Main program file: public class BankAccountMainProgram { public static void main(String[] arguments) { // create three bank accounts BankAccount accountNum1 = new BankAccount(); BankAccount accountNum2 = new BankAccount(1233203, 105.51, "Betty"); BankAccount accountNum3 = new BankAccount(6542345, 33.11, "Veronica"); // Test the two String Method System.out.println("*** Does the toStringMethod work?"); System.out.println("First account : " + accountNum1); System.out.println("Second account : " + accountNum2); System.out.println("Third account : " + accountNum3); System.out.println(); // Do the get() method work? System.out.println("*** Does the get() methods work?"); System.out.println(accountNum2.getName() + " has $" + accountNum2.getBalance() + " in account number " + accountNum2.getID() + "."); System.out.println(); // Can we change the account name System.out.println("*** Does the set() methods work?"); System.out.println("Second account Before : " + accountNum2); accountNum2.setName("Archie"); System.out.println("Second account After : " + accountNum2); // Can we change the account name System.out.println(); System.out.println("*** Does the changeBalance() methods work?"); System.out.println("Third account Before : " + accountNum3); accountNum3.changeBalance(10.10); System.out.println("Third account After : " + accountNum3); accountNum3.changeBalance(-50); System.out.println("Third account After : " + accountNum3); } } Create a Bank Account class with the Main program file above. Your Bank Account class should have the following Fields a Private ID (int) a private Name (String) a private balance (double) Methods A default constructor that sets the ID to 0 the balance to 0 the name to "unknown" A full constructor That accepts all three values and sets the values accordingly. A to String() method That returns a string in the format Name [ID] = $balance Example: Betty [1233203] = $105.51 getName() getBalance() getID() These should just return the values with no changes. setName() This should change the name on the account, and only the name. changeBalance(double x) This should add the amount x to the balance. if x is negative you still add it, but the balance should go down. If the balance is negative, then set it to zero before finishing.
Main program file:
public class BankAccountMainProgram
{
public static void main(String[] arguments)
{
// create three bank accounts
BankAccount accountNum1 = new BankAccount();
BankAccount accountNum2 = new BankAccount(1233203, 105.51, "Betty");
BankAccount accountNum3 = new BankAccount(6542345, 33.11, "Veronica");
// Test the two String Method
System.out.println("*** Does the toStringMethod work?");
System.out.println("First account : " + accountNum1);
System.out.println("Second account : " + accountNum2);
System.out.println("Third account : " + accountNum3);
System.out.println();
// Do the get() method work?
System.out.println("*** Does the get() methods work?");
System.out.println(accountNum2.getName() + " has $" + accountNum2.getBalance() +
" in account number " + accountNum2.getID() + ".");
System.out.println();
// Can we change the account name
System.out.println("*** Does the set() methods work?");
System.out.println("Second account Before : " + accountNum2);
accountNum2.setName("Archie");
System.out.println("Second account After : " + accountNum2);
// Can we change the account name
System.out.println();
System.out.println("*** Does the changeBalance() methods work?");
System.out.println("Third account Before : " + accountNum3);
accountNum3.changeBalance(10.10);
System.out.println("Third account After : " + accountNum3);
accountNum3.changeBalance(-50);
System.out.println("Third account After : " + accountNum3);
}
}
Create a Bank Account class with the Main program file above.
Your Bank Account class should have the following
- Fields
- a Private ID (int)
- a private Name (String)
- a private balance (double)
- Methods
- A default constructor
- that sets the ID to 0
- the balance to 0
- the name to "unknown"
- A full constructor
- That accepts all three values and sets the values accordingly.
- A to String() method
- That returns a string in the format
- Name [ID] = $balance
- Example: Betty [1233203] = $105.51
- getName()
- getBalance()
- getID()
- These should just return the values with no changes.
- setName()
- This should change the name on the account, and only the name.
- changeBalance(double x)
- This should add the amount x to the balance.
- if x is negative you still add it, but the balance should go down.
- If the balance is negative, then set it to zero before finishing.

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

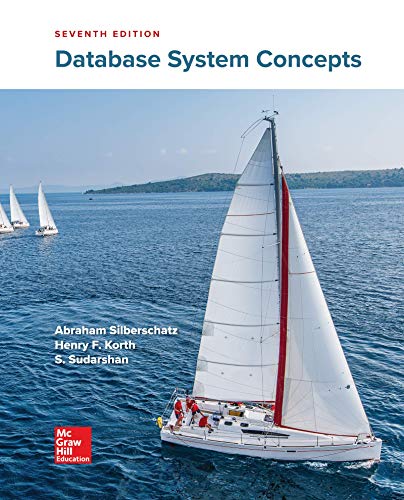
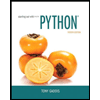
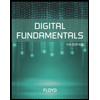
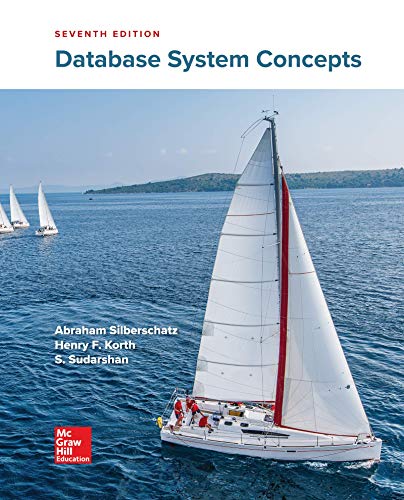
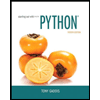
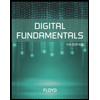
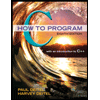
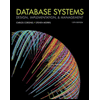
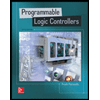