You must write tests for the following: BankAccount Class Constructing a Bank Account with zero Balance. Constructing a Bank Account with Initial Balance greater than zero. Setting AccountID (AccountID should only accept a string of length 4 with first letter as a character followed by 3 integers, Eg., "A001", is a valid AccountID). Getting AccountID. Depositing an amount in Bank. Withdrawing an amount from bank. Getting balance amount. Changes will be required in BankAccount class with respect to: Validation of AccountId - You may write a separate private method for this Given the class public class BankAccount { private String accountID; private double balance; /** Constructs a bank account with a zero balance @param accountID - ID of the Account */ public BankAccount(String accountID) { balance = 0; this.accountID = accountID; } /** Constructs a bank account with a given balance @param initialBalance the initial balance @param accountID - ID of the Account */ public BankAccount(double initialBalance, String accountID) { this.accountID = accountID; balance = initialBalance; } /** * Returns the Account ID * @return the accountID */ public String getAccountID() { return accountID; } /** * Sets the Account ID * @param accountID */ public void setAccountID(String accountID) { this.accountID = accountID; } /** Deposits money into the bank account. @param amount the amount to deposit */ public void deposit(double amount) { balance += amount; } /** Withdraws money from the bank account. @param amount the amount to withdraw @return true if allowed to withdraw */ public boolean withdraw(double amount) { boolean isAllowed = balance >= amount; if (isAllowed) balance -= amount; return isAllowed; } /** Gets the current balance of the bank account. @return the current balance */ public double getBalance() { return balance; }
You must write tests for the following:
- BankAccount Class
- Constructing a Bank Account with zero Balance.
- Constructing a Bank Account with Initial Balance greater than zero.
- Setting AccountID (AccountID should only accept a string of length 4 with first letter as a character followed by 3 integers, Eg., "A001", is a valid AccountID).
- Getting AccountID.
- Depositing an amount in Bank.
- Withdrawing an amount from bank.
- Getting balance amount.
- Changes will be required in BankAccount class with respect to:
- Validation of AccountId - You may write a separate private method for this
Given the class
public class BankAccount {
private String accountID;
private double balance;
/**
Constructs a bank account with a zero balance
@param accountID - ID of the Account
*/
public BankAccount(String accountID)
{
balance = 0;
this.accountID = accountID;
}
/**
Constructs a bank account with a given balance
@param initialBalance the initial balance
@param accountID - ID of the Account
*/
public BankAccount(double initialBalance, String accountID)
{
this.accountID = accountID;
balance = initialBalance;
}
/**
* Returns the Account ID
* @return the accountID
*/
public String getAccountID() {
return accountID;
}
/**
* Sets the Account ID
* @param accountID
*/
public void setAccountID(String accountID) {
this.accountID = accountID;
}
/**
Deposits money into the bank account.
@param amount the amount to deposit
*/
public void deposit(double amount)
{
balance += amount;
}
/**
Withdraws money from the bank account.
@param amount the amount to withdraw
@return true if allowed to withdraw
*/
public boolean withdraw(double amount)
{
boolean isAllowed = balance >= amount;
if (isAllowed)
balance -= amount;
return isAllowed;
}
/**
Gets the current balance of the bank account.
@return the current balance
*/
public double getBalance()
{
return balance;
}

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 6 images

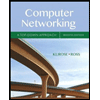
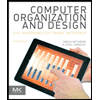
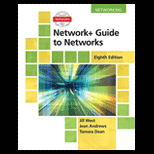
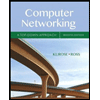
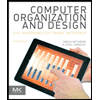
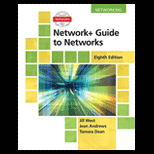
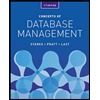
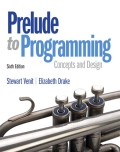
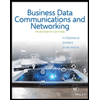