class PriceChecker(): # Constructor def __init__(self): self.levelsList = [] # Properties # A property is defined like a method, but you use it in your # code like a variable (no parentheses need to followed it when used in your code) # Refer: https://www.youtube.com/watch?v=jCzT9XFZ5bw # Refer BP411 slides: Week 2 - Chapter 10 - Slides about Encapsulation and properties @property def levelsList(self): return self.__levelsList @levelsList.setter def levelsList(self, newValue): self.__levelsList = newValue # Class Methods # ============= # Method: Sort and Display the levelsList def displayList(self): print(chr(27) + "[2J") # Clear the screen print("Price Levels In The List") print("========================") # Sort the list in reverse order ... # Print the items in the list (Based on the above sort, numbers should appear from large to small.) ... # Display the menu and get user input about what methods to execute next def displayMenu(self): min = 0 max = 3 errorMsg = "Please enter a valid option between " + str(min) + " and " + str(max) print("MENU OPTIONS") print("============") print("1. Add a price level") print("2. Remove a price level") print("3. Remove all price levels") print("0. Exit the program") print(" ") # Get user input. Keep on requesting input until the user enters a valid number between min and max selection = 99 while selection < min or selection > max: try: selection = int(input("Please enter one of the options: ")) except: print(errorMsg) # user did not enter a number continue # skip the following if statement if(selection < min or selection > max): print(errorMsg) # user entered a number outside the required range return selection # When this return is finally reached, selection will have a value between (and including) min and max # Method: Append a new price level to the levelsList def addLevel(self): try: # Let the user enter a new float value and append it to the list ... except: # Print and error message if the user entered invalid input ... # Method: Remove an existing price level from the levelsList def removeLevel(self): try: # Let the user enter a new float value. If found in the list, remove it from the list ... except: # Print and error message if the user entered invalid input ... # Method: Set levelsList to an empty list def removeAllLevels(self): # Set levelsList to an empty list ... # ************************************************************************************************* # Main Code Section # ************************************************************************************************* # Create an object based on the PriceChecker class checkerObj = PriceChecker() # Display the levelsList and Menu; and then get user input for what actions to take userInput = 99 while userInput != 0: checkerObj.displayList() userInput = checkerObj.displayMenu() if(userInput == 1): checkerObj.addLevel() elif(userInput == 2): checkerObj.removeLevel() elif(userInput == 3): checkerObj.removeAllLevels()
class PriceChecker():
# Constructor
def __init__(self):
self.levelsList = []
# Properties
# A property is defined like a method, but you use it in your
# code like a variable (no parentheses need to followed it when used in your code)
# Refer: https://www.youtube.com/watch?v=jCzT9XFZ5bw
# Refer BP411 slides: Week 2 - Chapter 10 - Slides about Encapsulation and properties
@property
def levelsList(self):
return self.__levelsList
@levelsList.setter
def levelsList(self, newValue):
self.__levelsList = newValue
# Class Methods
# =============
# Method: Sort and Display the levelsList
def displayList(self):
print(chr(27) + "[2J") # Clear the screen
print("Price Levels In The List")
print("========================")
# Sort the list in reverse order
...
# Print the items in the list (Based on the above sort, numbers should appear from large to small.)
...
# Display the menu and get user input about what methods to execute next
def displayMenu(self):
min = 0
max = 3
errorMsg = "Please enter a valid option between " + str(min) + " and " + str(max)
print("MENU OPTIONS")
print("============")
print("1. Add a price level")
print("2. Remove a price level")
print("3. Remove all price levels")
print("0. Exit the program")
print(" ")
# Get user input. Keep on requesting input until the user enters a valid number between min and max
selection = 99
while selection < min or selection > max:
try:
selection = int(input("Please enter one of the options: "))
except:
print(errorMsg) # user did not enter a number
continue # skip the following if statement
if(selection < min or selection > max):
print(errorMsg) # user entered a number outside the required range
return selection # When this return is finally reached, selection will have a value between (and including) min and max
# Method: Append a new price level to the levelsList
def addLevel(self):
try:
# Let the user enter a new float value and append it to the list
...
except:
# Print and error message if the user entered invalid input
...
# Method: Remove an existing price level from the levelsList
def removeLevel(self):
try:
# Let the user enter a new float value. If found in the list, remove it from the list
...
except:
# Print and error message if the user entered invalid input
...
# Method: Set levelsList to an empty list
def removeAllLevels(self):
# Set levelsList to an empty list
...
# *************************************************************************************************
# Main Code Section
# *************************************************************************************************
# Create an object based on the PriceChecker class
checkerObj = PriceChecker()
# Display the levelsList and Menu; and then get user input for what actions to take
userInput = 99
while userInput != 0:
checkerObj.displayList()
userInput = checkerObj.displayMenu()
if(userInput == 1):
checkerObj.addLevel()
elif(userInput == 2):
checkerObj.removeLevel()
elif(userInput == 3):
checkerObj.removeAllLevels()

Step by step
Solved in 4 steps with 2 images

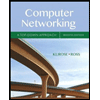
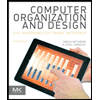
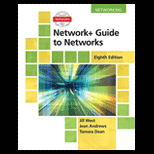
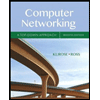
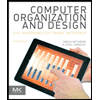
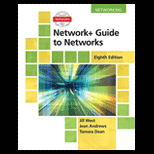
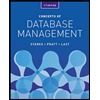
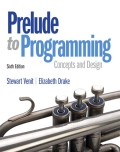
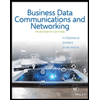