Guide to working through the project Steps: Write the GradeBook constructor that reads student information from the data file into the ArrayList roster). For now, ignore the grades. Test your GradeBook class before proceeding to the next step. Create the Grade class. Test your Grade class before proceeding to the next step. Modify the Student class to include an ArrayList of Grade. Test the Student class before proceeding to the next step. Finish writing the GradeBook constructor to process the grades as they are read in from the data file. Test the GradeBook class before proceeding to the next step. Add additional functionality to the GradeBook and Student classes one method at a time. Here is Grade.jave code is shown below. public class Grade { // TODO: complete this class as described in this task write-up } GradeBook Class The GradeBook class has a single instance variable roster, an ArrayList of Student. This class performs all operations related to the scores of all students in the data file. Starter code for the GradeBook class is provided. GradeBook(String fileName) This constructor needs to be modified to work with the given file format. As a first step, read the student information from the data file, but ignore the grades for now. You will want to use the provided getFileScanner method, and for looping through the data, you will want to mimic the loop in the EmployeeManager constructor in L07-Employees Download L07-Employees. Note that here we are adding a student to the instance variable roster. Once you have this part of the constructor finished, you should be able to run your code and see the output of a long list of student information (without grades). After creating the Grade class, you can return to working on this constructor to incorporate the reading of the grades. A suggestion would be that while reading the file a line at a time and parsing each line into tokens (such as first name, etc), create a loop over the grade-name/grade-value pairs. You may assume that the file is well-formatted, i.e., each line contains a first name, last name, age, major, username, and a number of grade-name/grade-value pairs. For each grade-name/grade-value pair, create a Grade, and then call the addGrade method you just wrote in the Student class. In summary, for each line of the file, you create a Student with first name, last name, age, major, and username, and then call the addGrade method in the Student class to add each grade to the Student object. Grade Class The Grade class will represent and store information about a single grade. A grade has two components: a name (stored as a String, e.g., "HW1"), and the score for that assignment (stored as an int). You will need to write a constructor for this class as well as a few methods. In particular, you will need instance methods to get a grade's name, get a grade's value, and change a grade value. The constructor of the Grade class will get the grade name and score as parameters. Instance methods in the Grade class In addition to the constructor, you will need to write the following instance methods in the Grade class. The required methods must be public. Test all your methods by calling them from main. String getName()Accessor method that returns the name component of a Grade.int getScore()Accessor method that returns the score component of a Grade.String toString()Returns a String representation of a Grade, eg, "HW1 23".void changeGrade(int score)Change the score of an individual Grade. Student Class The Student class will represent and store information about a single student. This class performs all operations related to a single student, such as constructing a Student and giving accessor/mutator methods. Starter code for the Student class is provided which stores the first name, last name, age, major, and username. After completing Steps 1 and 2, you will modify the Student class to include an ArrayList of grades, and will add instance methods to support the operations of the GradeBook class. Make the following modifications: (a) add a private instance variable grades that is an ArrayList of type Grade; (b) modify the constructors to initialize grades to a new ArrayList of type Grade [just one line to add]; (c) implement a new instance method addGrade() [just one line]; (d) complete method definitions for the other required instance methods listed below; (e) modify the Student.toString() method so the return statement becomes return super.toString() + " " + username + " " + major + " " + gradesString(); (f) add code to the main method in the Student class to test the changes. Printing result of call to student.getNameAndMajor(): Grace Hopper CSCI Printing student after adding a grade with student.addGrade(new Grade("HW1", 95)): Grace Hopper 20 ghopper CSCI HW1 95 Printing individual grades for username "pahmad": Q1 80, HW1 80, Q2 90, HW2 80, Q3 89, HW3 90, Q4 94, HW4 70, Q5 88, HW5 92, EXAM 89
Guide to working through the project
Steps:
- Write the GradeBook constructor that reads student information from the data file into the ArrayList roster). For now, ignore the grades. Test your GradeBook class before proceeding to the next step.
- Create the Grade class. Test your Grade class before proceeding to the next step.
- Modify the Student class to include an ArrayList of Grade. Test the Student class before proceeding to the next step.
- Finish writing the GradeBook constructor to process the grades as they are read in from the data file. Test the GradeBook class before proceeding to the next step.
- Add additional functionality to the GradeBook and Student classes one method at a time.
Here is Grade.jave code is shown below.
GradeBook Class
The GradeBook class has a single instance variable roster, an ArrayList of Student. This class performs all operations related to the scores of all students in the data file. Starter code for the GradeBook class is provided.
GradeBook(String fileName)This constructor needs to be modified to work with the given file format. As a first step, read the student information from the data file, but ignore the grades for now. You will want to use the provided getFileScanner method, and for looping through the data, you will want to mimic the loop in the EmployeeManager constructor in L07-Employees Download L07-Employees. Note that here we are adding a student to the instance variable roster. Once you have this part of the constructor finished, you should be able to run your code and see the output of a long list of student information (without grades).
After creating the Grade class, you can return to working on this constructor to incorporate the reading of the grades. A suggestion would be that while reading the file a line at a time and parsing each line into tokens (such as first name, etc), create a loop over the grade-name/grade-value pairs. You may assume that the file is well-formatted, i.e., each line contains a first name, last name, age, major, username, and a number of grade-name/grade-value pairs. For each grade-name/grade-value pair, create a Grade, and then call the addGrade method you just wrote in the Student class. In summary, for each line of the file, you create a Student with first name, last name, age, major, and username, and then call the addGrade method in the Student class to add each grade to the Student object.
Grade Class
The Grade class will represent and store information about a single grade. A grade has two components: a name (stored as a String, e.g., "HW1"), and the score for that assignment (stored as an int). You will need to write a constructor for this class as well as a few methods. In particular, you will need instance methods to get a grade's name, get a grade's value, and change a grade value. The constructor of the Grade class will get the grade name and score as parameters.
Instance methods in the Grade class
In addition to the constructor, you will need to write the following instance methods in the Grade class. The required methods must be public. Test all your methods by calling them from main.
String getName()Accessor method that returns the name component of a Grade.int getScore()Accessor method that returns the score component of a Grade.String toString()Returns a String representation of a Grade, eg, "HW1 23".void changeGrade(int score)Change the score of an individual Grade.Student Class
The Student class will represent and store information about a single student. This class performs all operations related to a single student, such as constructing a Student and giving accessor/mutator methods. Starter code for the Student class is provided which stores the first name, last name, age, major, and username. After completing Steps 1 and 2, you will modify the Student class to include an ArrayList of grades, and will add instance methods to support the operations of the GradeBook class. Make the following modifications: (a) add a private instance variable grades that is an ArrayList of type Grade; (b) modify the constructors to initialize grades to a new ArrayList of type Grade [just one line to add]; (c) implement a new instance method addGrade() [just one line]; (d) complete method definitions for the other required instance methods listed below; (e) modify the Student.toString() method so the return statement becomes
return super.toString() + " " + username + " " + major + " " + gradesString();
(f) add code to the main method in the Student class to test the changes.
Printing result of call to student.getNameAndMajor(): Grace Hopper CSCI
Printing student after adding a grade with student.addGrade(new Grade("HW1", 95)): Grace Hopper 20 ghopper CSCI HW1 95
Q1 80, HW1 80, Q2 90, HW2 80, Q3 89, HW3 90, Q4 94, HW4 70, Q5 88, HW5 92, EXAM 89
![8
9
10
HHHHHUNG9272~N47º24
11
12
13
14
15
16
17
18
19
20
21
23
25
26
28
30
31
32
33
34
35
36
37
38
39
40
41
43
45
46
48
49
50
51
52
private ArrayList<Student> roster;
// Default constructor
public GradeBook() {
roster = new ArrayList<Student> ();
}
// Constructor fills roster with data from file
public GradeBook (String fileName) {
// TODO: fill this in
}
// String representation of GradeBook
public String toString() {
// TODO: modify this placeholder, which is a way to get started
// Loop through roster and print each student on its own line
return roster.toString();
}
// TODO: add definitions for the following instance methods:
void print IndividualGrades (String username)
//
// void printGrades ByMajor (String major)
// void removeStudent (String username)
//
void changeGrade (String username, String assignment, int newScore)
// Helper method to open a file for reading
public static Scanner getFileScanner(String filename) {
Scanner myFile;
try { myFile = new Scanner(new FileReader(filename)); }
catch (Exception e) {
System.out.println("File not found: " + filename);
return null;
}
}
return myFile;
}
// Helper method to open a file for writing
public static PrintWriter getFileWriter(String filename) {
PrintWriter outFile;
try { outFile = new PrintWriter(filename); }
catch (Exception e) {
System.out.println("Error opening file: + filename);
return null;
}
return outFile;
// Testing the GradeBook class
Run | Debug
public static void main(String[] args) {
GradeBook grades = new GradeBook (fileName: "data/S23grades.txt");
System.out.println (grades);
// TODO: add method calls here to test your code](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F385eb8fb-6648-4df6-8047-4f830c46ad3b%2Fe24fedda-b1ab-40a5-95a0-006502df2e06%2Fu29vmwq_processed.png&w=3840&q=75)
![34567
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
public class Person {
}
protected String firstName;
protected String lastName;
protected int age;
// Default constructor
public Person () {
firstName =
lastName = "";
age = 0;
}
// Constructs a Person from data sent as parameters
public Person (String first, String last, int age) {
firstName = first;
lastName = last;
this.age = age;
}
// Person increases in age by one year
public void birthday () {
age++;
}
||||
// Return String representation of Person
public String toString() {
return firstName + " " + lastName +
}
}
||||
+ age;
// Testing the Person class
Run | Debug
public static void main(String[] args) {
Person prof = new Person (first: "Amy", last: "Briggs", age: 39);
System.out.println(prof);
prof.birthday();
System.out.println(prof);](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F385eb8fb-6648-4df6-8047-4f830c46ad3b%2Fe24fedda-b1ab-40a5-95a0-006502df2e06%2Frqhrrmc_processed.png&w=3840&q=75)

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

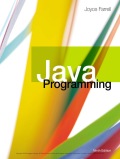
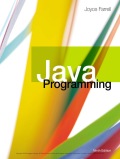