Use the Bisection Method to find the first root of f(x) = -12 – 21x + 18x² – 2.75a. In doing so, use the initial guesses (bracket) of a = – -1 and b = 0 with a stopping criterion of 1% relative error (also known as relative or approximation error), as given by xnew pold Crel = x 100% < a = 1% xnew where xew is the midpoint for the current interval (really, the current estimate of the root) and xold is that for the latest previous iteration. After you have implemented the Bisection Method to find the first root, plot the function f(x) from x = -2 to x = 7 (i.e. these are the x-limits). Using the hold on command, plot your estimate of the root on top of the original plot using a single marker that is of a different style and color than the main plot (for example, plot the range of values in blue with x-markers, and overlay the first root with a red circle marker). This plotting serves as a "sanity check" for the reasonableness of your results. Hints: • You can treat f as an anonymous function so that you can easily pass values of x to f as an argument without having to re-define the expression for f. Example syntax: my_fun = @(x) 2 + 5*x • If you are going to pass a vector of input values to your function f (for example, when plotting) then make sure to use the "dot" operator for element-wise operations (e.g., "*" instead of "*" etc. to avoid non-conformable errors). Explore the documentation for the built-in function plot or the plotting examples from class to beautify your figure.


Following is the Matlab code for the given problem:
%clear the command window
clc;
clear all;
%given function
f=@(x)-12-21*x+18*x^2-275*x^3;
low=-1;
high=0;
tol=0.00000001;
% Evaluate both ends of the interval
y1 = feval(f, low);
y2 = feval(f, high);
i = 0;
% Display error and finish if signs are not different
if y1 * y2 > 0
disp('Have not found a change in sign. Will not continue...');
m = 'Error'
return
end
% Work with the limits modifying them until you find
% a function close enough to zero.
disp('Iter low high x0');
while (abs(high - low) >= tol)
i = i + 1;
% Find a new value to be tested as a root
m = (high + low)/2;
y3 = feval(f, m);
if y3 == 0
fprintf('Root at x = %f \n\n', m);
return
end
fprintf('%2i \t %f \t %f \t %f \n', i-1, low, high, m);
% Update the limits
if y1 * y3 > 0
low = m;
y1 = y3;
else
high = m;
end
end
% Show the last approximation considering the tolerance
w = feval(f, m);
fprintf('\n x = %f produces f(x) = %f \n %i iterations\n', m, y3, i-1);
%plot
hold on;
fplot(f,[-2 7]);
xlabel('x');
ylabel('f(x)');
hS1 =
scatter(m,f(m),300,'*','MarkerEdgeColor',[0 .5 .5],'MarkerFaceColor',[0 .7 .7]);
Code demo for reference:
Step by step
Solved in 5 steps with 4 images

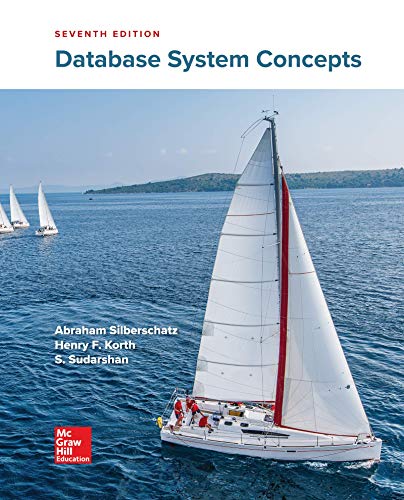
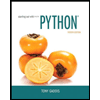
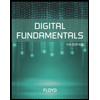
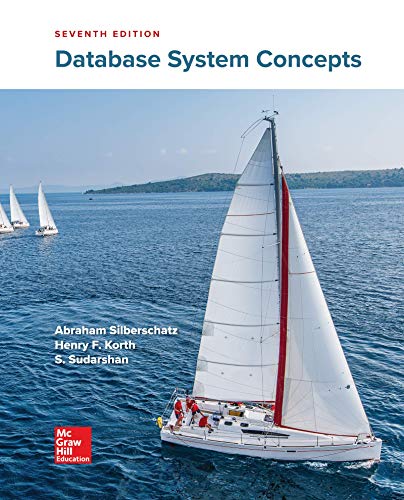
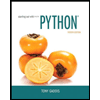
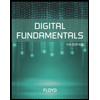
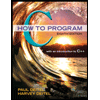
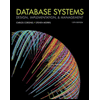
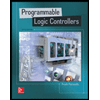