[1] : import matplotlib.pyplot as plt import numpy as np # Calculate the area using the formula: A = L x W def calculate_area (L, W): return L * W # Example values L = 10 # Example length W = 20 # Example width A calculate_area (L, W) print("Area: ", A) #Assuming we have multiple values of A for histogram plotting A_values= [calculate_area(i, i+5) for i in range (10, 30)] #Plotting the histogram for A plt.figure (figsize=(9, 6)) plt.hist (A_values, np.arange (250, 505, 5), facecolor='darkblue', alpha=0.75) plt.xlabel('area (mm$^2$) ') plt.ylabel('number of measurements per bin') plt.show()
[1] : import matplotlib.pyplot as plt import numpy as np # Calculate the area using the formula: A = L x W def calculate_area (L, W): return L * W # Example values L = 10 # Example length W = 20 # Example width A calculate_area (L, W) print("Area: ", A) #Assuming we have multiple values of A for histogram plotting A_values= [calculate_area(i, i+5) for i in range (10, 30)] #Plotting the histogram for A plt.figure (figsize=(9, 6)) plt.hist (A_values, np.arange (250, 505, 5), facecolor='darkblue', alpha=0.75) plt.xlabel('area (mm$^2$) ') plt.ylabel('number of measurements per bin') plt.show()
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
100%
Please do the histogram part.
![In [1]:
The surface area of the rectangle bounded by L and Wis
Translate this equation into code and verify a few values of A with your calculator. Again, this code is simple a one-liner.
import matplotlib.pyplot as plt
import numpy as np
# Calculate the area using the formula: A = L x W
def calculate_area (L, W):
return L * W
# Example values
L = 10 # Example length
W = 20 # Example width
A = calculate_area (L, W)
print("Area:", A)
#Assuming we have multiple values of A for histogram plotting
A_values = [calculate_area(i, i+5) for i in range (10, 30)]
#Plotting the histogram for A
plt.figure (figsize=(9, 6))
plt.hist(A_values, np.arange (250, 505, 5), facecolor='darkblue', alpha=0.75)
plt.xlabel('area (mm$^2$)')
plt.ylabel('number of measurements per bin')
plt.show()
Area: 200
number of measurements per bin
10
0.8
0.6
0.4
0.2
0.0
250
300
A = L. W
350
400
450
500](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fb7f470a0-93f2-43bf-9ed3-2f125d19f075%2F6530e124-8161-423a-8baa-679de4ec85a9%2Fzkqunir_processed.png&w=3840&q=75)
Transcribed Image Text:In [1]:
The surface area of the rectangle bounded by L and Wis
Translate this equation into code and verify a few values of A with your calculator. Again, this code is simple a one-liner.
import matplotlib.pyplot as plt
import numpy as np
# Calculate the area using the formula: A = L x W
def calculate_area (L, W):
return L * W
# Example values
L = 10 # Example length
W = 20 # Example width
A = calculate_area (L, W)
print("Area:", A)
#Assuming we have multiple values of A for histogram plotting
A_values = [calculate_area(i, i+5) for i in range (10, 30)]
#Plotting the histogram for A
plt.figure (figsize=(9, 6))
plt.hist(A_values, np.arange (250, 505, 5), facecolor='darkblue', alpha=0.75)
plt.xlabel('area (mm$^2$)')
plt.ylabel('number of measurements per bin')
plt.show()
Area: 200
number of measurements per bin
10
0.8
0.6
0.4
0.2
0.0
250
300
A = L. W
350
400
450
500
![In [3]:
Next, we plot the histogram for A:
plt.figure(figsize=(9,6))
#Define the figure size (the default is a little too small)
plt.hist (A, np.arange (250,505,5), facecolor='darkblue' , alpha=0.75)
plt.xlabel('area (mm$^2$) ')
plt.ylabel('number of measurements per bin');
number of measurements per bin
0.04
0.02
0.00
-0.02
-0.04
250
300
350
area (mm²)
400
450
500](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fb7f470a0-93f2-43bf-9ed3-2f125d19f075%2F6530e124-8161-423a-8baa-679de4ec85a9%2F3h7pk8_processed.png&w=3840&q=75)
Transcribed Image Text:In [3]:
Next, we plot the histogram for A:
plt.figure(figsize=(9,6))
#Define the figure size (the default is a little too small)
plt.hist (A, np.arange (250,505,5), facecolor='darkblue' , alpha=0.75)
plt.xlabel('area (mm$^2$) ')
plt.ylabel('number of measurements per bin');
number of measurements per bin
0.04
0.02
0.00
-0.02
-0.04
250
300
350
area (mm²)
400
450
500
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 3 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
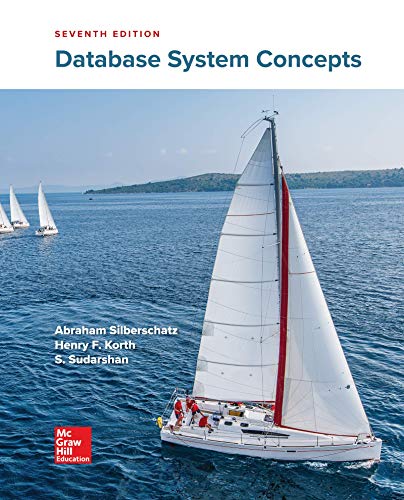
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
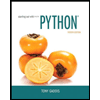
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
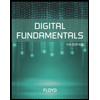
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
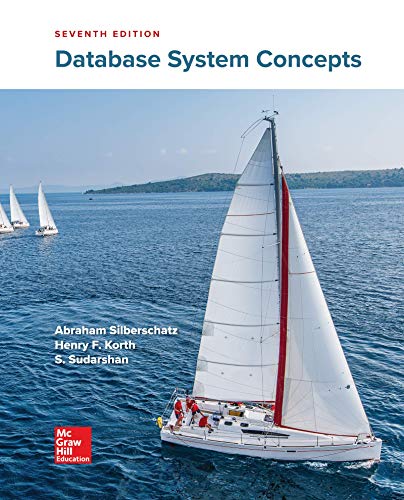
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
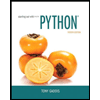
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
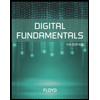
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
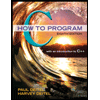
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
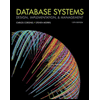
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
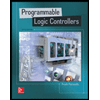
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education