Create a graph containing the following edges and display the nodes of a graph in depth first traversal and breadth first traversal. V(G) = {0, 1, 2, 3, 4, 5, 6, 7, 8, 9, 10} E(G) = {(0, 1), (0, 5), (1, 2), (1, 3), (1, 5), (2, 4), (4, 3), (5, 6), (6, 8), (7, 3), (7, 8), (8, 10), (9, 4), (9, 7), (9, 10)} The input file should consist of the number of vertices in the graph in the first line and the vertices that are adjacent to the vertex in the following lines. Header File #ifndef H_graph #define H_graph #include #include #include #include "linkedList.h" #include "unorderedLinkedList.h" #include "linkedQueue.h" using namespace std; class graphType { public: bool isEmpty() const; void createGraph(); void clearGraph(); void printGraph() const; void depthFirstTraversal(); void dftAtVertex(int vertex); void breadthFirstTraversal(); graphType(int size = 0); ~graphType(); protected: int maxSize; //maximum number of vertices int gSize; //current number of vertices unorderedLinkedList *graph; //array to create //adjacency lists private: void dft(int v, bool visited[]); }; bool graphType::isEmpty() const { return (gSize == 0); } void graphType::createGraph() { ifstream infile; char fileName[50]; int index; int vertex; int adjacentVertex; if (gSize != 0) //if the graph is not empty, make it empty clearGraph(); cout << "Enter input file name: "; cin >> fileName; cout << endl; infile.open(fileName); if (!infile) { cout << "Cannot open input file." << endl; return; } infile >> gSize; //get the number of vertices for (index = 0; index < gSize; index++) { infile >> vertex; infile >> adjacentVertex; while (adjacentVertex != -999) { graph[vertex].insertLast(adjacentVertex); infile >> adjacentVertex; } //end while } // end for infile.close(); } //end createGraph void graphType::clearGraph() { int index; for (index = 0; index < gSize; index++) graph[index].destroyList(); gSize = 0; } //end clearGraph void graphType::printGraph() const { int index; for (index = 0; index < gSize; index++) { cout << index << " "; graph[index].print(); cout << endl; } cout << endl; } //end printGraph void graphType::depthFirstTraversal() { bool *visited; //pointer to create the array to keep //track of the visited vertices visited = new bool[gSize]; int index; for (index = 0; index < gSize; index++) visited[index] = false; //For each vertex that is not visited, do a depth //first traverssal for (index = 0; index < gSize; index++) if (!visited[index]) dft(index,visited); delete [] visited; } //end depthFirstTraversal void graphType::dft(int v, bool visited[]) { visited[v] = true; cout << " " << v << " "; //visit the vertex linkedListIterator graphIt; //for each vertex adjacent to v for (graphIt = graph[v].begin(); graphIt != graph[v].end(); ++graphIt) { int w = *graphIt; if (!visited[w]) dft(w, visited); } //end while } //end dft void graphType::dftAtVertex(int vertex) { bool *visited; visited = new bool[gSize]; for (int index = 0; index < gSize; index++) visited[index] = false; dft(vertex, visited); delete [] visited; } // end dftAtVertex void graphType::breadthFirstTraversal() { linkedQueueType queue; bool *visited; visited = new bool[gSize]; for (int ind = 0; ind < gSize; ind++) visited[ind] = false; //initialize the array //visited to false linkedListIterator graphIt; for (int index = 0; index < gSize; index++) if (!visited[index]) { queue.addQueue(index); visited[index] = true; cout << " " << index << " "; while (!queue.isEmptyQueue()) { int u = queue.front(); queue.deleteQueue(); for (graphIt = graph[u].begin(); graphIt != graph[u].end(); ++graphIt) { int w = *graphIt; if (!visited[w]) { queue.addQueue(w); visited[w] = true; cout << " " << w << " "; } } } //end while } delete [] visited; } //end breadthFirstTraversal //Constructor graphType::graphType(int size) { maxSize = size; gSize = 0; graph = new unorderedLinkedList[size]; } //Destructor graphType::~graphType() { clearGraph(); }
Create a graph containing the following edges and display the nodes of a graph in depth first traversal and breadth first traversal. V(G) = {0, 1, 2, 3, 4, 5, 6, 7, 8, 9, 10} E(G) = {(0, 1), (0, 5), (1, 2), (1, 3), (1, 5), (2, 4), (4, 3), (5, 6), (6, 8), (7, 3), (7, 8), (8, 10), (9, 4), (9, 7), (9, 10)} The input file should consist of the number of vertices in the graph in the first line and the vertices that are adjacent to the vertex in the following lines. Header File #ifndef H_graph #define H_graph #include #include #include #include "linkedList.h" #include "unorderedLinkedList.h" #include "linkedQueue.h" using namespace std; class graphType { public: bool isEmpty() const; void createGraph(); void clearGraph(); void printGraph() const; void depthFirstTraversal(); void dftAtVertex(int vertex); void breadthFirstTraversal(); graphType(int size = 0); ~graphType(); protected: int maxSize; //maximum number of vertices int gSize; //current number of vertices unorderedLinkedList *graph; //array to create //adjacency lists private: void dft(int v, bool visited[]); }; bool graphType::isEmpty() const { return (gSize == 0); } void graphType::createGraph() { ifstream infile; char fileName[50]; int index; int vertex; int adjacentVertex; if (gSize != 0) //if the graph is not empty, make it empty clearGraph(); cout << "Enter input file name: "; cin >> fileName; cout << endl; infile.open(fileName); if (!infile) { cout << "Cannot open input file." << endl; return; } infile >> gSize; //get the number of vertices for (index = 0; index < gSize; index++) { infile >> vertex; infile >> adjacentVertex; while (adjacentVertex != -999) { graph[vertex].insertLast(adjacentVertex); infile >> adjacentVertex; } //end while } // end for infile.close(); } //end createGraph void graphType::clearGraph() { int index; for (index = 0; index < gSize; index++) graph[index].destroyList(); gSize = 0; } //end clearGraph void graphType::printGraph() const { int index; for (index = 0; index < gSize; index++) { cout << index << " "; graph[index].print(); cout << endl; } cout << endl; } //end printGraph void graphType::depthFirstTraversal() { bool *visited; //pointer to create the array to keep //track of the visited vertices visited = new bool[gSize]; int index; for (index = 0; index < gSize; index++) visited[index] = false; //For each vertex that is not visited, do a depth //first traverssal for (index = 0; index < gSize; index++) if (!visited[index]) dft(index,visited); delete [] visited; } //end depthFirstTraversal void graphType::dft(int v, bool visited[]) { visited[v] = true; cout << " " << v << " "; //visit the vertex linkedListIterator graphIt; //for each vertex adjacent to v for (graphIt = graph[v].begin(); graphIt != graph[v].end(); ++graphIt) { int w = *graphIt; if (!visited[w]) dft(w, visited); } //end while } //end dft void graphType::dftAtVertex(int vertex) { bool *visited; visited = new bool[gSize]; for (int index = 0; index < gSize; index++) visited[index] = false; dft(vertex, visited); delete [] visited; } // end dftAtVertex void graphType::breadthFirstTraversal() { linkedQueueType queue; bool *visited; visited = new bool[gSize]; for (int ind = 0; ind < gSize; ind++) visited[ind] = false; //initialize the array //visited to false linkedListIterator graphIt; for (int index = 0; index < gSize; index++) if (!visited[index]) { queue.addQueue(index); visited[index] = true; cout << " " << index << " "; while (!queue.isEmptyQueue()) { int u = queue.front(); queue.deleteQueue(); for (graphIt = graph[u].begin(); graphIt != graph[u].end(); ++graphIt) { int w = *graphIt; if (!visited[w]) { queue.addQueue(w); visited[w] = true; cout << " " << w << " "; } } } //end while } delete [] visited; } //end breadthFirstTraversal //Constructor graphType::graphType(int size) { maxSize = size; gSize = 0; graph = new unorderedLinkedList[size]; } //Destructor graphType::~graphType() { clearGraph(); }
Chapter7: Input/output Technology
Section: Chapter Questions
Problem 12VE
Related questions
Question
Create a graph containing the following edges and display the nodes of a graph in depth first traversal and breadth first traversal.
V(G) = {0, 1, 2, 3, 4, 5, 6, 7, 8, 9, 10}
E(G) = {(0, 1), (0, 5), (1, 2), (1, 3), (1, 5), (2, 4), (4, 3), (5, 6), (6, 8), (7, 3), (7, 8), (8, 10), (9, 4), (9, 7), (9, 10)}
The input file should consist of the number of vertices in the graph in the first line and the vertices that are adjacent to the vertex in the following lines.
Header File
#ifndef H_graph
#define H_graph
#include <iostream>
#include <fstream>
#include <iomanip>
#include "linkedList.h"
#include "unorderedLinkedList.h"
#include "linkedQueue.h"
using namespace std;
class graphType
{
public:
bool isEmpty() const;
void createGraph();
void clearGraph();
void printGraph() const;
void depthFirstTraversal();
void dftAtVertex(int vertex);
void breadthFirstTraversal();
graphType(int size = 0);
~graphType();
protected:
int maxSize; //maximum number of vertices
int gSize; //current number of vertices
unorderedLinkedList<int> *graph; //array to create
//adjacency lists
private:
void dft(int v, bool visited[]);
};
bool graphType::isEmpty() const
{
return (gSize == 0);
}
void graphType::createGraph()
{
ifstream infile;
char fileName[50];
int index;
int vertex;
int adjacentVertex;
if (gSize != 0) //if the graph is not empty, make it empty
clearGraph();
cout << "Enter input file name: ";
cin >> fileName;
cout << endl;
infile.open(fileName);
if (!infile)
{
cout << "Cannot open input file." << endl;
return;
}
infile >> gSize; //get the number of vertices
for (index = 0; index < gSize; index++)
{
infile >> vertex;
infile >> adjacentVertex;
while (adjacentVertex != -999)
{
graph[vertex].insertLast(adjacentVertex);
infile >> adjacentVertex;
} //end while
} // end for
infile.close();
} //end createGraph
void graphType::clearGraph()
{
int index;
for (index = 0; index < gSize; index++)
graph[index].destroyList();
gSize = 0;
} //end clearGraph
void graphType::printGraph() const
{
int index;
for (index = 0; index < gSize; index++)
{
cout << index << " ";
graph[index].print();
cout << endl;
}
cout << endl;
} //end printGraph
void graphType::depthFirstTraversal()
{
bool *visited; //pointer to create the array to keep
//track of the visited vertices
visited = new bool[gSize];
int index;
for (index = 0; index < gSize; index++)
visited[index] = false;
//For each vertex that is not visited, do a depth
//first traverssal
for (index = 0; index < gSize; index++)
if (!visited[index])
dft(index,visited);
delete [] visited;
} //end depthFirstTraversal
void graphType::dft(int v, bool visited[])
{
visited[v] = true;
cout << " " << v << " "; //visit the vertex
linkedListIterator<int> graphIt;
//for each vertex adjacent to v
for (graphIt = graph[v].begin(); graphIt != graph[v].end();
++graphIt)
{
int w = *graphIt;
if (!visited[w])
dft(w, visited);
} //end while
} //end dft
void graphType::dftAtVertex(int vertex)
{
bool *visited;
visited = new bool[gSize];
for (int index = 0; index < gSize; index++)
visited[index] = false;
dft(vertex, visited);
delete [] visited;
} // end dftAtVertex
void graphType::breadthFirstTraversal()
{
linkedQueueType<int> queue;
bool *visited;
visited = new bool[gSize];
for (int ind = 0; ind < gSize; ind++)
visited[ind] = false; //initialize the array
//visited to false
linkedListIterator<int> graphIt;
for (int index = 0; index < gSize; index++)
if (!visited[index])
{
queue.addQueue(index);
visited[index] = true;
cout << " " << index << " ";
while (!queue.isEmptyQueue())
{
int u = queue.front();
queue.deleteQueue();
for (graphIt = graph[u].begin();
graphIt != graph[u].end(); ++graphIt)
{
int w = *graphIt;
if (!visited[w])
{
queue.addQueue(w);
visited[w] = true;
cout << " " << w << " ";
}
}
} //end while
}
delete [] visited;
} //end breadthFirstTraversal
//Constructor
graphType::graphType(int size)
{
maxSize = size;
gSize = 0;
graph = new unorderedLinkedList<int>[size];
}
//Destructor
graphType::~graphType()
{
clearGraph();
}
#endif

Transcribed Image Text:Example input file contents:
5
0134-999
12-999
2 1-999
314-999
the number of vertices
Vertex 0: 1, 3, 4 are adjacent
Vertex 1: 2 are adjacent
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 3 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
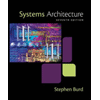
Systems Architecture
Computer Science
ISBN:
9781305080195
Author:
Stephen D. Burd
Publisher:
Cengage Learning
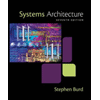
Systems Architecture
Computer Science
ISBN:
9781305080195
Author:
Stephen D. Burd
Publisher:
Cengage Learning