Python: How do I do specifically do the following to my code:1. Have a GUI asking for the amount of lakes, mountains, AND rivers to be RANDOMLY generated.2. Lower the 'island' land generating circles (beach/sand, grass) to 0,0 on the grid (prevent generating lakes/mountains off of land).3. INCREASE the size of the circles 3 times than it currently already is, to take up more of the grid. AKA, circles widened three times than they already are.4. Have "rivers" cut through ENTIRE island (slice in half, not cut half way and begin another cut).5. Make the mountains triangles instead of circles.Really need help on this. My code so far:import turtleimport random # Define colorsocean = "#000066"sand = "#ffff66"grass = "#00cc00"lake = "#0066ff"mountain_color = "#808080" # Gray color for mountains # Store mountain positionsmountain_positions = [] def draw_circle(radius, line_color, fill_color): my_turtle.color(line_color) my_turtle.fillcolor(fill_color) my_turtle.begin_fill() my_turtle.circle(radius) my_turtle.end_fill() def move_turtle(x, y): my_turtle.penup() my_turtle.goto(x, y) my_turtle.pendown() def draw_lake(): x = random.randint(-100, 100) y = random.randint(-50, 100) move_turtle(x, y) draw_circle(30, lake, lake) def draw_mountains(): x = random.randint(-100, 100) y = random.randint(-50, 100) mountain_positions.append((x, y)) screen = turtle.Screen()screen.bgcolor(ocean)screen.title("Island Generator") my_turtle = turtle.Turtle()my_turtle.pensize(4)my_turtle.shape("circle") # Draw the sand areadraw_circle(150, sand, sand) # Draw the grass areamove_turtle(0, 30)draw_circle(120, grass, grass) # Draw lakes as bigger random dotsnum_lakes = 2 # Number of lakes to drawfor _ in range(num_lakes): draw_lake() # Draw mountainsnum_mountains = 5 # Number of mountains to drawfor _ in range(num_mountains): draw_mountains() # Draw mountains as separate colorfor pos in mountain_positions: move_turtle(pos[0], pos[1]) draw_circle(15, mountain_color, mountain_color) # Draw riversnum_cuts = int(input("How many rivers do you want on your Island? "))move_turtle(0, 150)my_turtle.color(ocean)for _ in range(num_cuts): my_turtle.pendown() my_turtle.left(360 / num_cuts) my_turtle.forward(150) my_turtle.penup() my_turtle.backward(150) turtle.done()
Python: How do I do specifically do the following to my code:
1. Have a GUI asking for the amount of lakes, mountains, AND rivers to be RANDOMLY generated.
2. Lower the 'island' land generating circles (beach/sand, grass) to 0,0 on the grid (prevent generating lakes/mountains off of land).
3. INCREASE the size of the circles 3 times than it currently already is, to take up more of the grid. AKA, circles widened three times than they already are.
4. Have "rivers" cut through ENTIRE island (slice in half, not cut half way and begin another cut).
5. Make the mountains triangles instead of circles.
Really need help on this. My code so far:
import turtle
import random
# Define colors
ocean = "#000066"
sand = "#ffff66"
grass = "#00cc00"
lake = "#0066ff"
mountain_color = "#808080" # Gray color for mountains
# Store mountain positions
mountain_positions = []
def draw_circle(radius, line_color, fill_color):
my_turtle.color(line_color)
my_turtle.fillcolor(fill_color)
my_turtle.begin_fill()
my_turtle.circle(radius)
my_turtle.end_fill()
def move_turtle(x, y):
my_turtle.penup()
my_turtle.goto(x, y)
my_turtle.pendown()
def draw_lake():
x = random.randint(-100, 100)
y = random.randint(-50, 100)
move_turtle(x, y)
draw_circle(30, lake, lake)
def draw_mountains():
x = random.randint(-100, 100)
y = random.randint(-50, 100)
mountain_positions.append((x, y))
screen = turtle.Screen()
screen.bgcolor(ocean)
screen.title("Island Generator")
my_turtle = turtle.Turtle()
my_turtle.pensize(4)
my_turtle.shape("circle")
# Draw the sand area
draw_circle(150, sand, sand)
# Draw the grass area
move_turtle(0, 30)
draw_circle(120, grass, grass)
# Draw lakes as bigger random dots
num_lakes = 2 # Number of lakes to draw
for _ in range(num_lakes):
draw_lake()
# Draw mountains
num_mountains = 5 # Number of mountains to draw
for _ in range(num_mountains):
draw_mountains()
# Draw mountains as separate color
for pos in mountain_positions:
move_turtle(pos[0], pos[1])
draw_circle(15, mountain_color, mountain_color)
# Draw rivers
num_cuts = int(input("How many rivers do you want on your Island? "))
move_turtle(0, 150)
my_turtle.color(ocean)
for _ in range(num_cuts):
my_turtle.pendown()
my_turtle.left(360 / num_cuts)
my_turtle.forward(150)
my_turtle.penup()
my_turtle.backward(150)
turtle.done()

Step by step
Solved in 2 steps

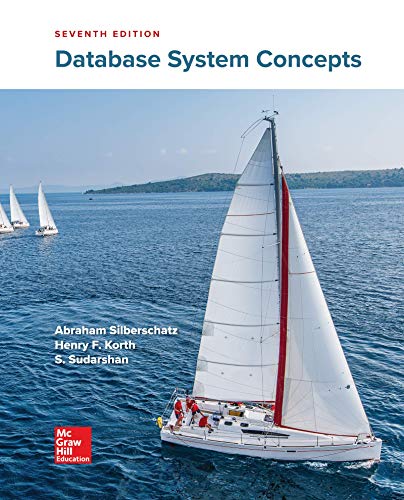
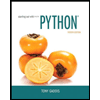
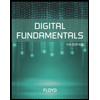
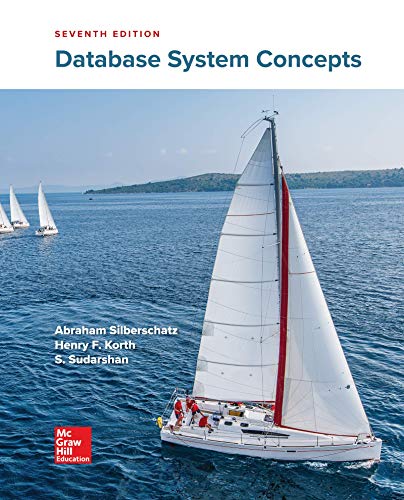
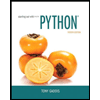
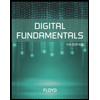
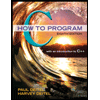
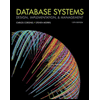
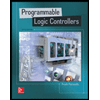