Program Specifications Write a program to play an automated game of Rock, Paper, Scissors. Two players make one of three hand signals at the same time. Hand signals represent a rock, a piece of paper, or a pair of scissors. Each combination results in a win for one of the players. Rock crushes scissors, paper covers rock, and scissors cut paper. A tie occurs if both players make the same signal. Use a random number generator of 0, 1, or 2 to represent the three signals. Note: this program is designed for incremental development. Complete each step and submit for grading before starting the next step. Only a portion of tests pass after each step but confirm progress. Step 0. Read starter template and do not change the provided code. Integer constants are defined for ROCK, PAPER, and SCISSORS. A seed is read from input to initialize the random number generator. This supports automated testing and creates predictable results that would otherwise be random. Step 1 . Read two player names from input (string). Read number of rounds from input. Continue reading number of rounds if value is below one and provide an error message. Output player names and number of rounds. Assume each player name contains less than 40 characters. Submit for grading to confirm 2 tests pass. Ex: If input is: 3 Anna Bert -3 -4 4 Sample output is: Rounds must be > 0 Rounds must be > 0 Anna vs Bert for 4 rounds Step 2 . Use rand() % 3 to generate random values (0 - 2) for player 1 followed by player 2. Continue to generate random values for both players until both values do not match. Output "Tie" when the values match. Submit for grading to confirm 3 tests pass. Ex: If input is: 10 Anna Bert 1 Sample output is: Anna vs Bert for 1 rounds Tie Tie Step 3 . Identify winner for this round and output a message. Rock crushes scissors, scissors cut paper, and paper covers rock. Submit for grading to confirm 6 tests pass. Ex: If input is: 39 Anna Bert 1 Sample output is: Anna vs Bert for 1 rounds Tie Tie Bert wins with scissors Step 4 . Add a loop to repeat steps 2 and 3 for the number of rounds. Output total wins for each player after all rounds are complete. Submit for grading to confirm all tests pass. Ex: If input is: 82 Anna Bert 3 Sample output is: Anna vs Bert for 3 rounds Anna wins with paper Tie Anna wins with rock Anna wins with paper Anna wins 3 and Bert wins 0 #include #include int main() { constint ROCK = 0; constint PAPER = 1; constint SCISSORS = 2; int seed; scanf("%d", &seed); srand(seed); /* Type your code here. */ return0; }
Program Specifications Write a program to play an automated game of Rock, Paper, Scissors. Two players make one of three hand signals at the same time. Hand signals represent a rock, a piece of paper, or a pair of scissors. Each combination results in a win for one of the players. Rock crushes scissors, paper covers rock, and scissors cut paper. A tie occurs if both players make the same signal. Use a random number generator of 0, 1, or 2 to represent the three signals.
Note: this program is designed for incremental development. Complete each step and submit for grading before starting the next step. Only a portion of tests pass after each step but confirm progress.
Step 0. Read starter template and do not change the provided code. Integer constants are defined for ROCK, PAPER, and SCISSORS. A seed is read from input to initialize the random number generator. This supports automated testing and creates predictable results that would otherwise be random.
Step 1 . Read two player names from input (string). Read number of rounds from input. Continue reading number of rounds if value is below one and provide an error message. Output player names and number of rounds. Assume each player name contains less than 40 characters. Submit for grading to confirm 2 tests pass.
Ex: If input is:
Sample output is:
Rounds must be > 0
Step 2 . Use rand() % 3 to generate random values (0 - 2) for player 1 followed by player 2. Continue to generate random values for both players until both values do not match. Output "Tie" when the values match. Submit for grading to confirm 3 tests pass.
Ex: If input is:
Sample output is:
Anna vs Bert for 1 rounds
Step 3 . Identify winner for this round and output a message. Rock crushes scissors, scissors cut paper, and paper covers rock. Submit for grading to confirm 6 tests pass.
Ex: If input is:
Sample output is:
Anna vs Bert for 1 rounds
Step 4 . Add a loop to repeat steps 2 and 3 for the number of rounds. Output total wins for each player after all rounds are complete. Submit for grading to confirm all tests pass.
Ex: If input is:
Sample output is:
Anna vs Bert for 3 rounds
Trending now
This is a popular solution!
Step by step
Solved in 5 steps with 4 images

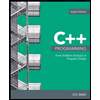
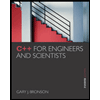
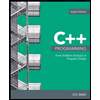
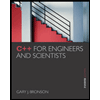