food_wastage_record.hpp class FoodWastageRecord { public: void SetDate(const std::string &date); void SetMeal(const std::string &meal); void SetFoodName(const std::string &food_name); void SetQuantityInOz(double qty_in_oz); void SetWastageReason(const std::string &wastage_reason); void SetDisposalMechanism(const std::string &disposal_mechanism); void SetCost(double cost); std::string Date() const; std::string Meal() const; std::string FoodName() const; double QuantityInOz() const; std::string WastageReason() const; std::string DisposalMechanism() const; double Cost() const; private: std::string date_; std::string meal_; std::string food_name_; double qty_in_oz_; std::string wastage_reason_; std::string disposal_mechanism_; double cost_; }; food_wastage_record.cpp #include "food_wastage_record.h" void FoodWastageRecord::SetDate(const std::string &date) { date_ = date; } void FoodWastageRecord::SetMeal(const std::string &meal) { meal_ = meal; } void FoodWastageRecord::SetFoodName(const std::string &food_name) { food_name_ = food_name; } void FoodWastageRecord::SetQuantityInOz(double qty_in_oz) { qty_in_oz_ = qty_in_oz; } void FoodWastageRecord::SetWastageReason(const std::string &wastage_reason) { wastage_reason_ = wastage_reason; } void FoodWastageRecord::SetDisposalMechanism( const std::string &disposal_mechanism) { disposal_mechanism_ = disposal_mechanism; } void FoodWastageRecord::SetCost(double cost) { cost_ = cost; } std::string FoodWastageRecord::Date() const { return date_; } std::string FoodWastageRecord::Meal() const { return meal_; } std::string FoodWastageRecord::FoodName() const { return food_name_; } double FoodWastageRecord::QuantityInOz() const { return qty_in_oz_; } std::string FoodWastageRecord::WastageReason() const { return wastage_reason_; } std::string FoodWastageRecord::DisposalMechanism() const { return disposal_mechanism_; } double FoodWastageRecord::Cost() const { return cost_; } food_wastage_report.hpp class FoodWastageReport { public: FoodWastageReport(const std::vector &food_wastage_records); std::vector MostCommonlyWastedFoods() const; std::vector MostCostlyWasteProducingMeals() const; double TotalCostOfFodWasted() const; std::vector MostCommonWastageReasons() const; std::vector MostCommonDisposalMechanisms() const; std::vector SuggestWasteReductionStrategies() const; private: const std::vector &food_wastage_records_; std::vector most_commonly_wasted_foods_; std::vector most_costly_waste_producing_meals_; double total_cost_of_food_wasted_; std::vector most_common_wastage_reasons_; std::vector most_common_disposal_mechanisms_; std::vector suggested_strategies_to_reduce_waste_; }; food_wastage_report.cpp //how would I define the member functions declared in the food_wastage_report.h fil? food_wastage_tracker.hpp class FoodWastageTracker { public: bool AddRecord(const FoodWastageRecord &record); bool DeleteRecord(const FoodWastageRecord &record); const std::vector &GetRecords() const; FoodWastageReport GetFoodWastageReport() const; private: std::vector food_wastage_records_; }; food_wastage_tracker.cpp //how would I define the member functions declared in the food_wastage_tracker.h file?
food_wastage_record.hpp
class FoodWastageRecord {
public:
void SetDate(const std::string &date);
void SetMeal(const std::string &meal);
void SetFoodName(const std::string &food_name);
void SetQuantityInOz(double qty_in_oz);
void SetWastageReason(const std::string &wastage_reason);
void SetDisposalMechanism(const std::string &disposal_mechanism);
void SetCost(double cost);
std::string Date() const;
std::string Meal() const;
std::string FoodName() const;
double QuantityInOz() const;
std::string WastageReason() const;
std::string DisposalMechanism() const;
double Cost() const;
private:
std::string date_;
std::string meal_;
std::string food_name_;
double qty_in_oz_;
std::string wastage_reason_;
std::string disposal_mechanism_;
double cost_;
};
food_wastage_record.cpp
#include "food_wastage_record.h"
void FoodWastageRecord::SetDate(const std::string &date) { date_ = date; }
void FoodWastageRecord::SetMeal(const std::string &meal) { meal_ = meal; }
void FoodWastageRecord::SetFoodName(const std::string &food_name) {
food_name_ = food_name;
}
void FoodWastageRecord::SetQuantityInOz(double qty_in_oz) {
qty_in_oz_ = qty_in_oz;
}
void FoodWastageRecord::SetWastageReason(const std::string &wastage_reason) {
wastage_reason_ = wastage_reason;
}
void FoodWastageRecord::SetDisposalMechanism(
const std::string &disposal_mechanism) {
disposal_mechanism_ = disposal_mechanism;
}
void FoodWastageRecord::SetCost(double cost) { cost_ = cost; }
std::string FoodWastageRecord::Date() const { return date_; }
std::string FoodWastageRecord::Meal() const { return meal_; }
std::string FoodWastageRecord::FoodName() const { return food_name_; }
double FoodWastageRecord::QuantityInOz() const { return qty_in_oz_; }
std::string FoodWastageRecord::WastageReason() const { return wastage_reason_; }
std::string FoodWastageRecord::DisposalMechanism() const {
return disposal_mechanism_;
}
double FoodWastageRecord::Cost() const { return cost_; }
food_wastage_report.hpp
class FoodWastageReport {
public:
FoodWastageReport(const std::
std::vector<std::string> MostCommonlyWastedFoods() const;
std::vector<std::string> MostCostlyWasteProducingMeals() const;
double TotalCostOfFodWasted() const;
std::vector<std::string> MostCommonWastageReasons() const;
std::vector<std::string> MostCommonDisposalMechanisms() const;
std::vector<std::string> SuggestWasteReductionStrategies() const;
private:
const std::vector<FoodWastageRecord> &food_wastage_records_;
std::vector<std::string> most_commonly_wasted_foods_;
std::vector<std::string> most_costly_waste_producing_meals_;
double total_cost_of_food_wasted_;
std::vector<std::string> most_common_wastage_reasons_;
std::vector<std::string> most_common_disposal_mechanisms_;
std::vector<std::string> suggested_strategies_to_reduce_waste_;
};
food_wastage_report.cpp
//how would I define the member functions declared in the food_wastage_report.h fil?
food_wastage_tracker.hpp
class FoodWastageTracker {
public:
bool AddRecord(const FoodWastageRecord &record);
bool DeleteRecord(const FoodWastageRecord &record);
const std::vector<FoodWastageRecord> &GetRecords() const;
FoodWastageReport GetFoodWastageReport() const;
private:
std::vector<FoodWastageRecord> food_wastage_records_;
};
food_wastage_tracker.cpp
//how would I define the member functions declared in the food_wastage_tracker.h file?

Trending now
This is a popular solution!
Step by step
Solved in 3 steps

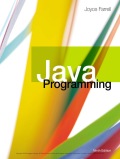
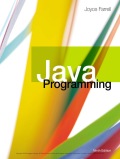