Answer the given question with a proper explanation and step-by-step solution. Instructions Create a VS C++ project using the name format: firstname_lastname_06 The program will ask for values (int, double, string, or any datatype that's in your class' attributes) to initialize an array of 5 objects of your Assignment 1's class. Note: If you received feedback about Assignment 1 design, update it accordingly. Then, sort the list using the insertion sort algorithm and display the content of the list. Make sure to modify the insertion sort algorithm from your textbook to work with an array of your Assignment 1's class. Your class may also need to be modified by implementing a few operator overloading functions. Submission Files to submit: Project/solution-related files Your class (.h and .cpp) main file (.cpp) Submit the project in a zip folder. Assignment 1 Car.h #pragma once #include using namespace std; class Car { private: int maxSpeed; //maximum speed of the car string model; //model of the car double price; //price of the car public: Car(); Car(string mdl, int m, double p); int getMaxSpeed(); void setMaxSpeed(int m); string getModel(); void setModel(string mdl); double getPrice(); void setPrice(double p); void display(); //destructor ~Car(); }; Assignment 1 Car.cpp #include #include "Car.h" using namespace std; Car::Car() { //default values of Car object model = "No defined"; maxSpeed = 80; price = 10000; } //parameterized constructor Car::Car(string mdl, int m, double p) { //applying business rule for maxSpeed if (m > 0 && m < 130) maxSpeed = m; else maxSpeed = 80; model = mdl; price = p; } //accessor and mutator int Car::getMaxSpeed() { return maxSpeed; } void Car::setMaxSpeed(int m) { //applying business rule for maxSpeed if (m > 0 && m < 130) maxSpeed = m; else maxSpeed = 80; } string Car::getModel() { return model; } void Car::setModel(string mdl) { model = mdl; } double Car::getPrice() { return price; } void Car::setPrice(double p) { price = p; } Car::~Car() { cout << "An Car object has been removed from memory." << endl; } void Car::display() { cout << "Model: " << model << ", maxspeed = " << maxSpeed << ", price = " << price << endl; } Assignment 1 Comments/mistake was Missing const modifier for accessors. Please Modify both file .h and .cpp and create the main file.
Answer the given question with a proper explanation and step-by-step solution.
Instructions
Create a VS C++ project using the name format:
firstname_lastname_06
The program will ask for values (int, double, string, or any datatype that's in your class' attributes) to initialize an array of 5 objects of your Assignment 1's class.
Note: If you received feedback about Assignment 1 design, update it accordingly.
Then, sort the list using the insertion sort
Make sure to modify the insertion sort algorithm from your textbook to work with an array of your Assignment 1's class.
Your class may also need to be modified by implementing a few operator overloading functions.
Submission
Files to submit:
Project/solution-related files
Your class (.h and .cpp)
main file (.cpp)
Submit the project in a zip folder.
Assignment 1 Car.h
#pragma once
#include <iostream>
using namespace std;
class Car
{
private:
int maxSpeed; //maximum speed of the car
string model; //model of the car
double price; //price of the car
public:
Car();
Car(string mdl, int m, double p);
int getMaxSpeed();
void setMaxSpeed(int m);
string getModel();
void setModel(string mdl);
double getPrice();
void setPrice(double p);
void display();
//destructor
~Car();
};
Assignment 1 Car.cpp
#include <iostream>
#include "Car.h"
using namespace std;
Car::Car()
{
//default values of Car object
model = "No defined";
maxSpeed = 80;
price = 10000;
}
//parameterized constructor
Car::Car(string mdl, int m, double p)
{
//applying business rule for maxSpeed
if (m > 0 && m < 130)
maxSpeed = m;
else
maxSpeed = 80;
model = mdl;
price = p;
}
//accessor and mutator
int Car::getMaxSpeed()
{
return maxSpeed;
}
void Car::setMaxSpeed(int m)
{
//applying business rule for maxSpeed
if (m > 0 && m < 130)
maxSpeed = m;
else
maxSpeed = 80;
}
string Car::getModel()
{
return model;
}
void Car::setModel(string mdl)
{
model = mdl;
}
double Car::getPrice()
{
return price;
}
void Car::setPrice(double p)
{
price = p;
}
Car::~Car()
{
cout << "An Car object has been removed from memory." << endl;
}
void Car::display()
{
cout << "Model: " << model << ", maxspeed = " << maxSpeed << ", price = " << price << endl;
}
Assignment 1 Comments/mistake was Missing const modifier for accessors.
Please Modify both file .h and .cpp and create the main file. Thank you!

Trending now
This is a popular solution!
Step by step
Solved in 3 steps

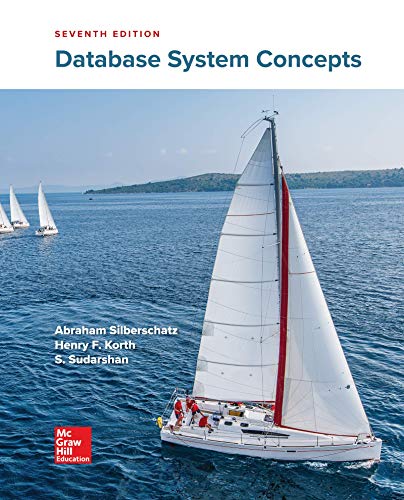
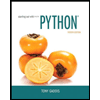
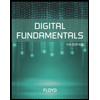
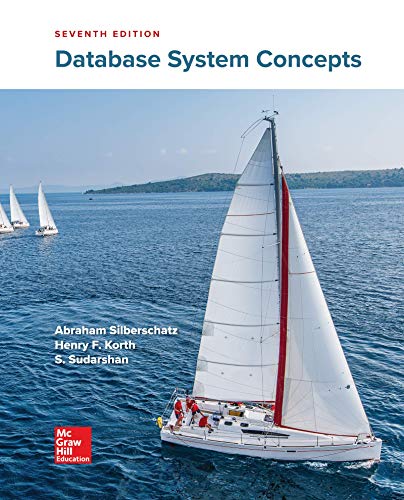
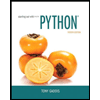
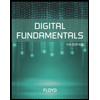
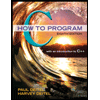
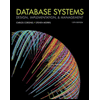
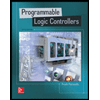