Month Class Exceptions Write a class named Month. The class should have an int field named monthNumber that holds the number of the month. For example, Janauary would be 1, February would be 2, and so forth. In addition, provide the following methods: A no-arg constructor that sets the monthNumber field to 1. A constructor that accepts the number of the month as an argument. It should set the monthNumber value to the value passed as the argument. If a value less than 1 or greater than 12 is passed, the constructor should throw an InvalidMonthNumberException. A constructor that accepts the name of the month, such as “January” or “February”, as an argument. It should set the monthNumber field to the correct corresponding value. If an invalid name is passed, the constructor should throw an InvalidMonthNameException. A setMonthNumber method that accepts an int argument, which is assigned to the monthNumber field. If a value less than 1 or greater than 12 is passed, throw an InvalidMonthNumberException. A getMonthNumber method that returns the value in the monthNumber field. A getMonthName method that returns the name of the month. For example, if the monthNumber field contains 1, then this method should return “January”. A toString method that returns the same value as the getMonthName method. An equals method that accepts a Month object as an argument. If the argument object holds the same data as the calling object, this method should return true. Otherwise, it should return false. A greaterThan method that accepts a Month object as an argument. If the calling object’s monthNumber field is greater than the argument’s monthNumber field, this method should return true. Otherwise, it should return false. A lessThan method that accepts a Month object as an argument. If the calling object’s monthNumber field is less than the argument’s monthNumber field, this method should return true. Otherwise, it should return false.
OOPs
In today's technology-driven world, computer programming skills are in high demand. The object-oriented programming (OOP) approach is very much useful while designing and maintaining software programs. Object-oriented programming (OOP) is a basic programming paradigm that almost every developer has used at some stage in their career.
Constructor
The easiest way to think of a constructor in object-oriented programming (OOP) languages is:
Month Class Exceptions
Write a class named Month. The class should have an int field named monthNumber that holds the number of the month. For example, Janauary would be 1, February would be 2, and so forth.
In addition, provide the following methods:
- A no-arg constructor that sets the monthNumber field to 1.
- A constructor that accepts the number of the month as an argument. It should set the monthNumber value to the value passed as the argument. If a value less than 1 or greater than 12 is passed, the constructor should throw an InvalidMonthNumberException.
- A constructor that accepts the name of the month, such as “January” or “February”, as an argument. It should set the monthNumber field to the correct corresponding value. If an invalid name is passed, the constructor should throw an InvalidMonthNameException.
- A setMonthNumber method that accepts an int argument, which is assigned to the monthNumber field. If a value less than 1 or greater than 12 is passed, throw an InvalidMonthNumberException.
- A getMonthNumber method that returns the value in the monthNumber field.
- A getMonthName method that returns the name of the month. For example, if the monthNumber field contains 1, then this method should return “January”.
- A toString method that returns the same value as the getMonthName method.
- An equals method that accepts a Month object as an argument. If the argument object holds the same data as the calling object, this method should return true. Otherwise, it should return false.
- A greaterThan method that accepts a Month object as an argument. If the calling object’s monthNumber field is greater than the argument’s monthNumber field, this method should return true. Otherwise, it should return false.
- A lessThan method that accepts a Month object as an argument. If the calling object’s monthNumber field is less than the argument’s monthNumber field, this method should return true. Otherwise, it should return false.



Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

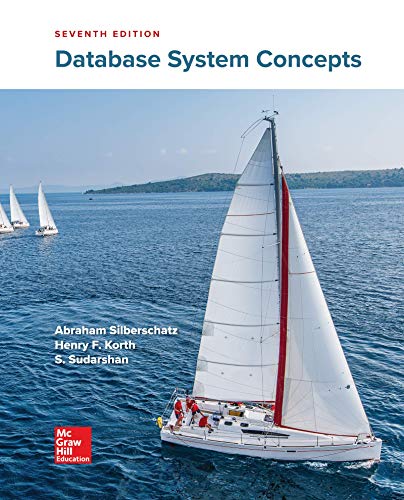
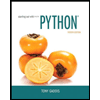
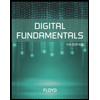
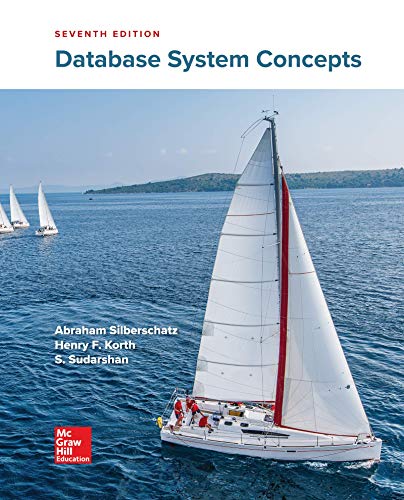
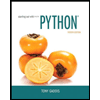
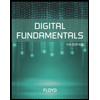
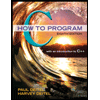
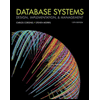
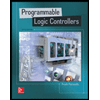