Serialization and Deserialization using Json and XML The Atom class This is the front end of the application. There are 12 members. Atom Class Fields Properties + «c# property, set» Name : string + «c# property, set» Symbol : string + «c# property, set» Proton : int + «c# property, set» Neutron : int + «c# property, set» Weight : double Methods + «c# constructor» Atom() + «c# constructor» Atom( string name, int proton, int neutron, double weight, string symbol) $+ Parse(string objectData) : Atom $+ GetAtoms() : List + ToString() : double Description of class members Fields: There are no fields in this class. Properties: All the properties have public get and set Name – this is a string representing the name of this atom. Both the getter and setter are public. Symbol – this is a two-letter string representing the name of this atom that is used in chemistry. Both the getter and setter are public. Proton – this is an int representing the number of protons in the nucleus of this atom. Both the getter and setter are public. Neutron – this is a int representing the number of neutrons in the nucleus of this atom. Both the getter and setter are public. Weight – this is a double representing the atomic weight of this atom. Both the getter and setter are public. Constructor: There are two constructors for this class: a default one and a user defined one public Atom() – This is a default constructor that is necessary for serialization. This is an empty constructor that does not do anything. public Atom(string name, int proton, int neutron, double weight, string symbol) – This constructor assigns the arguments to the appropriate fields. Methods public static Atom Parse(string line) – This is a public class method that takes a string and returns an Atom object. The argument is one string that is comprised of the five fields of this object. It does the following: It uses the Split() method to parse the argument into five parts. Create an Atom object and initialize the fields with the appropriate parts. Return the above object You will need to examine the arguments to decide what part will be assigned to which field. public override string ToString() – This is a public method overrides the corresponding method in the object class to return a string form of the object. Test Harness Insert the following code statements in your Program.cs file: Write or create Four methods that will be called from Main. The Write method will serialize a collection of atoms to a file and the Read method will deserialized the contents of a file to a list of atoms. static void WriteJson(List atoms, string filename) – This void class method that takes the list of atoms and a filename. You will write the necessary code to serialize the first argument to the specified file. static void ReadJson(string filename) – This void class method that takes a filename. You will write the necessary code to deserialize the file contents to a List and then display all atoms. static void WriteXML(List atoms, string filename) – This void class method that takes the list of atoms and a filename. You will write the necessary code to serialize the first argument to the specified file. static void ReadXML(string filename) – This void class method that takes a filename. You will write the necessary code to deserialize the file contents to a List and then display all atoms. Appendix Code for the GetAtoms method of the Atom class
OOPs
In today's technology-driven world, computer programming skills are in high demand. The object-oriented programming (OOP) approach is very much useful while designing and maintaining software programs. Object-oriented programming (OOP) is a basic programming paradigm that almost every developer has used at some stage in their career.
Constructor
The easiest way to think of a constructor in object-oriented programming (OOP) languages is:
Serialization and Deserialization using Json and XML
The Atom class
This is the front end of the application. There are 12 members.
Atom Class |
Fields |
|
Properties |
+ «c# property, set» Name : string + «c# property, set» Symbol : string + «c# property, set» Proton : int + «c# property, set» Neutron : int + «c# property, set» Weight : double
|
Methods |
+ «c# constructor» Atom() + «c# constructor» Atom( $+ Parse(string objectData) : Atom $+ GetAtoms() : List<Atom> + ToString() : double
|
Description of class members
Fields:
There are no fields in this class.
Properties:
All the properties have public get and set
Name – this is a string representing the name of this atom. Both the getter and setter are public.
Symbol – this is a two-letter string representing the name of this atom that is used in chemistry. Both the getter and setter are public.
Proton – this is an int representing the number of protons in the nucleus of this atom. Both the getter and setter are public.
Neutron – this is a int representing the number of neutrons in the nucleus of this atom. Both the getter and setter are public.
Weight – this is a double representing the atomic weight of this atom. Both the getter and setter are public.
Constructor:
There are two constructors for this class: a default one and a user defined one
public Atom() – This is a default constructor that is necessary for serialization. This is an empty constructor that does not do anything.
public Atom(string name, int proton, int neutron, double weight, string symbol) – This constructor assigns the arguments to the appropriate fields.
Methods
public static Atom Parse(string line) – This is a public class method that takes a string and returns an Atom object. The argument is one string that is comprised of the five fields of this object. It does the following:
- It uses the Split() method to parse the argument into five parts.
- Create an Atom object and initialize the fields with the appropriate parts.
- Return the above object
You will need to examine the arguments to decide what part will be assigned to which field.
public override string ToString() – This is a public method overrides the corresponding method in the object class to return a string form of the object.
Test Harness
Insert the following code statements in your Program.cs file:
Write or create Four methods that will be called from Main. The Write method will serialize a collection of atoms to a file and the Read method will deserialized the contents of a file to a list of atoms.
static void WriteJson(List<Atom> atoms, string filename) – This void class method that takes the list of atoms and a filename. You will write the necessary code to serialize the first argument to the specified file.
static void ReadJson(string filename) – This void class method that takes a filename. You will write the necessary code to deserialize the file contents to a List<Atom> and then display all atoms.
static void WriteXML(List<Atom> atoms, string filename) – This void class method that takes the list of atoms and a filename. You will write the necessary code to serialize the first argument to the specified file.
static void ReadXML(string filename) – This void class method that takes a filename. You will write the necessary code to deserialize the file contents to a List<Atom> and then display all atoms.
Appendix
Code for the GetAtoms method of the Atom class



Step by step
Solved in 2 steps with 3 images

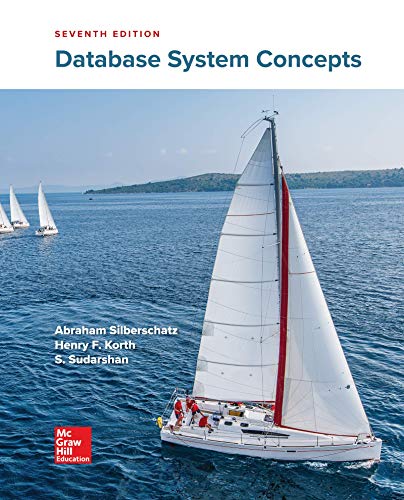
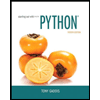
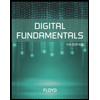
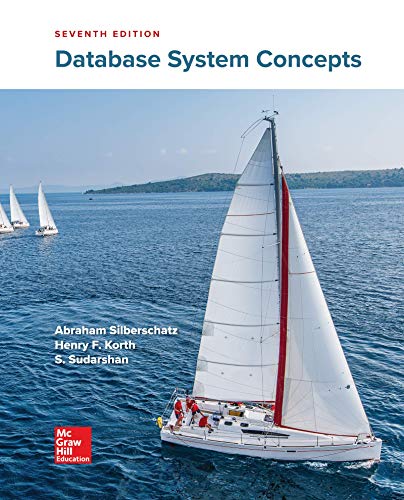
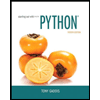
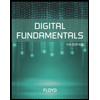
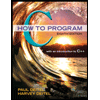
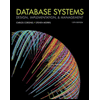
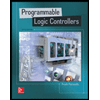