5f0-c16f-419b-a958-980dab663b78%2Fd63024d3-92ec-4747-923b-3eb78af271fb%2F32fsjui_processed.jpeg&w=3840&q=75)
Transcribed Image Text:1. Deck.java
The Deck class represents a deck of cards. It starts off with 52 cards, but as cards are dealt from the deck.
the number of cards becomes smaller. The class has one private instance variable that stores the Cards
that are currently in the Deck:
private Card] carde;
Important Note: The first Card in the array will be considered the card that Is at the top of the deck.
Whon cards aro removed from the deck they will be removed from the front of the array. You must
implement the flollowing methods for the Deck class:
• public Dock) This constructor initializes the Deck with 52 card objacts, representing the 52 cards
that are in a standard deck. The cards must be ordered as in the diagram below: Important: The
FIRST card in your array should be the Ace of Spades, and the LAST card should be the King of
Diamonds. Note that in the picture below, the Ace of Spades is at the TOP of the deck, which is to
the left.
A234567890 J.0KA234567
25456
10KA2 S45 57|8|90U.0高
• public Deck(Deck other) Standard copy constructor. Note that it is okay to make a shallow copy
of the array of cards. (The Card class is immutable, so alineng Cards is not a problem.)
• public Card getCardAt(int position)- Returns the card that is at the specified position in the array
(Uses the usual 0-based indexing.)
• public int getNumCards)- Returns the szo of the array of Cards. (It starts off equal to 52, but
becomes smaller as cards are dealt from the deck.)
• public vold shuffle() - This method will re-arrange the cards that are in the deck. The idea is that the
deck will be divided into two packets" the top half and the bottom half, The new array of cards
will consist of: the first card from the top packet, followed by the first card from the bottom packet,
followed by the second card from the top packet, followed by the second card from the bottom
packet, etc. Important: If there are an odd number of cards, the top packet should have one more
card than the bottom packet. Remember that the top of the deck is considered to be the front of the
array. In the pictures below, remember that the top of the deck appears at the left side. Below
are two examples of how the shuffle should work.
Example 1 (even number of cards):
Before Shuffling
A2G4507890
455
1900

Transcribed Image Text:413/2021
Proea 7
Projed
After Shuffling
- Copy the cards that are not being dealt from the original array into the new one.
Assign the instance variable "cards" so that it refers to the new array
S53627
2 PokerHandEvaluator.java
This dass consists of several static methods that you will write. The prototypes for the methods are:
public static boolean hasPair(Card cards)
• public static boolean hasTwoPair(Card) cards)
public static boolean hasThreeOIAKind(Card) cards)
- public static boolean hasStraight(Card cards)
• public static boolean hasFlush(Card) cards)
public static boolean hasFullHouse(Card) cards)
public static boolean hasFourOfAKind(Card) cards)
Example 2 (odd number of cards):
Before Shuffling
567890
789
public static boolean hasStraightFlush(Card) cards)
K NTES
After Shuffling
The parameter for each of these methods will be an array of exactly 5 cards. Each method will return true or false,
based on whether or not the given set of cards satisfies the poker hand being evaluated in the method. For
example, the has TwoPair method will return true if the set of cards has two pairs of different values (e.g. a pair of
4's and a pair of Jacks). If you need to review the various "poker hands' being tested in the methods, please take
another look at the Poker Hand Page (pokerHands html).
5 06
1950
Important: Each of these methods checks whether or not the set of cards satisfies the given poker hand, but it
does not care if it could also satisty a better hand. For example, if the current hand is
• public void cutfint position) - This method divides the deck into two subpackets: The part above
the specified position, and the part that is at the specified position or below. The two subpackets are
reversed (the top packet is placed on the bottom and the bottom packet is placed on the top.) The
position value uses 0-based Indexing as usual, so the card at the top of the deck (to the left in the
diagrams) is at position 0. Here is an example:
<Ace of diamonds, Ace of spades, Ace of hearts, Jack of Spades, Jack of Diamonds>
then the results of calling each method should be as indicated below:
hasPair -- true (there is at least one pair)
hasTwoPair- true (there are two pairs of distinct values)
• hasThreeOfAKind - true (there are three Aces)
Before cut
A23456789OJALA234567890OTA234567890J0KA23456789DOCK
hasStraight -- false
• hasFlushfalse
hasFullHouse -- true (there are three Aces and two Jacks -- that makes a "full house")
. hasFourOtAKind -- false
• hasStraightFlush- false
After cutting at position 4. (The 5 of spades was at position 4 in the diagram above.)
Important: In order for a hand to quality as "Two Pair", it must have two pairs of distinct values -- in particular,
having four-of-a-kind does not also count as two pair. For example, if the current hand is
567890J0EAZS436799)
89JOKA2 54
2 of diamonds, 2 of spades, 2 of hearts, 2 of clubs, 9 of spades>
then the results of calling each method should be as indicated below:
• public Card deal(int numCards) - This method will remove the specified number of cards from the
top of the deck and return them as an array. For example, it the parameter is 4, then the first four
cards in the deck will be returned as an array of size 4. Important: The cards will be removed from
the front of the 'cards" array, not the back. Hint: The 'cards" array will need to be resized. Here is the
idea:
• hasPair- true (there is at least one pair)
• hasTwoPair -- false (there are not two pairs of distinct values)
. has ThreeOfAKind-- true (there are three 2's)
• hasStraight -- false
• hasFlush - false
• hasFulHouse-- false
• hasFourOtAKind - true (there are four 2's)
• hasStraightFlush - false
• Make a new array (call it "smaller) that is the same size as the current deck of cards minus the
number of cads being dealt.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 6 steps with 11 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
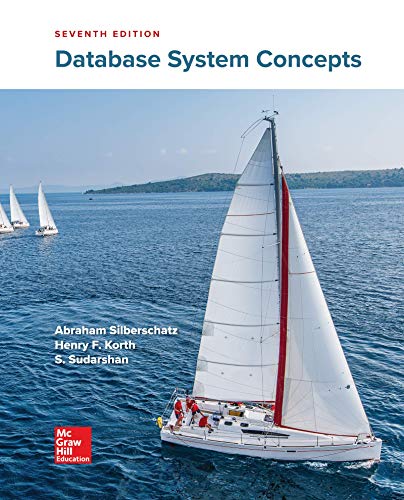
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
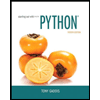
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
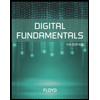
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
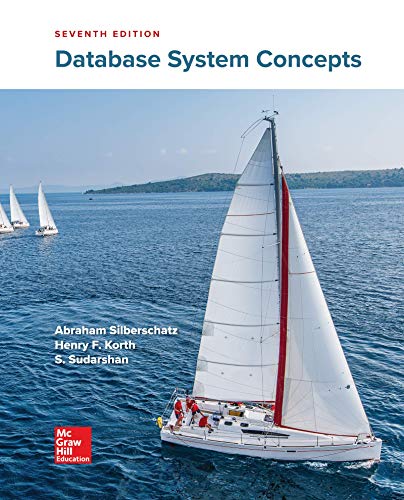
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
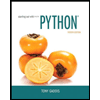
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
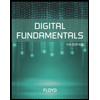
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
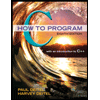
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
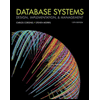
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
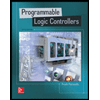
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education