***I am posting this for the second time, because last time my issue was not resolved. There was no update in the code and it gave the same output as before. So please make sure to actually make corrections in the code I provided.*** The output of the following problem should be: P1 -> P3 -> P4 -> P5 -> P0 -> P2 but the output I get when I run my code is: P1 -> P3 -> P4 -> P5 Note: I am sharing my code below. Please tell me what to fix in my code to get the desired output. P Problem: Write a c program that will generate the safe sequence of process execution for the situation given below:(Use Banker’s Algorithm). Note: The code can be implemented in several different ways, but make sure the parameter remains the same as shown below. n = 6; // Number of processes m = 4; // Number of resources int alloc[6][4] = { { 0, 1, 0, 3 }, // P0// Allocation Matrix { 2, 0, 0, 3 }, // P1 { 3, 0, 2, 0 }, // P2 { 2, 1, 1, 5 }, // P3 { 0, 0, 2, 2 }, // P4 {1, 2 , 3, 1 } }; //P5 int max[6][4] = { { 6, 4, 3, 4 }, // P0 // MAX Matrix { 3, 2, 2, 4 }, // P1 { 9, 1, 2, 6 }, // P2 { 2, 2, 2, 8 }, // P3 { 4, 3, 3, 7 }, // P4 { 6, 2, 6, 5 } }; //P5 int avail[4] = { 2, 2, 2, 1 }; //Available resources My code: #include int main() { int n, m; n = 6; // Number of processes m = 4; // Number of resources // Allocation Matrix int allocation[6][4] = { { 0, 1, 0, 3 }, // P0 { 2, 0, 0, 3 }, // P1 { 3, 0, 2, 0 }, // P2 { 2, 1, 1, 5 }, // P3 { 0, 0, 2, 2 }, // P4 {1, 2 , 3, 1 } }; //P5 // MAX Matrix int max[6][4] = { { 6, 4, 3, 4 }, // P0 { 3, 2, 2, 4 }, // P1 { 9, 1, 2, 6 }, // P2 { 2, 2, 2, 8 }, // P3 { 4, 3, 3, 7 }, // P4 { 6, 2 , 6, 5 } }; //P5 //Available resources int avail[4] = { 2, 2, 2, 1 }; //calculating Need Matrix int need[n][m]; for(int i = 0; i < n; i++){ for(int j = 0; j < m; j++) need[i][j] = max[i][j]-allocation[i][j]; } //Banker's Algorithm int k, flag; k = 0; //the variable k is the index of the safe sequence array int completion[20], safe_sequence[20]; /* The completion array stores the processes' completion status. It will store value 0 for an incomplete process and 1 for a complete process */ // Initally all processes are incomplete for(int i = 0; i < n; i++) { completion[i] = 0; } for(int i = 0; i < n; i++) { flag = 0; if(completion[i] == 0) { //Process is incomplete. So execute the Process for(int j = 0; j < m; j++) { if(need[i][j] > avail[j]) //If need is greater than available break out of the loop { flag = 1; break; } } if(flag==0) // Need is less than available { completion[i] = 1; safe_sequence[k] = i; k++; //Calculate new available for(int j = 0; j < m; j++) { avail[j] += allocation[i][j]; } i = i - 1; //loop from the first process } } } printf("Safe Sequence:\n"); for(int i = 0; i < k; i++) { printf("P%d", safe_sequence[i]); if(i < k-1) { printf(" -> "); } } return 0; }
***I am posting this for the second time, because last time my issue was not resolved. There was no update in the code and it gave the same output as before. So please make sure to actually make corrections in the code I provided.***
The output of the following problem should be: P1 -> P3 -> P4 -> P5 -> P0 -> P2
but the output I get when I run my code is: P1 -> P3 -> P4 -> P5
Note: I am sharing my code below. Please tell me what to fix in my code to get the desired output. P
Problem:
Write a c program that will generate the safe sequence of process execution for the situation
given below:(Use Banker’s
Note: The code can be implemented in several different ways, but make sure the parameter
remains the same as shown below.
n = 6; // Number of processes
m = 4; // Number of resources
int alloc[6][4] = { { 0, 1, 0, 3 }, // P0// Allocation Matrix
{ 2, 0, 0, 3 }, // P1
{ 3, 0, 2, 0 }, // P2
{ 2, 1, 1, 5 }, // P3
{ 0, 0, 2, 2 }, // P4
{1, 2 , 3, 1 } }; //P5
int max[6][4] = { { 6, 4, 3, 4 }, // P0 // MAX Matrix
{ 3, 2, 2, 4 }, // P1
{ 9, 1, 2, 6 }, // P2
{ 2, 2, 2, 8 }, // P3
{ 4, 3, 3, 7 }, // P4
{ 6, 2, 6, 5 } }; //P5
int avail[4] = { 2, 2, 2, 1 }; //Available resources
My code:
#include <stdio.h>
int main() {
int n, m;
n = 6; // Number of processes
m = 4; // Number of resources
// Allocation Matrix
int allocation[6][4] = { { 0, 1, 0, 3 }, // P0
{ 2, 0, 0, 3 }, // P1
{ 3, 0, 2, 0 }, // P2
{ 2, 1, 1, 5 }, // P3
{ 0, 0, 2, 2 }, // P4
{1, 2 , 3, 1 } }; //P5
// MAX Matrix
int max[6][4] = { { 6, 4, 3, 4 }, // P0
{ 3, 2, 2, 4 }, // P1
{ 9, 1, 2, 6 }, // P2
{ 2, 2, 2, 8 }, // P3
{ 4, 3, 3, 7 }, // P4
{ 6, 2 , 6, 5 } }; //P5
//Available resources
int avail[4] = { 2, 2, 2, 1 };
//calculating Need Matrix
int need[n][m];
for(int i = 0; i < n; i++){
for(int j = 0; j < m; j++)
need[i][j] = max[i][j]-allocation[i][j];
}
//Banker's Algorithm
int k, flag;
k = 0; //the variable k is the index of the safe sequence array
int completion[20], safe_sequence[20]; /* The completion array stores the processes' completion status.
It will store value 0 for an incomplete process and 1 for a
complete process */
// Initally all processes are incomplete
for(int i = 0; i < n; i++) {
completion[i] = 0;
}
for(int i = 0; i < n; i++) {
flag = 0;
if(completion[i] == 0)
{
//Process is incomplete. So execute the Process
for(int j = 0; j < m; j++) {
if(need[i][j] > avail[j]) //If need is greater than available break out of the loop
{
flag = 1;
break;
}
}
if(flag==0) // Need is less than available
{
completion[i] = 1;
safe_sequence[k] = i;
k++;
//Calculate new available
for(int j = 0; j < m; j++)
{
avail[j] += allocation[i][j];
}
i = i - 1; //loop from the first process
}
}
}
printf("Safe Sequence:\n");
for(int i = 0; i < k; i++)
{
printf("P%d", safe_sequence[i]);
if(i < k-1)
{
printf(" -> ");
}
}
return 0;
}

Step by step
Solved in 3 steps with 1 images

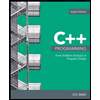
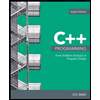