Full Code: #include #include #include #define seed 5457 #define max(a,b) \ ({ __typeof__ (a) _a = (a); \ __typeof__ (b) _b = (b); \ _a > _b ? _a : _b; }) #define min(a,b) \ ({ __typeof__ (a) _a = (a); \ __typeof__ (b) _b = (b); \ _a < _b ? _a : _b; }) int initializeArray(int [], const int); void randperm(int b[], int n); int checkboard(int b[], int n); void displayboard(int b[], int n); double factorial(double n); int main() { srandom(seed); int mini[21], maxi[21]; double meani[21], sizei[21], sizei_f[21]; int n; for (n=4; n<=20; n++) { int b[n]; initializeArray(b,n); printf("~~~~~~~~~PRINTING FOR %d~~~~~~~~~~~~~\n",n); int total = 0; int x; for (x = 0; x<10; x++) { int count = 1; randperm(b,n); while(!checkboard(b,n)) { randperm(b,n); count++; } if (x==0) { mini[n] = count; maxi[n] = count; displayboard(b,n); } mini[n] = min(mini[n],count); maxi[n] = max(maxi[n], count); total += count; } meani[n] = total/10.0f; double size = pow(n,n); sizei[n] = size; double size_f = factorial(n); sizei_f[n] = size_f; } printf("\n%13s%13s%13s%13s%13s%13s\n", "size", "min", "max", "mean", "size**size", "size!"); int x; for (x=4; x<=20; x++) { printf("%13d%13d%13d%13.1e%13.1e%13.1e\n", x, mini[x], maxi[x], meani[x], sizei[x], sizei_f[x]); } } int initializeArray(int *a, const int arraySize) { int i; for (i=0; i { a[i] = i; } } void randperm(int b[], int n) { int i; for (i=n-1; i>0; i--) { int j; int temp; j = random() % (i+1); temp = b[j]; b[j] = b[i]; b[i] = temp; } } int checkboard(int b[], int n) { int x; for (x = 0; x { int z; for (z=x+1; z { if (abs(x-z) == abs(b[x]-b[z])) { return 0; } } } return 1; } void displayboard(int b[], int n) { int x; for (x=0; x { int i; for (i = 0; i < n; i++) { if (i == b[x]) { printf("*"); } else printf("-"); } printf("\n"); } } double factorial(double n) { if (n==1) { return 1; } else { return n*factorial(n-1); } } Can you replace the part below with something else for the same outcome? Replace this part in the Full Code: #define max(a,b) \ ({ __typeof__ (a) _a = (a); \ __typeof__ (b) _b = (b); \ _a > _b ? _a : _b; }) #define min(a,b) \ ({ __typeof__ (a) _a = (a); \ __typeof__ (b) _b = (b); \ _a < _b ? _a : _b; })
Full Code:
#include
#include
#include
#define seed 5457
#define max(a,b) \
({ __typeof__ (a) _a = (a); \
__typeof__ (b) _b = (b); \
_a > _b ? _a : _b; })
#define min(a,b) \
({ __typeof__ (a) _a = (a); \
__typeof__ (b) _b = (b); \
_a < _b ? _a : _b; })
int initializeArray(int [], const int);
void randperm(int b[], int n);
int checkboard(int b[], int n);
void displayboard(int b[], int n);
double factorial(double n);
int main()
{
srandom(seed);
int mini[21], maxi[21];
double meani[21], sizei[21], sizei_f[21];
int n;
for (n=4; n<=20; n++)
{
int b[n];
initializeArray(b,n);
printf("~~~~~~~~~PRINTING FOR %d~~~~~~~~~~~~~\n",n);
int total = 0;
int x;
for (x = 0; x<10; x++)
{
int count = 1;
randperm(b,n);
while(!checkboard(b,n))
{
randperm(b,n);
count++;
}
if (x==0)
{
mini[n] = count;
maxi[n] = count;
displayboard(b,n);
}
mini[n] = min(mini[n],count);
maxi[n] = max(maxi[n], count);
total += count;
}
meani[n] = total/10.0f;
double size = pow(n,n);
sizei[n] = size;
double size_f = factorial(n);
sizei_f[n] = size_f;
}
printf("\n%13s%13s%13s%13s%13s%13s\n", "size", "min", "max", "mean", "size**size", "size!");
int x;
for (x=4; x<=20; x++)
{
printf("%13d%13d%13d%13.1e%13.1e%13.1e\n", x, mini[x], maxi[x], meani[x], sizei[x], sizei_f[x]);
}
}
int initializeArray(int *a, const int arraySize)
{
int i;
for (i=0; i {
a[i] = i;
}
}
void randperm(int b[], int n)
{
int i;
for (i=n-1; i>0; i--)
{
int j;
int temp;
j = random() % (i+1);
temp = b[j];
b[j] = b[i];
b[i] = temp;
}
}
int checkboard(int b[], int n)
{
int x;
for (x = 0; x {
int z;
for (z=x+1; z {
if (abs(x-z) == abs(b[x]-b[z]))
{
return 0;
}
}
}
return 1;
}
void displayboard(int b[], int n)
{
int x;
for (x=0; x {
int i;
for (i = 0; i < n; i++)
{
if (i == b[x])
{
printf("*");
}
else
printf("-");
}
printf("\n");
}
}
double factorial(double n)
{
if (n==1)
{
return 1;
}
else
{
return n*factorial(n-1);
}
}
Can you replace the part below with something else for the same outcome?
Replace this part in the Full Code:
#define max(a,b) \
({ __typeof__ (a) _a = (a); \
__typeof__ (b) _b = (b); \
_a > _b ? _a : _b; })
#define min(a,b) \
({ __typeof__ (a) _a = (a); \
__typeof__ (b) _b = (b); \
_a < _b ? _a : _b; })

Step by step
Solved in 6 steps with 1 images

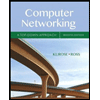
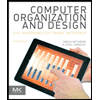
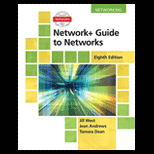
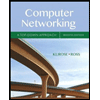
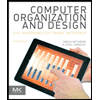
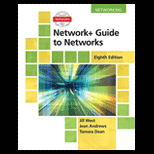
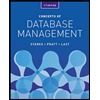
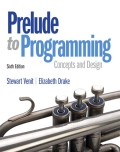
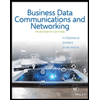