▾ Sample Case 0 Sample Input n 5 x_knots [-2.0, -1.0, 0.0, 1.0, 2.0] y_knots [0.0, 10.0, 15.0, 0.0, 5.0] x_input -0.3 Note: this sample input is formatted as arguments being passed into your function. If you want to write your own test case, it needs to be formatted as text which contains one number per line, in the order of n,x_knots, y_knots and x_input. In this example it will look like the following (for simplicity for all subsequent samples will still use the short format): 5 -2.0 -1.0 0.0 1.0 2.0 0.0 10.0 15.0 0.0 5.0 -0.3 Sample Output 13.5 Explanation x_input -0.3 lies between -1.0 and 0.0. In this interval, LI() is defined by the line segment connecting (-1.0, 10.0) and (0.0, 15.0). LI(-0.3) = 13.5
▾ Sample Case 0 Sample Input n 5 x_knots [-2.0, -1.0, 0.0, 1.0, 2.0] y_knots [0.0, 10.0, 15.0, 0.0, 5.0] x_input -0.3 Note: this sample input is formatted as arguments being passed into your function. If you want to write your own test case, it needs to be formatted as text which contains one number per line, in the order of n,x_knots, y_knots and x_input. In this example it will look like the following (for simplicity for all subsequent samples will still use the short format): 5 -2.0 -1.0 0.0 1.0 2.0 0.0 10.0 15.0 0.0 5.0 -0.3 Sample Output 13.5 Explanation x_input -0.3 lies between -1.0 and 0.0. In this interval, LI() is defined by the line segment connecting (-1.0, 10.0) and (0.0, 15.0). LI(-0.3) = 13.5
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
ANSWER MUST BE IN PYTHON3.
This is the method header:
#!/bin/python3
import math
import os
import random
import re
import sys
#
# Complete the 'linear_interpolate' function below.
#
# The function is expected to return a float.
# The function accepts following parameters:
# 1. int n
# 2. List[float] x_knots
# 3. List[float] y_knots
# 4. float x_input
#
def linear_interpolate(n, x_knots, y_knots, x_input):
![You are asked to implement a linear interpolator. Namely you are given n points on a 2-dimensional coordinate system (known as the knot points). When these points are sorted by their x coordinates and then
connected together using straight lines (in their sorting order), they define a piecewise-linear function LI(x) known as the linear interpolation. When x is outside the range of all knot points, LI(x) is defined by
extrapolation i.e. extending the straight line connecting its two nearest knot points.
You have to complete a function linear_interpolate(n: int, x_knots: List[float], y_knots: List[float], x_input: float) -> float where x_knots and y_knots give you x- and y-coordinate of knot points. Your function
should return LI(x_input). Below is a graph of this calculation for Sample Case 0.
(-2, 0)
(-1, 10)
I
(0, 15)
13.5
-0.3 0
Blue: knot points
Orange:
Linear Interpolation
x_input: -0.3
output: 13.5
(1, 0)
(2,5)
In case multiple knot points share the same x-coordinate x, when x_input <= x, we break the tie by using the smallest y-coordinate among those points. When x_input>x, we break the tie by using the largest y
coordinate among those points.
Further Notes
• 1 <n<= 100,000
• x_knots and y_knots are guaranteed to have the same length
. No guarantee that x_knots and y_knots are pre-sorted in any way
•
For this question we expect an implementation "by hand." Please do not utilize a library which has linear interpolation implemented.
• Floating-points numbers in all our tests have at most three decimal places (i.e. they are multiples of 0.001) so equality between them is unambiguous
▾ Input Format
Your function signature (in Python) should look like: linear_interpolate(n,x_knots, y_knots, x_input). The arguments are:
• n (int): number of knot points
• x_knots (List[float]): x-coordinates of the knot points
• y_knots (List[float]): y-coordinates of the knot points
• x_input (float): input to interpolation i.e. your function should return LI(x_input)
Note: The function signature in other languages will look slightly different due to grammar and naming conventions.](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F6d1ae07f-bdb5-47a8-9afa-ea5a04bdf9c8%2F920ef093-24b2-4f77-9b62-285feab5de8e%2Fwp9choq_processed.jpeg&w=3840&q=75)
Transcribed Image Text:You are asked to implement a linear interpolator. Namely you are given n points on a 2-dimensional coordinate system (known as the knot points). When these points are sorted by their x coordinates and then
connected together using straight lines (in their sorting order), they define a piecewise-linear function LI(x) known as the linear interpolation. When x is outside the range of all knot points, LI(x) is defined by
extrapolation i.e. extending the straight line connecting its two nearest knot points.
You have to complete a function linear_interpolate(n: int, x_knots: List[float], y_knots: List[float], x_input: float) -> float where x_knots and y_knots give you x- and y-coordinate of knot points. Your function
should return LI(x_input). Below is a graph of this calculation for Sample Case 0.
(-2, 0)
(-1, 10)
I
(0, 15)
13.5
-0.3 0
Blue: knot points
Orange:
Linear Interpolation
x_input: -0.3
output: 13.5
(1, 0)
(2,5)
In case multiple knot points share the same x-coordinate x, when x_input <= x, we break the tie by using the smallest y-coordinate among those points. When x_input>x, we break the tie by using the largest y
coordinate among those points.
Further Notes
• 1 <n<= 100,000
• x_knots and y_knots are guaranteed to have the same length
. No guarantee that x_knots and y_knots are pre-sorted in any way
•
For this question we expect an implementation "by hand." Please do not utilize a library which has linear interpolation implemented.
• Floating-points numbers in all our tests have at most three decimal places (i.e. they are multiples of 0.001) so equality between them is unambiguous
▾ Input Format
Your function signature (in Python) should look like: linear_interpolate(n,x_knots, y_knots, x_input). The arguments are:
• n (int): number of knot points
• x_knots (List[float]): x-coordinates of the knot points
• y_knots (List[float]): y-coordinates of the knot points
• x_input (float): input to interpolation i.e. your function should return LI(x_input)
Note: The function signature in other languages will look slightly different due to grammar and naming conventions.
![▾ Sample Case 0
Sample Input
n 5
x_knots = [-2.0, -1.0, 0.0, 1.0, 2.0]
y_knots [0.0, 10.0, 15.0, 0.0, 5.0]
x_input -0.3
Note: this sample input is formatted as arguments being passed into your function. If you want to write your own test case, it needs to be formatted as text which contains one number per line, in the order of
n,x_knots, y_knots and x_input. In this example it will look like the following (for simplicity for all subsequent samples will still use the short format):
5
-2.0
-1.0
0.0
1.0
2.0
0.0
10.0
15.0
0.0
5.0
-0.3
Sample Output
13.5
Explanation
x_input-0.3 lies between -1.0 and 0.0. In this interval, LI() is defined by the line segment connecting (-1.0, 10.0) and (0.0, 15.0). LI(-0.3) = 13.5](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F6d1ae07f-bdb5-47a8-9afa-ea5a04bdf9c8%2F920ef093-24b2-4f77-9b62-285feab5de8e%2Fky57u4_processed.jpeg&w=3840&q=75)
Transcribed Image Text:▾ Sample Case 0
Sample Input
n 5
x_knots = [-2.0, -1.0, 0.0, 1.0, 2.0]
y_knots [0.0, 10.0, 15.0, 0.0, 5.0]
x_input -0.3
Note: this sample input is formatted as arguments being passed into your function. If you want to write your own test case, it needs to be formatted as text which contains one number per line, in the order of
n,x_knots, y_knots and x_input. In this example it will look like the following (for simplicity for all subsequent samples will still use the short format):
5
-2.0
-1.0
0.0
1.0
2.0
0.0
10.0
15.0
0.0
5.0
-0.3
Sample Output
13.5
Explanation
x_input-0.3 lies between -1.0 and 0.0. In this interval, LI() is defined by the line segment connecting (-1.0, 10.0) and (0.0, 15.0). LI(-0.3) = 13.5
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 2 steps with 2 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
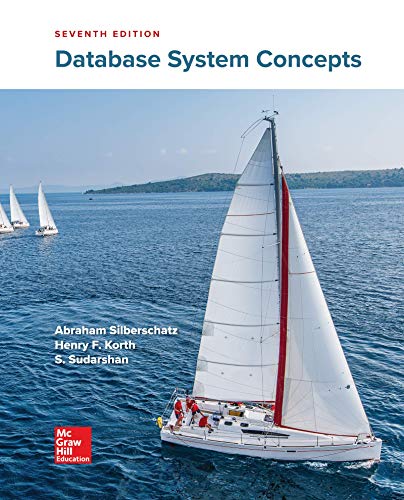
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
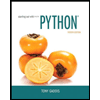
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
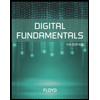
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
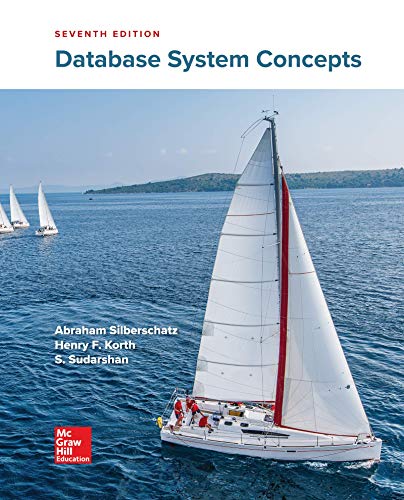
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
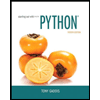
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
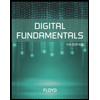
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
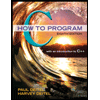
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
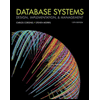
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
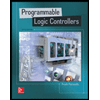
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education