1. Develop a class Volume that stores the volume for a stereo that has a value between 0 and 11. Usage of the class is listed below the problem descriptions. Throughout the class you must guarantee that: O The numeric value of the Volume is set to a number between 0 and 11. Any attempt to set the value to greater than 11 will set it to 11 instead, any attempt to set a negative value will instead set it to 0. O This applies to the following methods below:_init_, set, up, down You must write the following methods: _init_-constructor. Construct a Volume that is set to a given numeric value, or, if no number given, defaults the value to 0. (Subject to 0 <= vol <=11 constraint above.) _repr_- converts Volume to a str for display, see runs below. O set - sets the volume to the specified argument (Subject to 0<= vol <=11 constraint above.) O get - returns the numeric value of the Volume O up - given a numeric amount, increases the Volume by that amount. (Subject to 0 <= vol <=11 constraint above.) O down - given a numeric amount, decreases the Volume by that amount. (Subject to 0 <= vol <=11 constraint above.) implements the operator ==. Returns True if the two Volumes have the same value and eq False otherwise. ##### Volume ##### # set and get >>> v = Volume() >>> v.set(5.3) >>> v Volume (5.3) >>> v.get() 5.3 >>> v.get() ==5.3 # return not print True >>> # _init_, __repr__, up, down >>> v = Volume (4.5) # set Volume with value >>> v Volume (4.5) >>> v.up(1.4) >>> v Volume (5.9) >>> v.up(6) # should max out at 11 >>> v Volume (11) >>> v.down( 3.5) >>> v Volume (7.5) >>> v.down (10) # minimum must be 0 >>> v Volume (0) * default arguments for _init_ >>> v - Volume () # Volume defaults to 0 >>> v Volume (0) # can compare Volumes using = >>> # comparisons >>> v - Volume (5) >>> v.up(1.1) >>> v - Volume (6.1) True >>> Volume ( 3.1) == Volume(3.2) False # constructor cannot set the Volume greater # than 11 or less than 0 >>> v - Volume (20) >>> v Volume (11) >>> v - Volume (-1) >>> v Volume (0) >>>
1. Develop a class Volume that stores the volume for a stereo that has a value between 0 and 11. Usage of the class is listed below the problem descriptions. Throughout the class you must guarantee that: O The numeric value of the Volume is set to a number between 0 and 11. Any attempt to set the value to greater than 11 will set it to 11 instead, any attempt to set a negative value will instead set it to 0. O This applies to the following methods below:_init_, set, up, down You must write the following methods: _init_-constructor. Construct a Volume that is set to a given numeric value, or, if no number given, defaults the value to 0. (Subject to 0 <= vol <=11 constraint above.) _repr_- converts Volume to a str for display, see runs below. O set - sets the volume to the specified argument (Subject to 0<= vol <=11 constraint above.) O get - returns the numeric value of the Volume O up - given a numeric amount, increases the Volume by that amount. (Subject to 0 <= vol <=11 constraint above.) O down - given a numeric amount, decreases the Volume by that amount. (Subject to 0 <= vol <=11 constraint above.) implements the operator ==. Returns True if the two Volumes have the same value and eq False otherwise. ##### Volume ##### # set and get >>> v = Volume() >>> v.set(5.3) >>> v Volume (5.3) >>> v.get() 5.3 >>> v.get() ==5.3 # return not print True >>> # _init_, __repr__, up, down >>> v = Volume (4.5) # set Volume with value >>> v Volume (4.5) >>> v.up(1.4) >>> v Volume (5.9) >>> v.up(6) # should max out at 11 >>> v Volume (11) >>> v.down( 3.5) >>> v Volume (7.5) >>> v.down (10) # minimum must be 0 >>> v Volume (0) * default arguments for _init_ >>> v - Volume () # Volume defaults to 0 >>> v Volume (0) # can compare Volumes using = >>> # comparisons >>> v - Volume (5) >>> v.up(1.1) >>> v - Volume (6.1) True >>> Volume ( 3.1) == Volume(3.2) False # constructor cannot set the Volume greater # than 11 or less than 0 >>> v - Volume (20) >>> v Volume (11) >>> v - Volume (-1) >>> v Volume (0) >>>
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
In Python IDLE: How do you write a class to satisfy the proble in the attached image?

Transcribed Image Text:1. Develop a class Volume that stores the volume for a stereo that has a value between 0 and 11. Usage of the
class is listed below the problem descriptions. Throughout the class you must guarantee that:
O The numeric value of the Volume is set to a number between 0 and 11. Any attempt to set the value
to greater than 11 will set it to 11 instead, any attempt to set a negative value will instead set it to 0.
O This applies to the following methods below:_init__, set, up, down
You must write the following methods:
init_- constructor. Construct a Volume that is set to a given numeric value, or, if no number
given, defaults the value to 0. (Subject to 0 <= vol <=11 constraint above.)
_repr_ - converts Volume to a str for display, see runs below.
O set - sets the volume to the specified argument (Subject to 0 <= vol <=11 constraint above.)
O get - returns the numeric value of the Volume
O up – given a numeric amount, increases the Volume by that amount. (Subject to 0 <= vol <=11
constraint above.)
O down – given a numeric amount, decreases the Volume by that amount. (Subject to 0 <= vol <=11
constraint above.)
eq_ - implements the operator ==. Returns True if the two Volumes have the same value and
False otherwise.
##### Volume #####
# set and get
>>> v = Volume ()
>>> v.set(5.3)
>>> v
Volume (5.3)
>>> v.get()
5.3
>>> v.get () ==5.3 # return not print
True
>>>
# _init_, __repr__, up, down
>>> v = Volume (4.5) # set Volume with value
>>> v
Volume (4.5)
>>> v.up(1.4)
>>> v
Volume (5.9)
>>> v.up(6) # should max out at 11
>>> v
Volume (11)
>>> v.down ( 3.5)
>>> v
Volume (7.5)
>>> v.down (10) # minimum must be 0
>>> v
Volume (0)
# default arguments for _init_
>>> v = Volume () # Volume defaults to 0
>>> v
Volume (0)
# can compare Volumes using ==
>>> # comparisons
>>> v = Volume (5)
>>> v.up(1.1)
>>> v =- volume (6.1)
True
>>> Volume ( 3.1) == Volume (3.2)
False
# constructor cannot set the Volume greater
# than 11 or less than 0
>>> v = Volume (20)
>>> v
Volume (11)
>>> v = Volume (-1)
>>> v
Volume (0)
>>>
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 2 images

Recommended textbooks for you
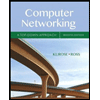
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
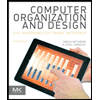
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
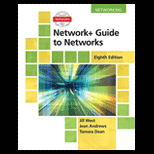
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
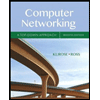
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
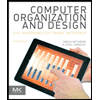
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
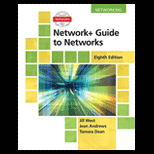
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
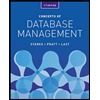
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
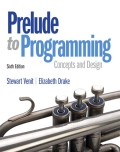
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
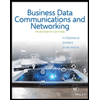
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY