Ch05 - Hw5-2
.docx
keyboard_arrow_up
School
California State University, Long Beach *
*We aren’t endorsed by this school
Course
340
Subject
Business
Date
May 10, 2024
Type
docx
Pages
3
Uploaded by DrFire6669
California State University, Long Beach
Information Systems College of Business Administration
IS 340 – Business Application Programming
Ch05 Functions
Professor: Jorge Echavarria
Week 05 Assignment
Chapter 05 (Functions)
Before you complete each of the following exercises, print this page
out and use it to keep notes about your progress. When you are done with each exercise, "check it off" of the list so you can keep track of your progress. Student Learning Outcomes:
1.
Students will implement different programs using each of the following: basic computation, simple I/O, conditional and iterative structures.
2.
Students will write programs that make use of function definitions, decomposition, parameter passing, and arrays.
3.
Students will write programs that implement programmer-defined types (classes or structures).
Submit the Assignment into “Assignment 5” Dropbox Folder. Attach it with the name LastnameFirstname_Assign5.docx (or .doc for Office 2003)
Don't forget to write your name and the chapter number and any relevant information on the top of
the document as well
The deadline
for all the assignments will be posted in Canvas
All Test Cases must be demonstrated in order to get full credit 1.
Write pseudocode/algorithm for a function that translates a telephone number with letters in it (such as 1-800-FLOWERS) into the actual phone number. Use the standard letters on a phone pad. Make sure your function has an input and a return result. Also, be specific on how the numbers are
translated in your algorithm (don’t use a “dictionary” or “map”. Use what we’ve learned: “if blocks”) 4 pts
IS 340: Ch05 Assignment
Page 1
California State University, Long Beach
Information Systems College of Business Administration
IS 340 – Business Application Programming
Ch05 Functions
Professor: Jorge Echavarria
2.
Write the following function and provide a program to test it (main and function in one .py) 5 pts
def repeat(string, n, delim)
that returns the string string
repeated n
times, separated by the string delim
. For example repeat(“ho”, 3, “,”) returns “ho, ho, ho”.
a)
Your code with comments
b)
A screenshot of the execution
3.
Write the following functions and provide a program to test them (main and all functions in one .py). 6 pts
A)
def allTheSame(x, y, z) (returning True if the arguments are all the same, otherwise False)
B)
def allDifferent(x, y, z) (returning True if the arguments are all different, otherwise False)
C)
def sorted(x, y, z) (returning True if the arguments are sorted in ascending, otherwise False)
Note
: Make sure your output has meaning (Don’t just print “True” or “False”)
a)
Your code with comments
b)
A screenshot of the execution
4.
Write the following function and provide a program to test it (main and function in one .py) 5 pts
Def countVowels(string)
that returns a count of all vowels in the string string
. Vowels are letters a, e, i, o, and u and their uppercase variants. Your main program should ask the user to input a string and then print the results.
Test Case:
Please enter a string: sentencE
The total number of vowels = 3
a)
Your code with comments
b)
A screenshot of the execution
5.
Write the following function and provide a program to test it (main and function in one .py) 5 pts
def readFloat(prompt)
that displays the prompt string, followed by a space, reads a floating-point number in, and returns it. Below is how you’re going to call the function:
salary = readFloat(“Please enter your salary:”)
percentageRaise = readFloat(“What percentage raise would you like?”)
print("The salary is", salary)
print("The raise percentage is", percentageRaise)
a)
Your code with comments
b)
A screenshot of the execution
IS 340: Ch05 Assignment
Page 2
Your preview ends here
Eager to read complete document? Join bartleby learn and gain access to the full version
- Access to all documents
- Unlimited textbook solutions
- 24/7 expert homework help
Related Questions
Student: Angelea Tingey
The following table details the tasks required for Indiana-based Frank Pianki Industries to manufacture a fully
portable industrial vacuum cleaner. The times in the table are in minutes. Demand forecasts indicate a need to
operate with a cycle time of 10 minutes.
Performance
Activity Activity Description Time (mins)
A Attach wheels to tub
B
Attach motor to lid
C
Attach battery pack
D
Attach safety cutoff
E
Attach filters
Attach lid to tub
(1) Yes
O No
F
G
H
I
Assemble
attachments
Function test
Final inspection
Packing
c) The goal is to have 100% efficiency. Is this possible? (1).
d) The theoretical minimum number of workstations =
number)
5.0
1.8
3.0
3.8
3.0
2.0
3.0
Instructor: Angela Johnson
Course: MGT-455-0500
4.0
2.0
2.0
Predecessors
B
с
B
A, E
a-b) One of the possible assignment of tasks to workstations is shown correctly in Fig. 2
The efficiency of the assembly line with 3 workstations =
decimal places)
D, F, G
H
I
% (enter your response rounded to two…
arrow_forward
You are alone at home and have to prepare a bread sandwich for yourself. The following preparation activities and time taken are given in the table below:
Task
Description
Predecessor
Time (Minutes)
A
Purchase of bread
-
20
B
Take cheese and apply on bread
A
5
C
Get onions from freezer
A
1
D
Fry onions with pepper
B,C
6
E
Purchase sauce for bread
-
15
F
Toast bread
B,C
4
G
Assemble bread and fried onions
F
2
H
Arrange in plat
G
1
Determine:
a. While purchasing sauce, you met a friend and spoke to him for 6 minutes. Did this cause any delay in the preparation?
arrow_forward
Weighted-Average Method Arsenio Company manufactures a single product that goes throughtwo processes, mixing and cooking. The following data pertain to the mixing department for August:[LO 6-2, 6-5][LO 6-2, 6-3, 6-4]Work-in-Process Inventory, August 1Conversion: 80% complete 34,000 unitsWork-in-Process Inventory, August 31Conversion: 50% complete 30,000 unitsUnits started 66,000Units completed and transferred out ?CostsWork-in-Process Inventory, August 1Material X $ 64,800Material Y 88,100Conversion 119,880Costs added during AugustMaterial X 152,700Material Y 135,900Conversion 305,120Material X is added at the beginning of work in the mixing department. Material Y is also added in themixing department, but not until units are 60% complete with regard to conversion. Conversion costs areincurred uniformly during the process. The company uses the weighted-average cost method.Required1. Calculate the equivalent units of material X, material Y, and conversion for the mixing department.2.…
arrow_forward
Practice Problem #1
A process consists of 30 activities
E task time = 42 min/unit
Longest activity = 2.4 min
Shortest activity = 0.3 min
Available Time, A = 480 min/day
(A) Max cycle time =
Min cycle time =
YC) If cycle time = 42 min, R =
(D) If cycle time = 2.4 min, R =
(E) If number of workstations, N = 10, and cycle time is 4.2 min/unit
%3D
Output Rate, R =
(F) Assembly-line Efficiency =
arrow_forward
SCA-1.2 - SELF-EMPLOYMENT AND WAGE EMPLOYMENT
Activity time: 20 minutes Instruction to the student:
The students have to discuss and write the advantage of self-employment, comparing it with wage employment.
WAGE / SALARY EMPLOYMENT
SELF EMPLOYMENT/ OWN BUSINESS
arrow_forward
Can we identify a specific area of difficulty being tackled by the workflow management tool?
arrow_forward
Hey can anyone please help me with this question, thanks
arrow_forward
(Question 23) As a process analyst – you are requested to – Map the above project(refer to case study attached) to the Business Process Management lifecycle, briefly explain what you will be doing at each of the following phases.
Please note you are NOT requested to define each phase. Fullmark’s will be allocated when the answers relate to the application of the scenario in the case study.
Process Identification
Phase
Details description of what you will be doing, how it will be done and why.
a)
Process Identification
b) Process discovery
c) Process analysis
d) Process Redesign
e) Process implementation
f) Process monitoring and Controlling
arrow_forward
Chris has been with Drake Manufacturing for five years and was recently promoted to the position of shift supervisor. He is still learning his new position, and it is important to him to be liked and respected. At the most recent operations review meeting, the director reported that the company’s financials are troublesome, and managers should be prepared for a major reorganization. Several jobs will be eliminated, but it had not yet determined which departments would be affected.
Questions
1.Should he give his employees “small doses” of the impending change?
arrow_forward
For tis week I will request from you to solve this mini case.
The CEO of the Baker Inc, wants to implement a new accounting system, to improve work flow through the company’s sales, cash receipts, accounts payable, and cash disbursement procedures. The CEO is, however, concerned about the potential disruption that the implementation will have on operations. In an announcement letter to Baker’s employees, the CEO stated that: “I have contracted Flybynight Consulting Group to do the needs analysis, system selection, and design work. The programming and implementation will be performed in-house using existing IT department staff. The development process will be unobtrusive to user departments because Flybynight knows what needs to be done. They will work independently, in the background, and will not disrupt departmental and internal audit work flow with time consuming interviews, surveys, and questionnaires. This promises to be an efficient process that will produce a system that will…
arrow_forward
Only typed explanation otherwise leave it
arrow_forward
Nine observations from a work measurement study using continuous timing are shown below. Allowances are determined as: personal, five percent; fatigue, five percent; delay, seven percent. Use the Work Measurement Excel template to determine the standard time for this operation.
arrow_forward
Kiko Teddy Bear is a manufacturer of stuffed teddy bears. Kiko would like to be able to produce 40teddy bears per hour on its assembly line. The following information will assist in answering thequestions that follow:
Task Information for Kiko Teddy Bear
Work Element Task Description ImmediatePredecessor Task Time (inseconds)A Cut teddy bear pattern None 90B Sew teddy bear cloth A 75C Stuff teddy bear B 50D Glue on eyes C 20E Glue on nose C…
arrow_forward
Kiko Teddy Bear is a manufacturer of stuffed teddy bears. Kiko would like to be able to produce 40teddy bears per hour on its assembly line. The following information will assist in answering thequestions that follow:
Task Information for Kiko Teddy Bear
Work Element Task Description ImmediatePredecessor Task Time (inseconds)A Cut teddy bear pattern None 90B Sew teddy bear cloth A 75C Stuff teddy bear B 50D Glue on eyes C 20E Glue on nose C…
arrow_forward
I need f and g thank you
arrow_forward
Work measurement is concerned with determining the length of time it should take to complete the job. Job times are vital inputs for capacity planning, workforce planning, estimating labor costs, scheduling, budgeting, and designing incentive systems. Comment.
arrow_forward
International management
arrow_forward
An assembly task was broken down into four elements, and timed using the continuous timing method. The resulting data and performance ratings are provided below. Calculate the total standard time for this task.
Element
1
2
3
4
Watch Time
1.85
2.96
5.42
7.10
Observed Time
Rating
95
105
120
115
Normal Time
Total ST
Standard Time
arrow_forward
Six jobs must be processed through machine A and then machine B as shown below. The processing time for each job is also shown.a. Develop a Gantt chart to determine the total time required to process all six jobs. Use the following sequence of jobs: 1, 2, 3, 4, 5, 6.b. Can you develop a better sequence to reduce the total time required for processing?
arrow_forward
Roger Ginde is developing a program in supply chain management certification for managers. Ginde has listed a number of activities that must be completed before a training program of this nature could be conducted. The activities, immediate predecessors, and times appear in the accompanying table:
Activity
Immediate Predecessor(s)
Time (days)
A
−
2
B
−
6
C
−
1
D
B
10
E
A, D
3
F
C
6
G
E, F
8
This exercise contains only parts b, c, and d.
b) The critical activities for the leadership training program development project are
▼
C - F - G
A - E - G
B - D - E - G
.
c) The project length for the leadership training program development project =
enter your response here
days.
d) Slack time for each of the activities is:
Activity
Slack Time
A
enter your response here
B
enter your response…
arrow_forward
Personnel Planning; TDABC Recent competitive pressures have caused National InsuranceCompany to examine policies regarding personnel planning. As a start, the company has decided toexperiment with and develop a time-driven ABC model for its claims processing center.A study of this support center indicates the following three primary activities: remote processingof customer claims, 0.5 hour; onsite processing of customer claims, 1.0 hour; and updating/maintaining customer records, 0.2 hour. Onsite processing is required for larger claims, while remote processing is done for smaller claims. (For onsite processing, assume—for simplicity—that the claimsprocessors use their own automobiles.) All claims will require that the customers’ records be updated.The claims processing center currently employs three full-time employees. Total annual cost ofthe center (salaries, depreciation, utilities, etc.) is estimated at $255,000. The net amount of availablepersonnel time per year for this…
arrow_forward
Roger Ginde is developing a program in supply chain management certification for managers. Ginde has listed a number of activities that must be completed before a training program of this nature could be conducted. The activities, immediate predecessors, and times appear in the accompanying table:
Activity
Immediate Predecessor(s)
Time (days)
A
−
4
B
−
6
C
−
1
D
B
10
E
A, D
5
F
C
5
G
E, F
8
This exercise contains only parts b, c, and d.
Part 2
b) The critical activities for the leadership training program development project are
▼
B - D - E - G
C - F - G
A - E - G
.
Part 3
c) The project length for the leadership training program development project =
enter your response here
days.
Part 4
d) Slack time for each of the activities is:
Part 5
Part 6
Part 7…
arrow_forward
SEE MORE QUESTIONS
Recommended textbooks for you
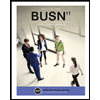
BUSN 11 Introduction to Business Student Edition
Business
ISBN:9781337407137
Author:Kelly
Publisher:Cengage Learning
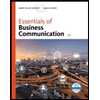
Essentials of Business Communication (MindTap Cou...
Business
ISBN:9781337386494
Author:Mary Ellen Guffey, Dana Loewy
Publisher:Cengage Learning
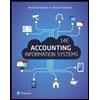
Accounting Information Systems (14th Edition)
Business
ISBN:9780134474021
Author:Marshall B. Romney, Paul J. Steinbart
Publisher:PEARSON
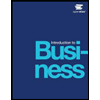
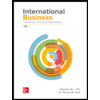
International Business: Competing in the Global M...
Business
ISBN:9781259929441
Author:Charles W. L. Hill Dr, G. Tomas M. Hult
Publisher:McGraw-Hill Education
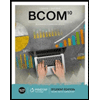
Related Questions
- Student: Angelea Tingey The following table details the tasks required for Indiana-based Frank Pianki Industries to manufacture a fully portable industrial vacuum cleaner. The times in the table are in minutes. Demand forecasts indicate a need to operate with a cycle time of 10 minutes. Performance Activity Activity Description Time (mins) A Attach wheels to tub B Attach motor to lid C Attach battery pack D Attach safety cutoff E Attach filters Attach lid to tub (1) Yes O No F G H I Assemble attachments Function test Final inspection Packing c) The goal is to have 100% efficiency. Is this possible? (1). d) The theoretical minimum number of workstations = number) 5.0 1.8 3.0 3.8 3.0 2.0 3.0 Instructor: Angela Johnson Course: MGT-455-0500 4.0 2.0 2.0 Predecessors B с B A, E a-b) One of the possible assignment of tasks to workstations is shown correctly in Fig. 2 The efficiency of the assembly line with 3 workstations = decimal places) D, F, G H I % (enter your response rounded to two…arrow_forwardYou are alone at home and have to prepare a bread sandwich for yourself. The following preparation activities and time taken are given in the table below: Task Description Predecessor Time (Minutes) A Purchase of bread - 20 B Take cheese and apply on bread A 5 C Get onions from freezer A 1 D Fry onions with pepper B,C 6 E Purchase sauce for bread - 15 F Toast bread B,C 4 G Assemble bread and fried onions F 2 H Arrange in plat G 1 Determine: a. While purchasing sauce, you met a friend and spoke to him for 6 minutes. Did this cause any delay in the preparation?arrow_forwardWeighted-Average Method Arsenio Company manufactures a single product that goes throughtwo processes, mixing and cooking. The following data pertain to the mixing department for August:[LO 6-2, 6-5][LO 6-2, 6-3, 6-4]Work-in-Process Inventory, August 1Conversion: 80% complete 34,000 unitsWork-in-Process Inventory, August 31Conversion: 50% complete 30,000 unitsUnits started 66,000Units completed and transferred out ?CostsWork-in-Process Inventory, August 1Material X $ 64,800Material Y 88,100Conversion 119,880Costs added during AugustMaterial X 152,700Material Y 135,900Conversion 305,120Material X is added at the beginning of work in the mixing department. Material Y is also added in themixing department, but not until units are 60% complete with regard to conversion. Conversion costs areincurred uniformly during the process. The company uses the weighted-average cost method.Required1. Calculate the equivalent units of material X, material Y, and conversion for the mixing department.2.…arrow_forward
- Practice Problem #1 A process consists of 30 activities E task time = 42 min/unit Longest activity = 2.4 min Shortest activity = 0.3 min Available Time, A = 480 min/day (A) Max cycle time = Min cycle time = YC) If cycle time = 42 min, R = (D) If cycle time = 2.4 min, R = (E) If number of workstations, N = 10, and cycle time is 4.2 min/unit %3D Output Rate, R = (F) Assembly-line Efficiency =arrow_forwardSCA-1.2 - SELF-EMPLOYMENT AND WAGE EMPLOYMENT Activity time: 20 minutes Instruction to the student: The students have to discuss and write the advantage of self-employment, comparing it with wage employment. WAGE / SALARY EMPLOYMENT SELF EMPLOYMENT/ OWN BUSINESSarrow_forwardCan we identify a specific area of difficulty being tackled by the workflow management tool?arrow_forward
- Hey can anyone please help me with this question, thanksarrow_forward(Question 23) As a process analyst – you are requested to – Map the above project(refer to case study attached) to the Business Process Management lifecycle, briefly explain what you will be doing at each of the following phases. Please note you are NOT requested to define each phase. Fullmark’s will be allocated when the answers relate to the application of the scenario in the case study. Process Identification Phase Details description of what you will be doing, how it will be done and why. a) Process Identification b) Process discovery c) Process analysis d) Process Redesign e) Process implementation f) Process monitoring and Controllingarrow_forwardChris has been with Drake Manufacturing for five years and was recently promoted to the position of shift supervisor. He is still learning his new position, and it is important to him to be liked and respected. At the most recent operations review meeting, the director reported that the company’s financials are troublesome, and managers should be prepared for a major reorganization. Several jobs will be eliminated, but it had not yet determined which departments would be affected. Questions 1.Should he give his employees “small doses” of the impending change?arrow_forward
- For tis week I will request from you to solve this mini case. The CEO of the Baker Inc, wants to implement a new accounting system, to improve work flow through the company’s sales, cash receipts, accounts payable, and cash disbursement procedures. The CEO is, however, concerned about the potential disruption that the implementation will have on operations. In an announcement letter to Baker’s employees, the CEO stated that: “I have contracted Flybynight Consulting Group to do the needs analysis, system selection, and design work. The programming and implementation will be performed in-house using existing IT department staff. The development process will be unobtrusive to user departments because Flybynight knows what needs to be done. They will work independently, in the background, and will not disrupt departmental and internal audit work flow with time consuming interviews, surveys, and questionnaires. This promises to be an efficient process that will produce a system that will…arrow_forwardOnly typed explanation otherwise leave itarrow_forwardNine observations from a work measurement study using continuous timing are shown below. Allowances are determined as: personal, five percent; fatigue, five percent; delay, seven percent. Use the Work Measurement Excel template to determine the standard time for this operation.arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- BUSN 11 Introduction to Business Student EditionBusinessISBN:9781337407137Author:KellyPublisher:Cengage LearningEssentials of Business Communication (MindTap Cou...BusinessISBN:9781337386494Author:Mary Ellen Guffey, Dana LoewyPublisher:Cengage LearningAccounting Information Systems (14th Edition)BusinessISBN:9780134474021Author:Marshall B. Romney, Paul J. SteinbartPublisher:PEARSON
- International Business: Competing in the Global M...BusinessISBN:9781259929441Author:Charles W. L. Hill Dr, G. Tomas M. HultPublisher:McGraw-Hill Education
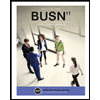
BUSN 11 Introduction to Business Student Edition
Business
ISBN:9781337407137
Author:Kelly
Publisher:Cengage Learning
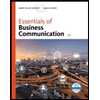
Essentials of Business Communication (MindTap Cou...
Business
ISBN:9781337386494
Author:Mary Ellen Guffey, Dana Loewy
Publisher:Cengage Learning
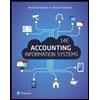
Accounting Information Systems (14th Edition)
Business
ISBN:9780134474021
Author:Marshall B. Romney, Paul J. Steinbart
Publisher:PEARSON
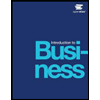
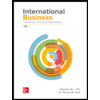
International Business: Competing in the Global M...
Business
ISBN:9781259929441
Author:Charles W. L. Hill Dr, G. Tomas M. Hult
Publisher:McGraw-Hill Education
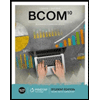