Problem 1 In this problem, we want to sort an input array. Problem 1A (easier) In this problem, develop an algorithm that, given an unsorted array, creates a new array which is the sorted version of the given array. Here is the main idea of the algorithm: first scan through the original input array, finding the smallest element, and then insert this element into the beginning (index 0) of the new array, and remove this smallest element from the input array. Next, scan the (shortened) input array again, finding the smallest element, and then insert it into the next available position of the new array and remove it from the input array. Repeat the process until the original array becomes size 0 (also the new array contains all the values in the original input array). Develop flowchart for this algorithm, Hint: you will need nested loops for doing the work in one main algorithm. You can also write a sub- program to do some work. In this case the main algorithm has one loop. Hint: to find the smallest element in an array, define a variable, say, minIndex, which is initially 0, then if an element at index k of the array is smaller than the element at index minIndex, update minIndex to be k. For removing an element at index k from array arr, you can just say "remove element at index k from arr", or "remove arr[k] from arr" Start your flowchart as shown in the following picture, which first get an input array arr, and then create new array newArr. Save the flowchart as img_01A.jpg start arr[] newArr []
Problem 1 In this problem, we want to sort an input array. Problem 1A (easier) In this problem, develop an algorithm that, given an unsorted array, creates a new array which is the sorted version of the given array. Here is the main idea of the algorithm: first scan through the original input array, finding the smallest element, and then insert this element into the beginning (index 0) of the new array, and remove this smallest element from the input array. Next, scan the (shortened) input array again, finding the smallest element, and then insert it into the next available position of the new array and remove it from the input array. Repeat the process until the original array becomes size 0 (also the new array contains all the values in the original input array). Develop flowchart for this algorithm, Hint: you will need nested loops for doing the work in one main algorithm. You can also write a sub- program to do some work. In this case the main algorithm has one loop. Hint: to find the smallest element in an array, define a variable, say, minIndex, which is initially 0, then if an element at index k of the array is smaller than the element at index minIndex, update minIndex to be k. For removing an element at index k from array arr, you can just say "remove element at index k from arr", or "remove arr[k] from arr" Start your flowchart as shown in the following picture, which first get an input array arr, and then create new array newArr. Save the flowchart as img_01A.jpg start arr[] newArr []
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
100%
![Problem 1
In this problem, we want to sort an input array.
Problem 1A (easier)
In this problem, develop an algorithm that, given an unsorted array, creates a new array which is the sorted
version of the given array.
Here is the main idea of the algorithm: first scan through the original input array, finding the smallest element, and
then insert this element into the beginning (index 0) of the new array, and remove this smallest element from the
input array. Next, scan the (shortened) input array again, finding the smallest element, and then insert it into the next
available position of the new array and remove it from the input array. Repeat the process until the original array
becomes size 0 (also the new array contains all the values in the original input array).
Develop flowchart for this algorithm,
Hint: you will need nested loops for doing the work in one main algorithm. You can also write a sub-
program to do some work. In this case the main algorithm has one loop.
Hint: to find the smallest element in an array, define a variable, say, minIndex, which is initially 0,
then if an element at index k of the array is smaller than the element at index minIndex, update
minIndex to be k.
For removing an element at index k from array arr, you can just say "remove element at indexk from
arr", or "remove arr[k] from arr"
Start your flowchart as shown in the following picture, which first get an input array arr, and then
create new array newArr.
Save the flowchart as img_01A.jpg
start
arr[]
newArr []](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fdac761a6-ba1c-4b50-93cd-f82ea0746880%2F65d19518-06fe-4383-a024-5c156847b1af%2F8gnk919_processed.jpeg&w=3840&q=75)
Transcribed Image Text:Problem 1
In this problem, we want to sort an input array.
Problem 1A (easier)
In this problem, develop an algorithm that, given an unsorted array, creates a new array which is the sorted
version of the given array.
Here is the main idea of the algorithm: first scan through the original input array, finding the smallest element, and
then insert this element into the beginning (index 0) of the new array, and remove this smallest element from the
input array. Next, scan the (shortened) input array again, finding the smallest element, and then insert it into the next
available position of the new array and remove it from the input array. Repeat the process until the original array
becomes size 0 (also the new array contains all the values in the original input array).
Develop flowchart for this algorithm,
Hint: you will need nested loops for doing the work in one main algorithm. You can also write a sub-
program to do some work. In this case the main algorithm has one loop.
Hint: to find the smallest element in an array, define a variable, say, minIndex, which is initially 0,
then if an element at index k of the array is smaller than the element at index minIndex, update
minIndex to be k.
For removing an element at index k from array arr, you can just say "remove element at indexk from
arr", or "remove arr[k] from arr"
Start your flowchart as shown in the following picture, which first get an input array arr, and then
create new array newArr.
Save the flowchart as img_01A.jpg
start
arr[]
newArr []
![Implement the algorithm using JavaScript
Complete function problem1A() in JS file, and attach the onclick event to the corresponding button m
html. This function first generates a random array, and at the end output both the original and tune
sorted arrays.
Your implementation should match your flowchart. E.g., if your flowchart contains only one main
algorithm, then
flowchart, then you should implement a sub-function.
you should implement the whole algorithm in the function. If you use sub-algorithm in
For removing an element from array, turn to w3school for help. Hint, function splice() is one of the
choices. To use this, you need to keep track of the index of the element to be removed.
Sample output (each run will generate a different input array)
2,82,62,59, 30,52,56,40,25,93
2,25,30,40,52,56,59,62,82,93
problem1A-prray sort
problem18 -- array sort [in-place]
problem8-- array search
problem3A- string compare [same length]
problem38 string compare](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fdac761a6-ba1c-4b50-93cd-f82ea0746880%2F65d19518-06fe-4383-a024-5c156847b1af%2Fp5f60v_processed.jpeg&w=3840&q=75)
Transcribed Image Text:Implement the algorithm using JavaScript
Complete function problem1A() in JS file, and attach the onclick event to the corresponding button m
html. This function first generates a random array, and at the end output both the original and tune
sorted arrays.
Your implementation should match your flowchart. E.g., if your flowchart contains only one main
algorithm, then
flowchart, then you should implement a sub-function.
you should implement the whole algorithm in the function. If you use sub-algorithm in
For removing an element from array, turn to w3school for help. Hint, function splice() is one of the
choices. To use this, you need to keep track of the index of the element to be removed.
Sample output (each run will generate a different input array)
2,82,62,59, 30,52,56,40,25,93
2,25,30,40,52,56,59,62,82,93
problem1A-prray sort
problem18 -- array sort [in-place]
problem8-- array search
problem3A- string compare [same length]
problem38 string compare
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 2 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
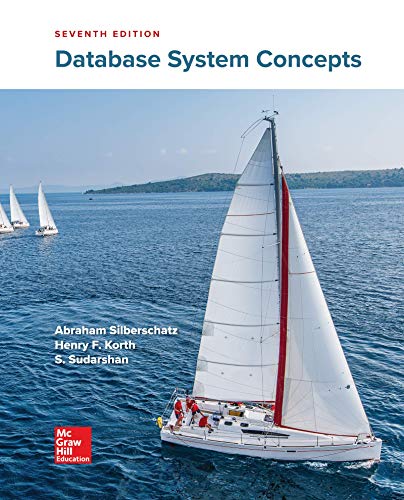
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
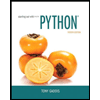
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
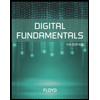
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
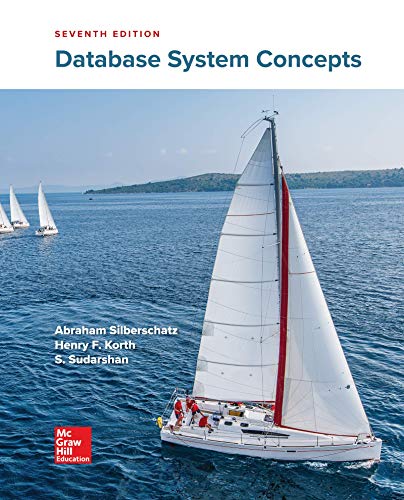
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
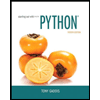
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
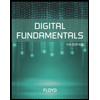
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
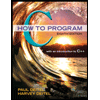
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
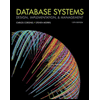
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
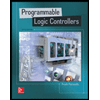
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education