Indicate where the different constructors, assignment operator- and desctructor will run with a comment under the relevant line of code. HINT: run the program but make modifications that help see what is going on or step through using https://onlinegdb.com. Start in main and use the following tags to indicate the function that will run:
Indicate where the different constructors, assignment operator- and desctructor will run with a comment under the relevant line of code. HINT: run the program but make modifications that help see what is going on or step through using https://onlinegdb.com. Start in main and use the following tags to indicate the function that will run:
Programming with Microsoft Visual Basic 2017
8th Edition
ISBN:9781337102124
Author:Diane Zak
Publisher:Diane Zak
Chapter6: Sub And Function Procedures
Section: Chapter Questions
Problem 2MQ2
Related questions
Question
Need help with C++ Question.
The question is attatched as a image, and the code is copied below:
#include <iostream> | ||
2 | using namespace std; | |
3 | ||
4 | class Student { | |
5 | public: | |
6 | friend Student OutputDuplicate (Student obj); // friend function | |
7 | ||
8 | Student (); // Default constructor | |
9 | ||
10 | Student (string n); // String constructor | |
11 | ||
12 | Student (const Student & obj); // Copy constructor | |
13 | ||
14 | Student& operator= (const Student & right); // Assignment operator | |
15 | ||
16 | ~Student() ; // Destructor | |
17 | ||
18 | private: | |
19 | string name; | |
20 | ||
21 | }; | |
22 | ||
23 | // Default constructor | |
24 | Student::Student() : name("no name") | |
25 | { | |
26 | // Intentionally left blank | |
27 | } | |
28 | ||
29 | // String constructor | |
30 | Student::Student(string n) : name(n) | |
31 | { | |
32 | // Intentionally left blank | |
33 | } | |
34 | ||
35 | // Copy constructor | |
36 | Student::Student(const Student & obj) : name(obj.name) | |
37 | { | |
38 | // Intentionally left blank | |
39 | } | |
40 | ||
41 | // Assignment operator | |
42 | Student& Student::operator= (const Student & right) | |
43 | { | |
44 | name=right.name; | |
45 | return (*this); | |
46 | } | |
47 | ||
48 | // Destructor | |
49 | Student::~Student() | |
50 | { | |
51 | // Intentionally left blank | |
52 | } | |
53 | ||
54 | ////////////////////////////////////////////////////////////////////////////// | |
55 | // MARK UP FROM HERE DOWN | |
56 | ////////////////////////////////////////////////////////////////////////////// | |
57 | ||
58 | // friend function | |
59 | Student OutputDuplicate (Student obj) | |
60 | { | |
61 | Student tmp("Jack"); | |
62 | ||
63 | cout <<"Creating a new student Jame smith\n"; | |
64 | Student * anotherObj = new Student ("Jane Smith"); | |
65 | ||
66 | *anotherObj = obj; | |
67 | return tmp; | |
68 | } | |
69 | ||
70 | int main() | |
71 | { | |
72 | Student a, b ("John Smith"); | |
73 | ||
74 | cout <<"calling OutputDuplicate\n\n"; | |
75 | ||
76 | a = OutputDuplicate (b); | |
77 | ||
78 | cout <<"\nExiting\n"; | |
79 | } |

Transcribed Image Text:Indicate where the different constructors, assignment operator- and desctructor will run with a comment under the relevant line of code. HINT: run the program but make modifications that help see what is going on or step through using https://onlinegdb.com.
Start in main and use the following tags to indicate the function that will run:
Default constructor <object name>
String constructor <object name>
Copy constructor <object name
Assignment operator
Destructor <object name>
Substitute the variable name for the object constructed or destructed. Use a comma to separate if multiple functions are run on a line.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
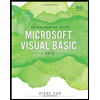
Programming with Microsoft Visual Basic 2017
Computer Science
ISBN:
9781337102124
Author:
Diane Zak
Publisher:
Cengage Learning
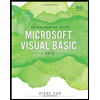
Programming with Microsoft Visual Basic 2017
Computer Science
ISBN:
9781337102124
Author:
Diane Zak
Publisher:
Cengage Learning