In programming, a recursive function calls itself. The classical example is factorial(n), which can be defined recursively as n*factorial(n-1). Nonethessless, it is important to take note that a recursive function should have a terminating condition (or base case), in the case of factorial, factorial(0)=1. Hence, the full definition is: factorial(n) = 1, for n = 0 factorial(n) = n * factorial(n-1), for all n > 1 For example, suppose n = 5: // Recursive call factorial(5) = 5 * factorial(4) factorial(4) = 4 * factorial(3) factorial(3) = 3 * factorial(2) factorial(2) = 2 * factorial(1) factorial(1) = 1 * factorial(0) factorial(0) = 1 // Base case // Unwinding factorial(1) = 1 * 1 = 1 factorial(2) = 2 * 1 = 2 factorial(3) = 3 * 2 = 6 factorial(4) = 4 * 6 = 24 factorial(5) = 5 * 24 = 120 (DONE) Exercise (Factorial) (Recursive): Write a recursive method called factorial() to compute the factorial of the given integer. public static int factorial(int n) The recursive algorithm is: factorial(n) = 1, if n = 0 factorial(n) = n * factorial(n-1), if n > 0 Compare your code with the iteractive version of the factorial(): factorial(n) = 1*2*3*...*n Hints: // Return the factorial of the given integer, recursively public static int factorial(int n) { if (n == 0) { return 1; // base case } else { return n * factorial(n-1); // call itself } // or one liner // return (n == 0) ? 1 : n*factorial(n-1); } Notes: Recursive version is often much shorter. The recursive version uses much more computation and storage resources, and it need to save its states before each successive recursive call. Exercise (Fibonacci) (Recursive): Write a recursive method to compute the Fibonacci sequence of n, defined as follows: F(0) = 0 F(1) = 1 F(n) = F(n-1) + F(n-2) for n >= 2 Compare the recursive version with the iteractive version written earlier. Exercise (A Running Number Sequence) (Recursive): A special number sequence is defined as follows: S(1) = 1 S(2) = 12 S(3) = 123 S(4) = 1234 ...... S(9) = 123456789 // length is 9 S(10) = 12345678910 // length is 11 S(11) = 1234567891011 // length is 13 ...... Write a recursive method to compute the length of S(n). Also write an iteractive version. Exercise (GCD) (Recursive): Write a recursive method called gcd() to compute the greatest common divisor of two given integers. public static void int gcd(int a, int b) gcd(a,b) = a, if b = 0 gcd(a,b) = gcd(b, remainder(a,b)), if b > 0 Exercise (Tower of Hanoi Recursive) (Recursive): [TODO] Exercises on Algorithms - Sorting and Searching Efficient sorting and searching are big topics, typically covered in a course called "Data Structures and Algorithms". There are many searching and sorting algorithms available, with their respective strengths and weaknesses. See Wikipedia "Sorting Algorithms" and "Searching Algorithms" for the algorithms, examples and illustrations. JDK provides searching and sorting utilities in the Arrays class (in package java.util), such as Arrays.sort() and Arrays.binarySearch() - you don't have to write your searching and sorting in your production program. These exercises are for academic purpose and for you to gain some understandings and practices on these algorithms. Exercise (Linear Search): (Reference: Wikipedia "Linear Search") Compare each item with the search key in the linear manner. Linear search is applicable to unsorted list. // Return the array index, if key is found; or 0 otherwise public static int linearSearch(int[] array, int key) // or // Return true if the key is found public static boolean linearSearch(int[] array, int key) Exercise (Selection Sort): (Reference: Wikipedia "Selection Sort") This algorithm divides the lists into two parts: the left-sublist of items already sorted, and the right-sublist for the remaining items. Initially, the left-sorted-sublist is empty, while the right-unsorted-sublist is the entire list. The algorithm proceeds by finding the smallest (or largest) items from the right-unsorted-sublist, swapping it with the leftmost element of the right-unsorted-sublist, and increase the left-sorted-sublist by one. For example, given the list {9 6 4 1 5}: {} {9 6 4 1 5} -> {} {1 6 4 9 5} {1} {6 4 9 5} -> {1} {4 6 9 5} {1 4} {6 9 5} -> {1 4} {5 9 6} {1 4 5} {9 6} -> {1 4 5} {6 9} {1 4 5 6} {9} -> DONE {1 4 5 6 9} Write a method to sort an int array (in place) with the following signature: public static void selectionSort(int[] array)
In
factorial(n) = 1, for n = 0
factorial(n) = n * factorial(n-1), for all n > 1
For example, suppose n = 5:
// Recursive call
factorial(5) = 5 * factorial(4)
factorial(4) = 4 * factorial(3)
factorial(3) = 3 * factorial(2)
factorial(2) = 2 * factorial(1)
factorial(1) = 1 * factorial(0)
factorial(0) = 1 // Base case
// Unwinding
factorial(1) = 1 * 1 = 1
factorial(2) = 2 * 1 = 2
factorial(3) = 3 * 2 = 6
factorial(4) = 4 * 6 = 24
factorial(5) = 5 * 24 = 120 (DONE)
Exercise (Factorial) (Recursive): Write a recursive method called factorial() to compute the factorial of the given integer.
public static int factorial(int n)
The recursive
factorial(n) = 1, if n = 0
factorial(n) = n * factorial(n-1), if n > 0
Compare your code with the iteractive version of the factorial():
factorial(n) = 1*2*3*...*n
Hints:
// Return the factorial of the given integer, recursively
public static int factorial(int n) {
if (n == 0) {
return 1; // base case
} else {
return n * factorial(n-1); // call itself
}
// or one liner
// return (n == 0) ? 1 : n*factorial(n-1);
}
Notes:
- Recursive version is often much shorter.
- The recursive version uses much more computation and storage resources, and it need to save its states before each successive recursive call.
Exercise (Fibonacci) (Recursive): Write a recursive method to compute the Fibonacci sequence of n, defined as follows:
F(0) = 0
F(1) = 1
F(n) = F(n-1) + F(n-2) for n >= 2
Compare the recursive version with the iteractive version written earlier.
Exercise (A Running Number Sequence) (Recursive): A special number sequence is defined as follows:
S(1) = 1
S(2) = 12
S(3) = 123
S(4) = 1234
......
S(9) = 123456789 // length is 9
S(10) = 12345678910 // length is 11
S(11) = 1234567891011 // length is 13
......
Write a recursive method to compute the length of S(n). Also write an iteractive version.
Exercise (GCD) (Recursive): Write a recursive method called gcd() to compute the greatest common divisor of two given integers.
public static void int gcd(int a, int b)
gcd(a,b) = a, if b = 0
gcd(a,b) = gcd(b, remainder(a,b)), if b > 0
Exercise (Tower of Hanoi Recursive) (Recursive): [TODO]
- Exercises on Algorithms - Sorting and Searching
Efficient sorting and searching are big topics, typically covered in a course called "Data Structures and Algorithms". There are many searching and sorting algorithms available, with their respective strengths and weaknesses. See Wikipedia "Sorting Algorithms" and "Searching Algorithms" for the algorithms, examples and illustrations.
JDK provides searching and sorting utilities in the Arrays class (in package java.util), such as Arrays.sort() and Arrays.binarySearch() - you don't have to write your searching and sorting in your production program. These exercises are for academic purpose and for you to gain some understandings and practices on these algorithms.
Exercise (Linear Search): (Reference: Wikipedia "Linear Search") Compare each item with the search key in the linear manner. Linear search is applicable to unsorted list.
// Return the array index, if key is found; or 0 otherwise
public static int linearSearch(int[] array, int key)
// or
// Return true if the key is found
public static boolean linearSearch(int[] array, int key)
Exercise (Selection Sort): (Reference: Wikipedia "Selection Sort") This algorithm divides the lists into two parts: the left-sublist of items already sorted, and the right-sublist for the remaining items. Initially, the left-sorted-sublist is empty, while the right-unsorted-sublist is the entire list. The algorithm proceeds by finding the smallest (or largest) items from the right-unsorted-sublist, swapping it with the leftmost element of the right-unsorted-sublist, and increase the left-sorted-sublist by one.
For example, given the list {9 6 4 1 5}:
{} {9 6 4 1 5} -> {} {1 6 4 9 5}
{1} {6 4 9 5} -> {1} {4 6 9 5}
{1 4} {6 9 5} -> {1 4} {5 9 6}
{1 4 5} {9 6} -> {1 4 5} {6 9}
{1 4 5 6} {9} -> DONE
{1 4 5 6 9}
Write a method to sort an int array (in place) with the following signature:
public static void selectionSort(int[] array)

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 2 images

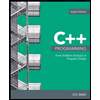
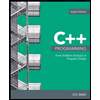